Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Android Options Menu
Android Options Menu is the collection of menu items for an activity. Options menu allows placing actions that impact globally on the application.
Options Menu is created by overriding the onCreateOptionsMenu() function. The menu resource is inflated by and calling the inflate() method of MenuInflater class. To act on menu items, override the onOptionsItemSelected() function.
Kotlin Android Options Menu Example
In this example, we will add the options menu items on the action bar. Clicking on the menu shows the option menu items on which we can perform the relevant action.
Create an android project and select the Basic Activity. This activity auto generates codes for menu option and Toolbar.
activity_main.xml
Add the following code in the activity_main.xml file in layout directory. This code is auto-generated while creating Basic Activity.
< ?xml version="1.0" encoding="utf-8"?> < android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinoptionsmenu.MainActivity"> < android.support.design.widget.AppBarLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:theme="@style/AppTheme.AppBarOverlay"> < android.support.v7.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:background="?attr/colorPrimary" app:popupTheme="@style/AppTheme.PopupOverlay" /> < /android.support.design.widget.AppBarLayout> < include layout="@layout/content_main" /> < /android.support.design.widget.CoordinatorLayout>
content_main.xml
Add the following code in the content_main.xml file in layout directory. In this layout, you can place your UI components.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" app:layout_behavior="@string/appbar_scrolling_view_behavior" tools:context="example.javatpoint.com.kotlinoptionsmenu.MainActivity" tools:showIn="@layout/activity_main"> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" /> < /android.support.constraint.ConstraintLayout>
strings.xml
Add the following code in the strings.xml file.
< resources> < string name="app_name">Kotlin OptionsMenu< /string> < string name="action_settings">Settings< /string> < string name="action_share">Share< /string> < string name="action_exit">Exit< /string> < /resources>
menu_main.xml
Add the following code in the menu_main.xml file in menu directory. Add the item tag which creates the menu item for options menu.
< menu xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" tools:context="example.javatpoint.com.kotlinoptionsmenu.MainActivity"> < item android:id="@+id/action_settings" android:orderInCategory="100" android:title="@string/action_settings"/> < item android:id="@+id/action_share" android:title="@string/action_share" app:showAsAction="never"/> < item android:id="@+id/action_exit" android:title="@string/action_exit" app:showAsAction="never"/> < /menu>
MainActivity.kt
Add the following code in the MainActivity.kt class. In this class, we override the function onCreateOptionsMenu() and call the inflate() of MenuInflater class which inflates the menu and adds items to the action bar.
To perform action on each items of options, menu overrides the onOptionsItemSelected() function.
package example.javatpoint.com.kotlinoptionsmenu import android.os.Bundle import android.support.v7.app.AppCompatActivity import android.view.Menu import android.view.MenuItem import android.widget.Toast import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) setSupportActionBar(toolbar) } override fun onCreateOptionsMenu(menu: Menu): Boolean { // Inflate the menu; this adds items to the action bar if it is present. menuInflater.inflate(R.menu.menu_main, menu) return true } override fun onOptionsItemSelected(item: MenuItem): Boolean { return when (item.itemId) { R.id.action_settings -> { Toast.makeText(applicationContext, "click on setting", Toast.LENGTH_LONG).show() true } R.id.action_share ->{ Toast.makeText(applicationContext, "click on share", Toast.LENGTH_LONG).show() return true } R.id.action_exit ->{ Toast.makeText(applicationContext, "click on exit", Toast.LENGTH_LONG).show() return true } else -> super.onOptionsItemSelected(item) } } }
Output:
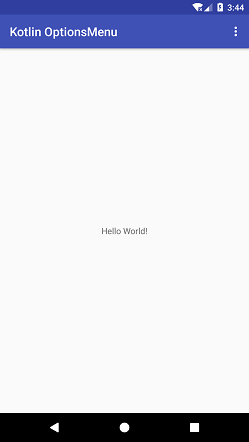
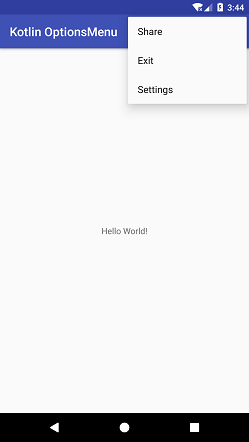
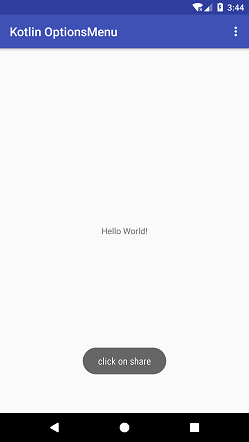
Creating Option Menu Using Images on Action Bar
We can also display the Options Menu as images on the Action Bar. To set image in the Options Menu add the icon attribute in the item tag as give below.
menu_main.xml
< menu xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" tools:context="example.javatpoint.com.kotlinoptionmenu.MainActivity"> < item android:id="@+id/action_settings" android:orderInCategory="100" android:title="@string/action_settings" android:icon="@android:drawable/btn_star" /> < item android:id="@+id/action_share" android:title="@string/action_share" app:showAsAction="ifRoom" android:icon="@drawable/shareimage"/> < item android:id="@+id/action_exit" android:title="@string/action_exit" app:showAsAction="ifRoom" android:icon="@drawable/exitimage"/> < /menu>