Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Android XML Parsing using SAX Parser
XML document is commonly used to share the data on the internet. The data provided in the XML format are able to update frequently and parsing them is a common task for network-based apps.
In Android, there are three types of XML parsers to parse the XML data and read them in android applications, these are:
- DOM Parser
- SAX Parser
- XMLPullParser
In Android, SAX (Simple API for XML) is widely used API for XML parsing. The SAX parser will examines an XML document, character by character and translates it into a series of events such as startElement(), endElement() and characters(). To read and parse the XML data using SAX parser, we need to create an instance of SAXParserFactory, SAXParser and DefaultHandler objects in android applications.
Example of XML Parsing using SAX Parser
In this example, we will parse the XML data using SAX parser and display them into ListView.
activity_main.xml
Add the ListView in the activity_main.xml layout.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinxmlparsingusingsaxparser.MainActivity"> < ListView android:id="@+id/listView" android:layout_width="match_parent" android:layout_height="match_parent"> < /ListView> < /android.support.constraint.ConstraintLayout>
empdetail.xml
Create the XML document empdetail.xml in assets directory to parse the data using SAX parser.
< ?xml version="1.0" encoding="utf-8"?> < records> < employee> < name>Sachin Kumar< /name> < salary>50000< /salary> < designation>Developer< /designation> < /employee> < employee> < name>Rahul Kumar< /name> < salary>60000< /salary> < designation>Team Leader< /designation> < /employee> < employee> < name>John Mike< /name> < salary>70000< /salary> < designation>Manager< /designation> < /employee> < employee> < name>Ajay Kumar< /name> < salary>45000< /salary> < designation>Developer< /designation> < /employee> < employee> < name>Toni Nayer< /name> < salary>55000< /salary> < designation>Marketing< /designation> < /employee> < employee> < name>Mr Bony< /name> < salary>42000< /salary> < designation>Sales< /designation> < /employee> < employee> < name>Raj Kumar< /name> < salary>30000< /salary> < designation>Production< /designation> < /employee> < employee> < name>Rahul Kumar< /name> < salary>60000< /salary> < designation>Team Leader< /designation> < /employee> < employee> < name>John Mike< /name> < salary>70000< /salary> < designation>Manager< /designation> < /employee> < employee> < name>Sachin Kumar< /name> < salary>50000< /salary> < designation>Developer< /designation> < /employee> < employee> < name>Rahul Kumar< /name> < salary>60000< /salary> < designation>Team Leader< /designation> < /employee> < employee> < name>John Mike< /name> < salary>70000< /salary> < designation>Manager< /designation> < /employee> < /records>
custom_list.xml
Create a custom layout to display the list of data into ListView.
< ?xml version="1.0" encoding="utf-8"?> < LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="horizontal"> < LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="1" android:orientation="vertical"> < TextView android:id="@+id/name" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="name" android:textStyle="bold" android:layout_marginLeft="15dp" android:layout_marginStart="15dp" android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" /> < TextView android:id="@+id/salary" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="salary" android:layout_marginLeft="15dp" android:layout_marginStart="15dp" android:layout_marginTop="5dp" android:textSize="16sp"/> < TextView android:id="@+id/designation" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="designation" android:layout_marginLeft="15dp" android:layout_marginStart="15dp" android:layout_marginTop="5dp" android:textSize="16sp"/> < /LinearLayout> < /LinearLayout>
MainActivity.kt
Add the following code to read and parse the XML data using SAX parser. Create the instance of SAXParserFactory, SAXParser and DefaultHandler objects.
The HashMap< String, String> is used to read the data from XML document and add them into ArrayList().
package example.javatpoint.com.kotlinxmlparsingusingsaxparser import android.support.v7.app.AppCompatActivity import android.os.Bundle import org.xml.sax.helpers.DefaultHandler import javax.xml.parsers.SAXParserFactory import android.widget.ListView import android.widget.SimpleAdapter import org.xml.sax.SAXException import java.io.IOException import java.util.ArrayList import java.util.HashMap import javax.xml.parsers.ParserConfigurationException import javax.xml.parsers.SAXParser class MainActivity : AppCompatActivity() { internal var empList = ArrayList< HashMap>() internal var empData = HashMap< String, String>() override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val lv:ListView = findViewById(R.id.listView) try { //instancing the SAXParserFactory class val parserFactory:SAXParserFactory = SAXParserFactory.newInstance() //instancing the SAXParser class val saxParser:SAXParser = parserFactory.newSAXParser() val defaultHandler= object : DefaultHandler() { var currentValue = "" var currentElement = false //overriding the startElement() method of DefaultHandler override fun startElement(uri: String, localName: String, qName: String, attributes: org.xml.sax.Attributes) { currentElement = true currentValue = "" if (localName == "employee") { empData = HashMap() } } //overriding the endElement() method of DefaultHandler override fun endElement(uri: String, localName: String, qName: String) { currentElement = false if (localName.equals("name", ignoreCase = true)) empData["name"] = currentValue else if (localName.equals("salary", ignoreCase = true)) empData["salary"] = currentValue else if (localName.equals("designation", ignoreCase = true)) empData["designation"] = currentValue else if (localName.equals("employee", ignoreCase = true)) empList.add(empData) } //overriding the characters() method of DefaultHandler override fun characters(ch: CharArray, start: Int, length: Int) { if (currentElement) { currentValue = currentValue + String(ch, start, length) } } } val istream = assets.open("empdetail.xml") saxParser.parse(istream, defaultHandler) //creating Adapter class to access the custom list val adapter = SimpleAdapter(this@MainActivity, empList, R.layout.custom_list, arrayOf("name", "salary", "designation"), intArrayOf(R.id.name, R.id.salary, R.id.designation)) lv.adapter = adapter } catch (e: IOException) { e.printStackTrace() } catch (e: ParserConfigurationException) { e.printStackTrace() } catch (e: SAXException) { e.printStackTrace() } } }
Output:
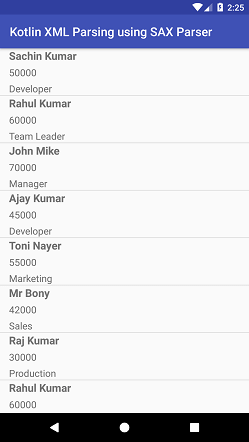