Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
JSON Parsing using URL
JSON refers to JavaScript Object Notation, and it is a programming language. JSON is used to parse the data between the server and the client. It is minimal, textual and a subset of JavaScript. It is the alternative to XML parsing.
The advantage of JSON over XML
- JSON is faster and easier than XML for AJAX applications.
- Unlike XML, it is shorter and quicker to read and write.
- It uses an array.
JSON Object
A JSON object contains key/value pairs like a map. The keys are strings and the values are the JSON types. A comma separates keys and values (,). The curly brace {} represents the JSON object.
Kotlin JSON Parsing using URL Example
In this example, we parse the JSON data from URL and bind them into a ListView. The JSON data contains the "id" "name" "email".
JSON data index.html
Create a JSON file index.html.
{"info":[ {"name":"Ajay","id":"111","email":"ajay@gmail.com"}, {"name":"John","id":"112","email":"john@gmail.com"}, {"name":"Rahul","id":"113","email":"rahul@gmail.com"}, {"name":"Maich","id":"114","email":"maich@gmail.com"}, {"name":"Vikash","id":"115","email":"vikash@gmail.com"}, {"name":"Mayank","id":"116","email":"mayank@gmail.com"}, {"name":"Prem","id":"117","email":"prem@gmail.com"}, {"name":"Chandan","id":"118","email":"chandan@gmail.com"}, {"name":"Soham","id":"119","email":"soham@gmail.com"}, {"name":"Mukesh","id":"120","email":"mukesh@gmail.com"}, {"name":"Ajad","id":"121","email":"ajad@gmail.com"} ] } {"info":[ {"name":"Ajay","id":"111","email":"ajay@gmail.com"}, {"name":"John","id":"112","email":"john@gmail.com"}, {"name":"Rahul","id":"113","email":"rahul@gmail.com"}, {"name":"Maich","id":"114","email":"maich@gmail.com"}, {"name":"Vikash","id":"115","email":"vikash@gmail.com"}, {"name":"Mayank","id":"116","email":"mayank@gmail.com"}, {"name":"Prem","id":"117","email":"prem@gmail.com"}, {"name":"Chandan","id":"118","email":"chandan@gmail.com"}, {"name":"Soham","id":"119","email":"soham@gmail.com"}, {"name":"Mukesh","id":"120","email":"mukesh@gmail.com"}, {"name":"Ajad","id":"121","email":"ajad@gmail.com"} ] }
While executing the JSON file (index.html) looks like as:

activity_main.xml
Add the ListView in the activity_main.xml layout file.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinjsonparsing.MainActivity"> < ListView android:id="@+id/listView" android:layout_width="match_parent" android:layout_height="match_parent"> < /ListView> < /android.support.constraint.ConstraintLayout> < ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinjsonparsing.MainActivity"> < ListView android:id="@+id/listView" android:layout_width="match_parent" android:layout_height="match_parent"> < /ListView> < /android.support.constraint.ConstraintLayout>
build.gradle
Add the following okhttp dependency in the build.gradle file.
compile 'com.squareup.okhttp3:okhttp:3.8.1' compile 'com.squareup.okhttp3:okhttp:3.8.1'
Model.kt
Create a data model class Model.kt which includes the information String "id", String "name" and String "email".
package example.javatpoint.com.kotlinjsonparsing public class Model{ lateinit var id:String lateinit var name:String lateinit var email:String constructor(id: String,name:String,email:String) { this.id = id this.name = name this.email = email } constructor() } package example.javatpoint.com.kotlinjsonparsing public class Model{ lateinit var id:String lateinit var name:String lateinit var email:String constructor(id: String,name:String,email:String) { this.id = id this.name = name this.email = email } constructor() }
adapter_layout.xml
Create an adapter_layout.xml file in the layout directory which contains the row items for ListView.
