Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Android ListView
Android ListView is a view component that contains the list of items and is displayed in a scrollable list. The list items are automatically added to the list using the Adapter class. The Adapter class bridges the list of data between an AdapterViews other Views components (ListView, ScrollView, etc.).
Call the setAdapter(adapter) to connect an adapter with the list to display the items in list view. To know more about Android ListView go to https://www.javatpoint.com/android-listview-exampler
Kotlin Android ListView Example
In this example, we will create a ListView and perform click action on the list items. The items of a list can be created in the class file or in a separate file such as in strings.xml.
For example, create list items in a class file and add it on ArrayAdapter class:
val language = arrayOf< String>("C","C++","Java",".Net","Kotlin","Ruby","Rails","Python","Java Script","Php","Ajax","Perl","Hadoop") override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val arrayAdapter = ArrayAdapter(this,android.R.layout.simple_list_item_1,language) listView.adapter = arrayAdapter }
Creating list items in a separate strings.xml file and added it on ArrayAdapter class:
< string-array name="technology_list"> < item>C< /item> < item>C++< /item> < item>Java< /item> < item>.Net< /item> < /string-array> val language:Array< String> = resources.getStringArray(R.array.technology_list) val arrayAdapter = ArrayAdapter< String>(this,android.R.layout.simple_list_item_1,language) listView.adapter = arrayAdapter
activity_main.xml
Add a ListView component to display the list of items in the activity_main.xml file.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinlistview.MainActivity"> < ListView android:id="@+id/listView" android:layout_width="match_parent" android:layout_height="fill_parent" /> < /android.support.constraint.ConstraintLayout>
strings.xml
In the strings.xml file, create a string-array and add the list items in the item tag.
< resources> < string name="app_name">Kotlin ListView < string-array name="technology_list"> < item>C< /item> < item>C++< /item> < item>Java< /item> < item>.Net< /item> < item>Kotlin< /item> < item>Ruby< /item> < item>Rails< /item> < item>Python< /item> < item>Java Script< /item> < item>Php< /item> < item>Ajax< /item> < item>Perl< /item> < item>Hadoop< /item> < /string-array> < /resources>
MainActivity.kt
Add the following code in the MainActivity.kt class file. In this class, we are getting the list of items from the strings.xml file using resource.getStringArray() method. The ArrayAdapter class initialized with the application context, sets the resource type of list and array items as an argument. The listView.adapter = arrayAdapter sets the adapter to the ListView. To perform the click action on items of the list, call the OnItemClickListener{}.
package example.javatpoint.com.kotlinlistview import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.widget.* import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { // val language = arrayOf< String>("C","C++","Java",".Net","Kotlin","Ruby","Rails","Python","Java Script","Php","Ajax","Perl","Hadoop") override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val language:Array< String> = resources.getStringArray(R.array.technology_list) val arrayAdapter = ArrayAdapter< String>(this,android.R.layout.simple_list_item_1,language) listView.adapter = arrayAdapter listView.onItemClickListener = AdapterView.OnItemClickListener { adapterView, view, position, id -> val selectedItem = adapterView.getItemAtPosition(position) as String val itemIdAtPos = adapterView.getItemIdAtPosition(position) Toast.makeText(applicationContext,"click item $selectedItem its position $itemIdAtPos",Toast.LENGTH_SHORT).show() } } }
Output:
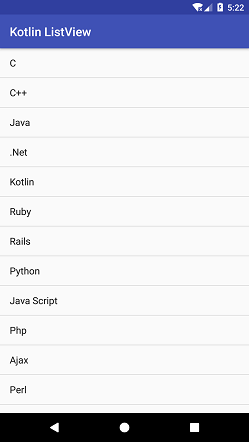
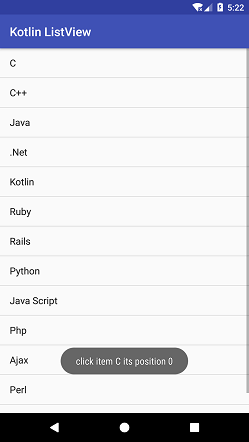