Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Google Map Search Location
In the previous tutorial, we build the application that locate the Map Fixed Location and Map Current Location.
In this tutorial, we will implement search location functionality in Google Map. The searching of Google location is done through Geocoder class. Geocoder class facilitates the geocoding and reverse geocoding.
Geocoding is a process in which street address is converted into a coordinate (latitude, longitude). Reverse geocoding is a process in which a coordinate (latitude, longitude) is converted into the street address.
Methods of Geocoder class
- List
getFromLocation(double latitude, double longitude, int maxResults): This method returns an array of Address which specifies the surrounding latitude and longitude. - List
getFromLocationName(String location, int results, double leftLatitude, double leftLongitude, double rightLatitude, double rightLongitude): This method returns an array of Address which describes the given location such as place, an address, etc. - List
getFromLocationName(String location, int results): This method returns an array of Address which describes the given location such as place, an address, etc. - static boolean isPresent(): This method returns true if the methods getFromLocation() and getFromLocationName() are implemented.
activity_maps.xml
Add the following code in an activity_maps.xml layout file. The EditText is used to input search location and Button is used for click event to search the place.
< fragment xmlns:android="http://schemas.android.com/apk/res/android" xmlns:map="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/map" android:name="com.google.android.gms.maps.SupportMapFragment" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlingooglesearchlocation.MapsActivity" > < LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> < EditText android:layout_width="248dp" android:layout_height="wrap_content" android:id="@+id/editText" android:layout_weight="0.5" android:inputType="textPersonName" android:hint="Search Location" /> < Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="0.5" android:onClick="searchLocation" android:text="Search" /> < /LinearLayout> < /fragment> < fragment xmlns:android="http://schemas.android.com/apk/res/android" xmlns:map="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/map" android:name="com.google.android.gms.maps.SupportMapFragment" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlingooglesearchlocation.MapsActivity" > < LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> < EditText android:layout_width="248dp" android:layout_height="wrap_content" android:id="@+id/editText" android:layout_weight="0.5" android:inputType="textPersonName" android:hint="Search Location" /> < Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="0.5" android:onClick="searchLocation" android:text="Search" /> < /LinearLayout> < /fragment>
build.gradle
Add the Google Map Service and Google Location Service dependencies in build.gradle file.
dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk8:$kotlin_version" implementation 'com.android.support:appcompat-v7:26.1.0' implementation 'com.google.android.gms:play-services-maps:11.8.0' compile 'com.google.android.gms:play-services-location:11.8.0' testImplementation 'junit:junit:4.12' testImplementation 'junit:junit:4.12' } dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk8:$kotlin_version" implementation 'com.android.support:appcompat-v7:26.1.0' implementation 'com.google.android.gms:play-services-maps:11.8.0' compile 'com.google.android.gms:play-services-location:11.8.0' testImplementation 'junit:junit:4.12' testImplementation 'junit:junit:4.12' }
strings.xml
< resources> < string name="app_name">Kotlin Google Search Location< /string> < string name="title_activity_maps">Google Search Location< /string> < /resources> < resources> < string name="app_name">Kotlin Google Search Location< /string> < string name="title_activity_maps">Google Search Location< /string> < /resources>
google_map_api.xml
Place the Google Map API key in res/values/google_map_api.xml file.
< resources> < !-- https://console.developers.google.com/flows/enableapi?apiid=maps_android_backend&keyType=CLIENT_SIDE_ANDROID&r=20:0B:71:3B:B2:46:75:A1:87:78:2E:4C:49:3F:E3:B6:FD:2D:76:D3%3Bexample.javatpoint.com.kotlingooglesearchlocation Alternatively, follow the directions here: https://developers.google.com/maps/documentation/android/start#get-key Once you have your key (it starts with "AIza"), replace the "google_maps_key" string in this file. --> < string name="google_maps_key" templateMergeStrategy="preserve" translatable="false">AIzaSyCKvLn2KTPKD_-REPLACE-WITH-YOUR-API< /string> < /resources> < resources> < !-- https://console.developers.google.com/flows/enableapi?apiid=maps_android_backend&keyType=CLIENT_SIDE_ANDROID&r=20:0B:71:3B:B2:46:75:A1:87:78:2E:4C:49:3F:E3:B6:FD:2D:76:D3%3Bexample.javatpoint.com.kotlingooglesearchlocation Alternatively, follow the directions here: https://developers.google.com/maps/documentation/android/start#get-key Once you have your key (it starts with "AIza"), replace the "google_maps_key" string in this file. --> < string name="google_maps_key" templateMergeStrategy="preserve" translatable="false">AIzaSyCKvLn2KTPKD_-REPLACE-WITH-YOUR-API< /string> < /resources>
MapsActivity.kt
Add the following code in MapsActivity.kt class file.