< ?xml version="1.0" encoding="utf-8"?> < LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/linearLayout" android:padding="5dp" android:orientation="vertical"> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/tvId" android:layout_margin="5dp" android:textSize="16dp"/> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/tvName" android:textSize="16dp" android:layout_margin="5dp"/> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/tvEmail" android:layout_margin="5dp" android:textSize="16dp"/> < /LinearLayout> < ?xml version="1.0" encoding="utf-8"?> < LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/linearLayout" android:padding="5dp" android:orientation="vertical"> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/tvId" android:layout_margin="5dp" android:textSize="16dp"/> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/tvName" android:textSize="16dp" android:layout_margin="5dp"/> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/tvEmail" android:layout_margin="5dp" android:textSize="16dp"/> < /LinearLayout>
CustomAdapter.kt
Create a custom adapter class CustomAdapter.kt and extend BaseAdapter to handle the custom ListView.
package example.javatpoint.com.kotlinjsonparsing import android.content.Context import android.view.LayoutInflater import android.view.View import android.view.ViewGroup import android.widget.BaseAdapter import android.widget.LinearLayout import android.widget.TextView class CustomAdapter(context: Context,arrayListDetails:ArrayList< Model>) : BaseAdapter(){ private val layoutInflater: LayoutInflater private val arrayListDetails:ArrayList< Model> init { this.layoutInflater = LayoutInflater.from(context) this.arrayListDetails=arrayListDetails } override fun getCount(): Int { return arrayListDetails.size } override fun getItem(position: Int): Any { return arrayListDetails.get(position) } override fun getItemId(position: Int): Long { return position.toLong() } override fun getView(position: Int, convertView: View?, parent: ViewGroup): View? { val view: View? val listRowHolder: ListRowHolder if (convertView == null) { view = this.layoutInflater.inflate(R.layout.adapter_layout, parent, false) listRowHolder = ListRowHolder(view) view.tag = listRowHolder } else { view = convertView listRowHolder = view.tag as ListRowHolder } listRowHolder.tvName.text = arrayListDetails.get(position).name listRowHolder.tvEmail.text = arrayListDetails.get(position).email listRowHolder.tvId.text = arrayListDetails.get(position).id return view } } private class ListRowHolder(row: View?) { public val tvName: TextView public val tvEmail: TextView public val tvId: TextView public val linearLayout: LinearLayout init { this.tvId = row?.findViewById< TextView>(R.id.tvId) as TextView this.tvName = row?.findViewById< TextView>(R.id.tvName) as TextView this.tvEmail = row?.findViewById< TextView>(R.id.tvEmail) as TextView this.linearLayout = row?.findViewById< LinearLayout>(R.id.linearLayout) as LinearLayout } } package example.javatpoint.com.kotlinjsonparsing import android.content.Context import android.view.LayoutInflater import android.view.View import android.view.ViewGroup import android.widget.BaseAdapter import android.widget.LinearLayout import android.widget.TextView class CustomAdapter(context: Context,arrayListDetails:ArrayList< Model>) : BaseAdapter(){ private val layoutInflater: LayoutInflater private val arrayListDetails:ArrayList< Model> init { this.layoutInflater = LayoutInflater.from(context) this.arrayListDetails=arrayListDetails } override fun getCount(): Int { return arrayListDetails.size } override fun getItem(position: Int): Any { return arrayListDetails.get(position) } override fun getItemId(position: Int): Long { return position.toLong() } override fun getView(position: Int, convertView: View?, parent: ViewGroup): View? { val view: View? val listRowHolder: ListRowHolder if (convertView == null) { view = this.layoutInflater.inflate(R.layout.adapter_layout, parent, false) listRowHolder = ListRowHolder(view) view.tag = listRowHolder } else { view = convertView listRowHolder = view.tag as ListRowHolder } listRowHolder.tvName.text = arrayListDetails.get(position).name listRowHolder.tvEmail.text = arrayListDetails.get(position).email listRowHolder.tvId.text = arrayListDetails.get(position).id return view } } private class ListRowHolder(row: View?) { public val tvName: TextView public val tvEmail: TextView public val tvId: TextView public val linearLayout: LinearLayout init { this.tvId = row?.findViewById< TextView>(R.id.tvId) as TextView this.tvName = row?.findViewById< TextView>(R.id.tvName) as TextView this.tvEmail = row?.findViewById< TextView>(R.id.tvEmail) as TextView this.linearLayout = row?.findViewById< LinearLayout>(R.id.linearLayout) as LinearLayout } }
MainActivity.kt
Add the following code in MainActivity.kt class file. This class read the JSON data in the form of a JSON object. Using the JSON object, we read the JSON array data. The JSON data are bind in ArrayList.
