Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Android WebView
Android WebView is a view component which displays the web pages in the application. It uses a WebKit engine to show the web pages. The android.webkit.WebView class is the subclass of AbsoluteLayout class.
The loadUrl() and loadData() methods of WebView are used to load and display the web pages. To learn more about Android WebView go to https://www.javatpoint.com/android-webview-example
There are different ways to load the web page in WebView such as:
Load the HTML content as a string in the class:
val wedData: String = "< html>< body>< h1>Hello, Javatpoint!< /h1>< /body>< /html>" val mimeType: String = "text/html" val utfType: String = "UTF-8" webView.loadData(wedData,mimeType,utfType)
Load the web page (.html, .jsp, etc.) from within the application. In such case, web pages are placed in assets directory.
webView.loadUrl("file:///android_asset/index.html")
Load the web URL inside WebView as:
webView.loadUrl("https://www.javatpoint.com/")
Kotlin Android WebView Example
In this example, we will load the web URL in the WebView component and override the URL.
Directory structure
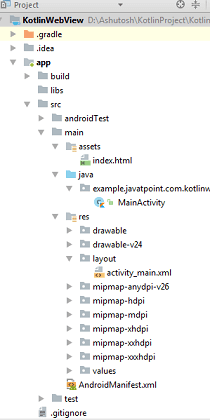
activity_main.xml
Add the WebView component in the activity_main.xml file.
< ?xml version="1.0" encoding="utf-8"?> < LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinwebview.MainActivity"> < WebView android:id="@+id/webView" android:layout_width="fill_parent" android:layout_height="fill_parent"/> < /LinearLayout>
AndroidMenifest.xml
In the AndroidMenifest.xml file, add the Internet permission to connect the network connection.
< uses-permission android:name="android.permission.INTERNET"/> < ?xml version="1.0" encoding="utf-8"?> < manifest xmlns:android="http://schemas.android.com/apk/res/android" package="example.javatpoint.com.kotlinwebview"> < uses-permission android:name="android.permission.INTERNET"/> < application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> < activity android:name=".MainActivity"> < intent-filter> < action android:name="android.intent.action.MAIN" /> < category android:name="android.intent.category.LAUNCHER" /> < /intent-filter> < /activity> < /application> < /manifest>
MainActivity.kt
Add the following code in the MainActivity.kt class. In this class, we are using the loadUrl() method of WebView to load web URL.
Create a MyWebViewClient class that extends the WebViewClient that override the shouldOverrideUrlLoading() method.
package example.javatpoint.com.kotlinwebview import android.app.Activity import android.os.Build import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.support.annotation.RequiresApi import android.webkit.WebResourceError import android.webkit.WebResourceRequest import android.webkit.WebView import android.webkit.WebViewClient import android.widget.Toast import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) /* val wedData: String = "< html>< body>< h1>Hello, Javatpoint!< /h1>< /body>< /html>" val mimeType: String = "text/html" val utfType: String = "UTF-8" webView.loadData(wedData,mimeType,utfType)*/ /* val myWebUrl: String = "file:///android_asset/index.html" webView.loadUrl(myWebUrl)*/ webView.webViewClient = MyWebViewClient(this) webView.loadUrl("https://www.javatpoint.com/") } class MyWebViewClient internal constructor(private val activity: Activity) : WebViewClient() { @RequiresApi(Build.VERSION_CODES.LOLLIPOP) override fun shouldOverrideUrlLoading(view: WebView?, request: WebResourceRequest?): Boolean { val url: String = request?.url.toString(); view?.loadUrl(url) return true } override fun shouldOverrideUrlLoading(webView: WebView, url: String): Boolean { webView.loadUrl(url) return true } override fun onReceivedError(view: WebView, request: WebResourceRequest, error: WebResourceError) { Toast.makeText(activity, "Got Error! $error", Toast.LENGTH_SHORT).show() } } }
Output:
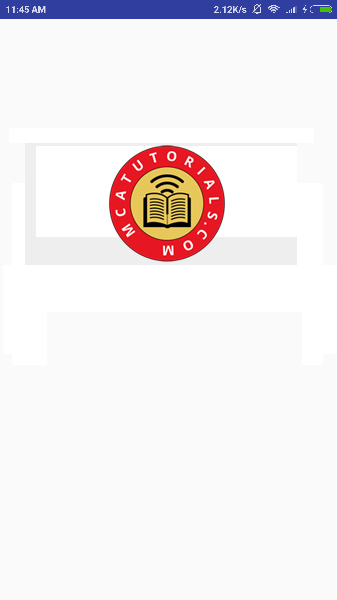