package example.javatpoint.com.kotlingooglesearchlocation import android.os.Bundle import com.google.android.gms.maps.CameraUpdateFactory import com.google.android.gms.maps.GoogleMap import com.google.android.gms.maps.OnMapReadyCallback import com.google.android.gms.maps.SupportMapFragment import com.google.android.gms.maps.model.LatLng import com.google.android.gms.maps.model.MarkerOptions import android.location.Address import android.location.Geocoder import android.os.Build import android.support.v4.app.FragmentActivity import com.google.android.gms.common.api.GoogleApiClient import com.google.android.gms.maps.model.BitmapDescriptorFactory import com.google.android.gms.maps.model.Marker import com.google.android.gms.location.LocationServices import android.location.Location import android.Manifest import android.content.pm.PackageManager import android.support.v4.content.ContextCompat import android.view.View import android.widget.EditText import android.widget.Toast import com.google.android.gms.common.ConnectionResult import com.google.android.gms.location.LocationListener import com.google.android.gms.location.LocationRequest import java.io.IOException class MapsActivity() : FragmentActivity(), OnMapReadyCallback, LocationListener, GoogleApiClient.ConnectionCallbacks, GoogleApiClient.OnConnectionFailedListener { private var mMap: GoogleMap? = null internal lateinit var mLastLocation: Location internal var mCurrLocationMarker: Marker? = null internal var mGoogleApiClient: GoogleApiClient? = null internal lateinit var mLocationRequest: LocationRequest override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_maps) // Obtain the SupportMapFragment and get notified when the map is ready to be used. val mapFragment = supportFragmentManager .findFragmentById(R.id.map) as SupportMapFragment mapFragment.getMapAsync(this) } override fun onMapReady(googleMap: GoogleMap) { mMap = googleMap if (android.os.Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) { if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) { buildGoogleApiClient() mMap!!.isMyLocationEnabled = true } } else { buildGoogleApiClient() mMap!!.isMyLocationEnabled = true } } @Synchronized protected fun buildGoogleApiClient() { mGoogleApiClient = GoogleApiClient.Builder(this) .addConnectionCallbacks(this) .addOnConnectionFailedListener(this) .addApi(LocationServices.API).build() mGoogleApiClient!!.connect() } override fun onConnected(bundle: Bundle?) { mLocationRequest = LocationRequest() mLocationRequest.interval = 1000 mLocationRequest.fastestInterval = 1000 mLocationRequest.priority = LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) { LocationServices.getFusedLocationProviderClient(this) } } override fun onConnectionSuspended(i: Int) { } override fun onLocationChanged(location: Location) { mLastLocation = location if (mCurrLocationMarker != null) { mCurrLocationMarker!!.remove() } //Place current location marker val latLng = LatLng(location.latitude, location.longitude) val markerOptions = MarkerOptions() markerOptions.position(latLng) markerOptions.title("Current Position") markerOptions.icon(BitmapDescriptorFactory.defaultMarker(BitmapDescriptorFactory.HUE_GREEN)) mCurrLocationMarker = mMap!!.addMarker(markerOptions) //move map camera mMap!!.moveCamera(CameraUpdateFactory.newLatLng(latLng)) mMap!!.animateCamera(CameraUpdateFactory.zoomTo(11f)) //stop location updates if (mGoogleApiClient != null) { LocationServices.getFusedLocationProviderClient(this) } } override fun onConnectionFailed(connectionResult: ConnectionResult) { } fun searchLocation(view: View) { val locationSearch:EditText = findViewById< EditText>(R.id.editText) lateinit var location: String location = locationSearch.text.toString() var addressList: List< Address>? = null if (location == null || location == "") { Toast.makeText(applicationContext,"provide location",Toast.LENGTH_SHORT).show() } else{ val geoCoder = Geocoder(this) try { addressList = geoCoder.getFromLocationName(location, 1) } catch (e: IOException) { e.printStackTrace() } val address = addressList!![0] val latLng = LatLng(address.latitude, address.longitude) mMap!!.addMarker(MarkerOptions().position(latLng).title(location)) mMap!!.animateCamera(CameraUpdateFactory.newLatLng(latLng)) Toast.makeText(applicationContext, address.latitude.toString() + " " + address.longitude, Toast.LENGTH_LONG).show() } } } package example.javatpoint.com.kotlingooglesearchlocation import android.os.Bundle import com.google.android.gms.maps.CameraUpdateFactory import com.google.android.gms.maps.GoogleMap import com.google.android.gms.maps.OnMapReadyCallback import com.google.android.gms.maps.SupportMapFragment import com.google.android.gms.maps.model.LatLng import com.google.android.gms.maps.model.MarkerOptions import android.location.Address import android.location.Geocoder import android.os.Build import android.support.v4.app.FragmentActivity import com.google.android.gms.common.api.GoogleApiClient import com.google.android.gms.maps.model.BitmapDescriptorFactory import com.google.android.gms.maps.model.Marker import com.google.android.gms.location.LocationServices import android.location.Location import android.Manifest import android.content.pm.PackageManager import android.support.v4.content.ContextCompat import