Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Android Custom Toast
In the previous example, Kotlin Android Toast we display a simple toast message. We can also able to customize our message which will show as a toast. In the toast, we can display String text, image or both and customize the position of it.
Kotlin Android Custom Toast Example
In this example, we will display text and an image as a custom toast on clicking the Button.
activity_main.xml
Add the following code in the activity_main.xml file. In this file, we added a Button to perform a click action.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlincustomtoast.MainActivity"> < Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:text="click for custom toast" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.266" /> < /android.support.constraint.ConstraintLayout>
custom_toast.xml
Create a layout file named as custom_toast.xml and add an ImageView and TextView to display as a toast message. Set an image to ImageView and place a message in TextView.
< ?xml version="1.0" encoding="utf-8"?> < LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/linearLayout"> < ImageView android:id="@+id/custom_toast_image" android:layout_width="wrap_content" android:layout_height="wrap_content" android:contentDescription="logo image" android:src="@drawable/jtp_logo"/> < TextView android:id="@+id/custom_toast_message" android:layout_width="wrap_content" android:layout_height="wrap_content" android:contentDescription="To" android:text="JavaTpoint custom Toast" /> < /LinearLayout>
MainActivity.kt
In this MainActivity.kt class, we are getting the View object using the layoutInfleter.infalate() method. Clicking on the button displays the toast message with image and text. The custom layout is added over toast by calling the View using the instance of Toast class (as myToast.view = layout).
package example.javatpoint.com.kotlincustomtoast import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.widget.Toast import android.view.Gravity import kotlinx.android.synthetic.main.activity_main.* import kotlinx.android.synthetic.main.custom_toast.* class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) //getting the View object as defined in the custom_toast.xml file val layout = layoutInflater.inflate(R.layout.custom_toast,linearLayout) button.setOnClickListener(){ val myToast = Toast(applicationContext) myToast.duration = Toast.LENGTH_LONG myToast.setGravity(Gravity.CENTER_VERTICAL, 0, 0) myToast.view = layout//setting the view of custom toast layout myToast.show() } } }
The above code performing the following tasks:
- val myToast = Toast(applicationContext : creates the instance of Toast class.
- myToast.duration = Toast.LENGTH_LONG: set the constant time duration to display the toast message.
- myToast.setGravity(Gravity.CENTER_VERTICAL, 0, 0): set the gravity (position) of toast message.
- myToast.view = layout: add the custom_toast layout to view.
- myToast.show(): display the toast message.
Output:
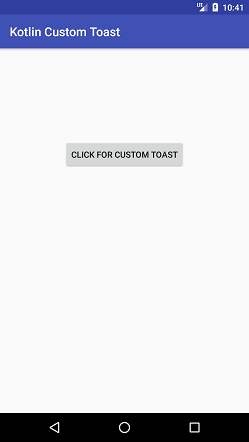
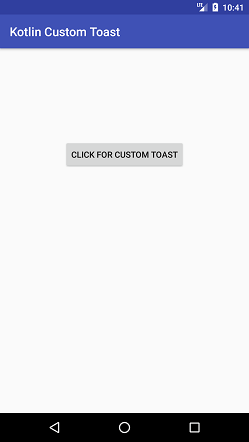