package example.javatpoint.com.kotlinjsonparsing import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.view.View import android.widget.ListView import android.widget.ProgressBar import okhttp3.* import org.json.JSONArray import org.json.JSONObject import java.io.IOException import kotlin.collections.ArrayList class MainActivity : AppCompatActivity() { lateinit var progress:ProgressBar lateinit var listView_details: ListView var arrayList_details:ArrayList< Model> = ArrayList(); //OkHttpClient creates connection pool between client and server val client = OkHttpClient() override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) progress = findViewById(R.id.progressBar) progress.visibility = View.VISIBLE listView_details = findViewById< ListView>(R.id.listView) as ListView run("http://10.0.0.7:8080/jsondata/index.html") } fun run(url: String) { progress.visibility = View.VISIBLE val request = Request.Builder() .url(url) .build() client.newCall(request).enqueue(object : Callback { override fun onFailure(call: Call, e: IOException) { progress.visibility = View.GONE } override fun onResponse(call: Call, response: Response) { var str_response = response.body()!!.string() //creating json object val json_contact:JSONObject = JSONObject(str_response) //creating json array var jsonarray_info:JSONArray= json_contact.getJSONArray("info") var i:Int = 0 var size:Int = jsonarray_info.length() arrayList_details= ArrayList(); for (i in 0.. size-1) { var json_objectdetail:JSONObject=jsonarray_info.getJSONObject(i) var model:Model= Model(); model.id=json_objectdetail.getString("id") model.name=json_objectdetail.getString("name") model.email=json_objectdetail.getString("email") arrayList_details.add(model) } runOnUiThread { //stuff that updates ui val obj_adapter : CustomAdapter obj_adapter = CustomAdapter(applicationContext,arrayList_details) listView_details.adapter=obj_adapter } progress.visibility = View.GONE } }) } } package example.javatpoint.com.kotlinjsonparsing import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.view.View import android.widget.ListView import android.widget.ProgressBar import okhttp3.* import org.json.JSONArray import org.json.JSONObject import java.io.IOException import kotlin.collections.ArrayList class MainActivity : AppCompatActivity() { lateinit var progress:ProgressBar lateinit var listView_details: ListView var arrayList_details:ArrayList< Model> = ArrayList(); //OkHttpClient creates connection pool between client and server val client = OkHttpClient() override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) progress = findViewById(R.id.progressBar) progress.visibility = View.VISIBLE listView_details = findViewById< ListView>(R.id.listView) as ListView run("http://10.0.0.7:8080/jsondata/index.html") } fun run(url: String) { progress.visibility = View.VISIBLE val request = Request.Builder() .url(url) .build() client.newCall(request).enqueue(object : Callback { override fun onFailure(call: Call, e: IOException) { progress.visibility = View.GONE } override fun onResponse(call: Call, response: Response) { var str_response = response.body()!!.string() //creating json object val json_contact:JSONObject = JSONObject(str_response) //creating json array var jsonarray_info:JSONArray= json_contact.getJSONArray("info") var i:Int = 0 var size:Int = jsonarray_info.length() arrayList_details= ArrayList(); for (i in 0.. size-1) { var json_objectdetail:JSONObject=jsonarray_info.getJSONObject(i) var model:Model= Model(); model.id=json_objectdetail.getString("id") model.name=json_objectdetail.getString("name") model.email=json_objectdetail.getString("email") arrayList_details.add(model) } runOnUiThread { //stuff that updates ui val obj_adapter : CustomAdapter obj_adapter = CustomAdapter(applicationContext,arrayList_details) listView_details.adapter=obj_adapter } progress.visibility = View.GONE } }) } }
AndroidManifest.xml
Add the Internet permission in AndroidManifest.xml file.
< uses-permission android:name="android.permission.INTERNET"/> < uses-permission android:name="android.permission.INTERNET"/>
Output:
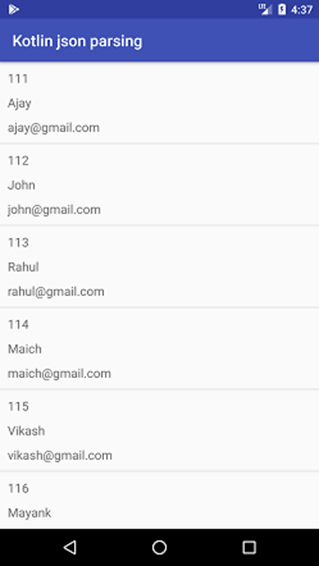