android.view.View import android.widget.EditText import android.widget.Toast import com.google.android.gms.common.ConnectionResult import com.google.android.gms.location.LocationListener import com.google.android.gms.location.LocationRequest import java.io.IOException class MapsActivity() : FragmentActivity(), OnMapReadyCallback, LocationListener, GoogleApiClient.ConnectionCallbacks, GoogleApiClient.OnConnectionFailedListener { private var mMap: GoogleMap? = null internal lateinit var mLastLocation: Location internal var mCurrLocationMarker: Marker? = null internal var mGoogleApiClient: GoogleApiClient? = null internal lateinit var mLocationRequest: LocationRequest override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_maps) // Obtain the SupportMapFragment and get notified when the map is ready to be used. val mapFragment = supportFragmentManager .findFragmentById(R.id.map) as SupportMapFragment mapFragment.getMapAsync(this) } override fun onMapReady(googleMap: GoogleMap) { mMap = googleMap if (android.os.Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) { if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) { buildGoogleApiClient() mMap!!.isMyLocationEnabled = true } } else { buildGoogleApiClient() mMap!!.isMyLocationEnabled = true } } @Synchronized protected fun buildGoogleApiClient() { mGoogleApiClient = GoogleApiClient.Builder(this) .addConnectionCallbacks(this) .addOnConnectionFailedListener(this) .addApi(LocationServices.API).build() mGoogleApiClient!!.connect() } override fun onConnected(bundle: Bundle?) { mLocationRequest = LocationRequest() mLocationRequest.interval = 1000 mLocationRequest.fastestInterval = 1000 mLocationRequest.priority = LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) { LocationServices.getFusedLocationProviderClient(this) } } override fun onConnectionSuspended(i: Int) { } override fun onLocationChanged(location: Location) { mLastLocation = location if (mCurrLocationMarker != null) { mCurrLocationMarker!!.remove() } //Place current location marker val latLng = LatLng(location.latitude, location.longitude) val markerOptions = MarkerOptions() markerOptions.position(latLng) markerOptions.title("Current Position") markerOptions.icon(BitmapDescriptorFactory.defaultMarker(BitmapDescriptorFactory.HUE_GREEN)) mCurrLocationMarker = mMap!!.addMarker(markerOptions) //move map camera mMap!!.moveCamera(CameraUpdateFactory.newLatLng(latLng)) mMap!!.animateCamera(CameraUpdateFactory.zoomTo(11f)) //stop location updates if (mGoogleApiClient != null) { LocationServices.getFusedLocationProviderClient(this) } } override fun onConnectionFailed(connectionResult: ConnectionResult) { } fun searchLocation(view: View) { val locationSearch:EditText = findViewById< EditText>(R.id.editText) lateinit var location: String location = locationSearch.text.toString() var addressList: List< Address>? = null if (location == null || location == "") { Toast.makeText(applicationContext,"provide location",Toast.LENGTH_SHORT).show() } else{ val geoCoder = Geocoder(this) try { addressList = geoCoder.getFromLocationName(location, 1) } catch (e: IOException) { e.printStackTrace() } val address = addressList!![0] val latLng = LatLng(address.latitude, address.longitude) mMap!!.addMarker(MarkerOptions().position(latLng).title(location)) mMap!!.animateCamera(CameraUpdateFactory.newLatLng(latLng)) Toast.makeText(applicationContext, address.latitude.toString() + " " + address.longitude, Toast.LENGTH_LONG).show() } } }
AndroidManifest.xml
< ?xml version="1.0" encoding="utf-8"?> < manifest xmlns:android="http://schemas.android.com/apk/res/android" package="example.javatpoint.com.kotlingooglesearchlocation"> < uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> < uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> < uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> < uses-permission android:name="android.permission.INTERNET" /> < application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> < meta-data android:name="com.google.android.geo.API_KEY" android:value="@string/google_maps_key" /> < activity android:name=".MapsActivity" android:label="@string/title_activity_maps"> < intent-filter> < action android:name="android.intent.action.MAIN" /> < category android:name="android.intent.category.LAUNCHER" /> < /intent-filter> < /activity> < /application> < /manifest> < ?xml version="1.0" encoding="utf-8"?> < manifest xmlns:android="http://schemas.android.com/apk/res/android" package="example.javatpoint.com.kotlingooglesearchlocation"> < uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> < uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> < uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> < uses-permission android:name="android.permission.INTERNET" /> < application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> < meta-data android:name="com.google.android.geo.API_KEY" android:value="@string/google_maps_key" /> < activity android:name=".MapsActivity" android:label="@string/title_activity_maps"> < intent-filter> < action android:name="android.intent.action.MAIN" /> < category android:name="android.intent.category.LAUNCHER" /> < /intent-filter> < /activity> < /application> < /manifest>
Output:
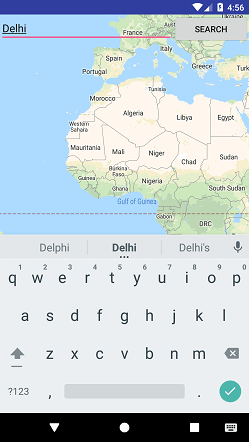
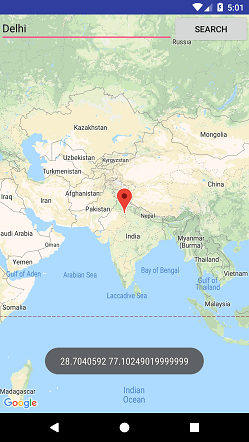