Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Android XML Parsing using DOM Parser
XML document is commonly used to share the data on the internet. The data provided in XML format are able to update frequently and parsing them is a common task for network-based apps.
In Android, there are three types of XML parsers to parse the XML data and read them in android applications, these are:
- DOM Parser
- SAX Parser
- XMLPullParser
Android DOM (Document Object Model) parser uses an object-based approach to create and parse the XML files in android applications. The DOM parser loads the XML file into memory to parse the XML document. Due to this reason, it consumes more memory.
Example of XML Parsing using DOM Parser
In this example, we parse XML data and display them into ListView.
activity_main.xml
Add the ListView in the activity_main.xml layout.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinxmlparsingusingdomparser.MainActivity"> < ListView android:id="@+id/listView" android:layout_width="match_parent" android:layout_height="match_parent"> < /ListView> < /android.support.constraint.ConstraintLayout>
empdetail.xml
Create the XML document empdetail.xml in assets directory to parse the data using DOM parser.
< ?xml version="1.0" encoding="utf-8"?> < records> < employee> < name>Sachin Kumar< /name> < salary>50000< /salary> < designation>Developer< /designation> < /employee> < employee> < name>Rahul Kumar< /name> < salary>60000< /salary> < designation>Team Leader< /designation> < /employee> < employee> < name>John Mike< /name> < salary>70000< /salary> < designation>Manager< /designation> < /employee> < employee> < name>Ajay Kumar name> < salary>45000< /salary> < designation>Developer< /designation> < /employee> < employee> < name>Toni Nayer< /name> < salary>55000< /salary> < designation>Marketing< /designation> < /employee> < employee> < name>Mr Bony< /name> < salary>42000< /salary> < designation>Sales< /designation> < /employee> < employee> < name>Raj Kumar< /name> < salary>30000< /salary> < designation>Production< /designation> < /employee> < employee> < name>Rahul Kumar< /name> < salary>60000< /salary> < designation>Team Leader< /designation> < /employee> < employee> < name>John Mike< /name> < salary>70000< /salary> < designation>Manager< /designation> < /employee> < employee> < name>Sachin Kumar< /name> < salary>50000< /salary> < designation>Developer< /designation> < /employee> < employee> < name>Rahul Kumar< /name> < salary>60000< /salary> < designation>Team Leader< /designation> < /employee> < employee> < name>John Mike< /name> < salary>70000< /salary> < designation>Manager< /designation> < /employee> < /records>
custom_list.xml
Create a custom layout to display the list of data into ListView.
< ?xml version="1.0" encoding="utf-8"?> < LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="horizontal"> < LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="1" android:orientation="vertical"> < TextView android:id="@+id/name" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="name" android:textStyle="bold" android:layout_marginLeft="15dp" android:layout_marginStart="15dp" android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" /> < TextView android:id="@+id/salary" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="salary" android:layout_marginLeft="15dp" android:layout_marginStart="15dp" android:layout_marginTop="5dp" android:textSize="16sp"/> < TextView android:id="@+id/designation" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="designation" android:layout_marginLeft="15dp" android:layout_marginStart="15dp" android:layout_marginTop="5dp" android:textSize="16sp"/> < /LinearLayout> < /LinearLayout>
MainActivity.kt
Add the following code to read and parse the XML data using DOM parser. Create the instance of DocumentBuilderFactory, DocumentBuilder and Document objects.
The HashMap< String, String> is used to read the data from XML document and add them into ArrayList().
package example.javatpoint.com.kotlinxmlparsingusingdomparser import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.widget.ListView import android.widget.SimpleAdapter import org.w3c.dom.Element import org.w3c.dom.Node import org.xml.sax.SAXException import java.io.IOException import javax.xml.parsers.DocumentBuilderFactory import javax.xml.parsers.ParserConfigurationException class MainActivity : AppCompatActivity() { var empDataHashMap = HashMap< String, String>() var empList: ArrayList< HashMap< String, String>> = ArrayList() override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) try { val lv = findViewById< ListView>(R.id.listView) val istream = assets.open("empdetail.xml") val builderFactory = DocumentBuilderFactory.newInstance() val docBuilder = builderFactory.newDocumentBuilder() val doc = docBuilder.parse(istream) //reading the tag "employee" of empdetail file val nList = doc.getElementsByTagName("employee") for (i in 0 until nList.getLength()) { if (nList.item(0).getNodeType().equals(Node.ELEMENT_NODE) ) { //creating instance of HashMap to put the data of node value empDataHashMap = HashMap() val element = nList.item(i) as Element empDataHashMap.put("name", getNodeValue("name", element)) empDataHashMap.put("salary", getNodeValue("salary", element)) empDataHashMap.put("designation", getNodeValue("designation", element)) //adding the HashMap data to ArrayList empList.add(empDataHashMap) } } val adapter = SimpleAdapter(this@MainActivity, empList, R.layout.custom_list, arrayOf("name", "salary", "designation"), intArrayOf(R.id.name, R.id.salary, R.id.designation)) lv.setAdapter(adapter) } catch (e: IOException) { e.printStackTrace() } catch (e: ParserConfigurationException) { e.printStackTrace() } catch (e: SAXException) { e.printStackTrace() } } // function to return node value protected fun getNodeValue(tag: String, element: Element): String { val nodeList = element.getElementsByTagName(tag) val node = nodeList.item(0) if (node != null) { if (node.hasChildNodes()) { val child = node.getFirstChild() while (child != null) { if (child.getNodeType() === Node.TEXT_NODE) { return child.getNodeValue() } } } } return "" } }
Output:
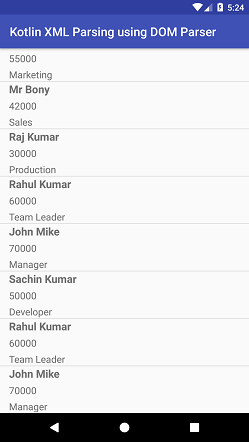