Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Hello World App
Let?s develop a first hello word application on Android studio using Kotlin programming language. To develop android application launch Android Studio and select option 'Start a new Android Studio project'.
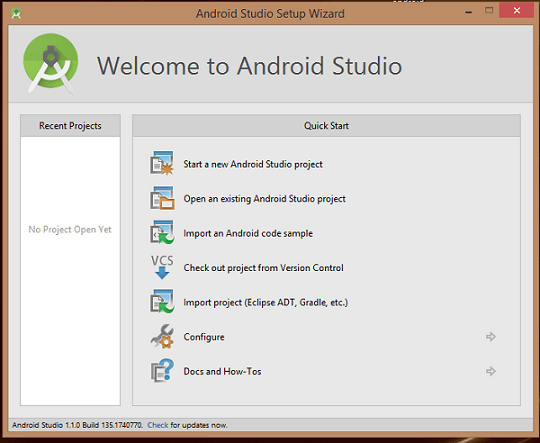
Provide a application name ('Hello World' in my case) and check 'Include Kotlin support' and proceed.
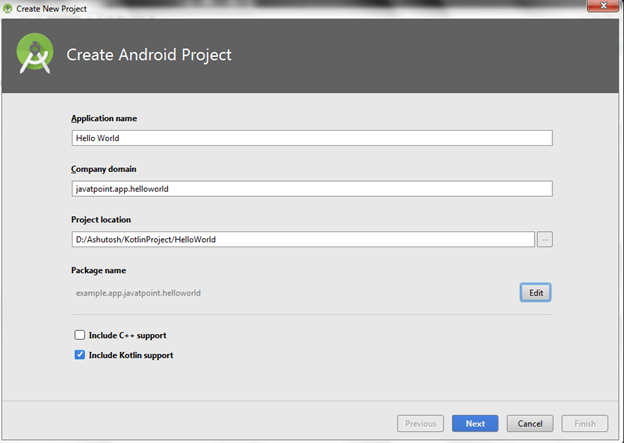
Select API level for android application and click next.
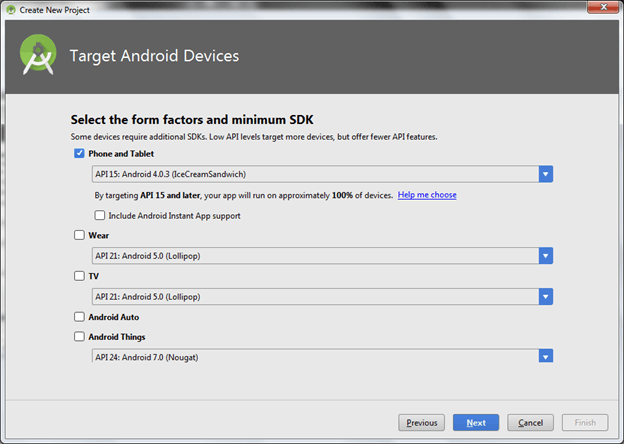
Select Activity type and click next.
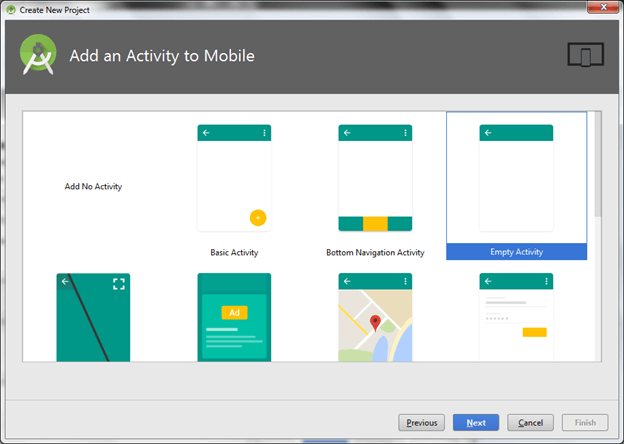
activity_main.xml
Create an activity_main.xml file in layout folder and add the following code.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.app.javatpoint.helloworld.MainActivity"> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" /> < /android.support.constraint.ConstraintLayout>
MainActivity.kt
Create MainActivity.kt file in example.app.javatpoint.helloworld package add the following code.
package example.app.javatpoint.helloworld import android.support.v7.app.AppCompatActivity import android.os.Bundle class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) } }
Now run your app.
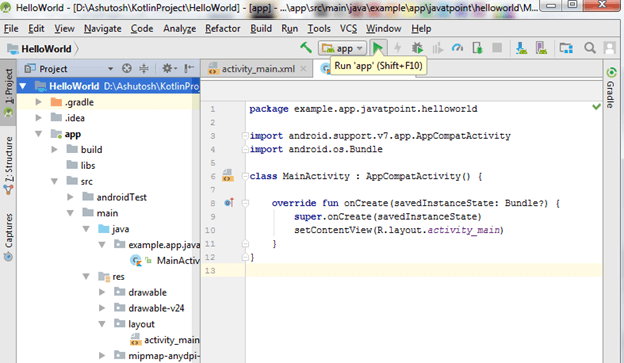
Output:
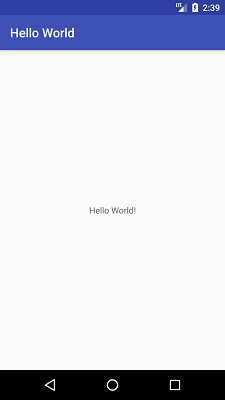