Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Android Toast
Android Toast is used to display a sort time notification to the user without affecting the user interaction with UI. The message displayed using Toast class displays quickly, and it disappears after some time. The message in the Toast can be of type text, image or both.
To learn more about Android Toast, go to Android Toast Example
Kotlin Android Toast Example
In this example, we will display a toast message by clicking on a Button. See the following example:
Toast.makeText(applicationContext,"this is toast message",Toast.LENGTH_SHORT).show() val toast = Toast.makeText(applicationContext, "Hello Javatpoint", Toast.LENGTH_LONG) toast.show() val myToast = Toast.makeText(applicationContext,"toast message with gravity",Toast.LENGTH_SHORT) myToast.setGravity(Gravity.LEFT,200,200) myToast.show()
- The applicationContest returns the instance of Context class.
- The message is of String type as ("this is toast message").
- The Toast.LENGTH_SHORT and Toast.LENGTH_LONG are the constant defines the time duration for message display.
- The show() method of Toast class used to display the toast message.
- The setGravity() method of Toast used to customize the position of toast message.
activity_main.xml
Add the following code in the activity_main.xml file. In this file, we have added a button to the layout to perform a click action.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlintoast.MainActivity"> < Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:text="Click to display toast" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> < /android.support.constraint.ConstraintLayout>
MainActivity.kt
Add the following code in the MainActivity.kt class. In this class, we are performing a click action on the button which displays the toast message.
package example.javatpoint.com.kotlintoast import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.view.Gravity import android.widget.Toast import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) button.setOnClickListener(){ Toast.makeText(applicationContext,"this is toast message",Toast.LENGTH_SHORT).show() val toast = Toast.makeText(applicationContext, "Hello Javatpoint", Toast.LENGTH_SHORT) toast.show() val myToast = Toast.makeText(applicationContext,"toast message with gravity",Toast.LENGTH_SHORT) myToast.setGravity(Gravity.LEFT,200,200) myToast.show() } } }
Output:
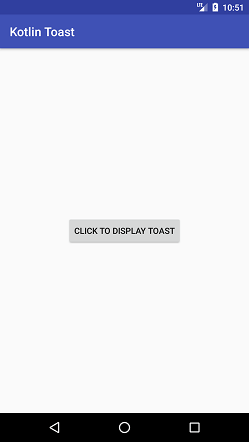
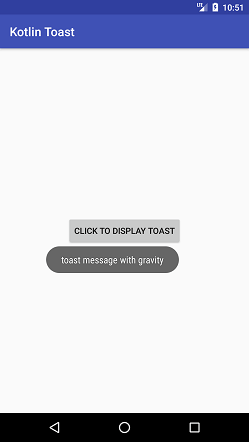