Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Android Context Menu
Android Context Menu is a floating menu which appears when a user performs a long-click on an element. The action performs on context menu affect only on the selected content. Context Menu can be implemented on any view, but it is mostly used with items of ListView, GridView or other view collections.
Context Menu is created by overriding the onCreateContextMenu() function. The menu resource is inflated by and calling the inflate() method of MenuInflater class. To act on menu items, override the onContextItemSelected () function.
Kotlin Android Context Menu Example
In this example, we will add a ListView and implements the context menu on its items. Performing the long-click on the list item shows the context menu items on which we can perform the relevant action.
Create an android project and select the Basic Activity. This activity auto-generates codes for the menu option.
activity_main.xml
Add the following code in the activity_main.xml file in layout directory. This code is auto-generated while creating Basic Activity.
< ?xml version="1.0" encoding="utf-8"?> < android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlincontextmenu.MainActivity"> < android.support.design.widget.AppBarLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:theme="@style/AppTheme.AppBarOverlay"> < android.support.v7.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:background="?attr/colorPrimary" app:popupTheme="@style/AppTheme.PopupOverlay" /> < /android.support.design.widget.AppBarLayout> < include layout="@layout/content_main" /> < /android.support.design.widget.CoordinatorLayout>
content_main.xml
Add the following code in the content_main.xml file in layout directory. In this file, we added a ListView.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" app:layout_behavior="@string/appbar_scrolling_view_behavior" tools:context="example.javatpoint.com.kotlincontextmenu.MainActivity" tools:showIn="@layout/activity_main"> < ListView android:id="@+id/listView" android:layout_width="match_parent" android:layout_height="match_parent" /> < /android.support.constraint.ConstraintLayout>
strings.xml
Add the following code in the strings.xml file.
< resources> < string name="app_name">Kotlin ContextMenu< /string> < string name="action_call">Call< /string> < string name="action_sms">Sms< /string> < /resources>
menu_main.xml
Add the following code in the menu_main.xml file in menu directory. Add the item tag which creates the menu item for the context menu.
< menu xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" tools:context="example.javatpoint.com.kotlincontextmenu.MainActivity"> < item android:id="@+id/call" android:orderInCategory="100" android:title="@string/action_call" app:showAsAction="never" /> < item android:id="@+id/sms" android:orderInCategory="100" android:title="@string/action_sms" app:showAsAction="never" /> < /menu>
MainActivity.kt
Add the following code in the MianActivity.kt class. In this class, we create a list view and implement the context menu on its items. To add the list items for context menu the registerForContextMenu(list) method is used. To create the context menu override the onCreateContextMenu() and call the inflate() method of MenuInflater class.
To perform action on each items of context menu override the onContextItemSelected() function.
package example.javatpoint.com.kotlincontextmenu import android.os.Bundle import android.support.v7.app.AppCompatActivity import android.view.ContextMenu import android.view.MenuItem import android.view.View import android.widget.ArrayAdapter import kotlinx.android.synthetic.main.activity_main.* import kotlinx.android.synthetic.main.content_main.* import android.widget.Toast class MainActivity : AppCompatActivity() { private val contact = arrayOf("Akash","Vikash","John","Rahul","Ajay") override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) setSupportActionBar(toolbar) val arrayAdapter: ArrayAdapter = ArrayAdapter (this,android.R.layout.simple_list_item_1,contact) listView.adapter = arrayAdapter registerForContextMenu(listView) } override fun onCreateContextMenu(menu: ContextMenu?, v: View?, menuInfo: ContextMenu.ContextMenuInfo?) { super.onCreateContextMenu(menu, v, menuInfo) val inflater = menuInflater inflater.inflate(R.menu.menu_main, menu) } override fun onContextItemSelected(item: MenuItem?): Boolean { return when (item!!.itemId) { R.id.call ->{ Toast.makeText(applicationContext, "call code", Toast.LENGTH_LONG).show() return true } R.id.sms ->{ Toast.makeText(applicationContext, "sms code", Toast.LENGTH_LONG).show() return true } else -> super.onOptionsItemSelected(item) } } }
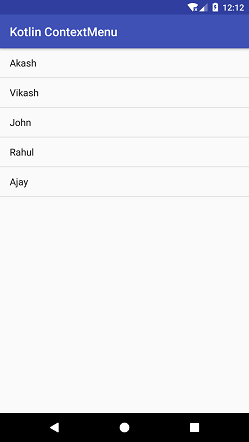
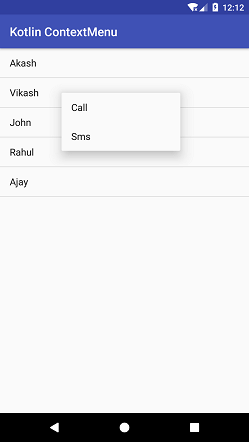
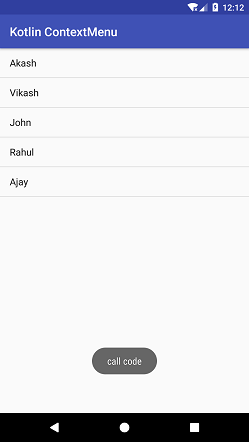