Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Android TabLayout with FrameLayout
Android TabLayout is a Layout which is used to build horizontal tabs. In the tutorial, Android TabLayout with ViewPager we create the tabs of TabLayout using the newTab() method but the tabs are also be implemented using android.support.design.widget.TabItem in layout activity.
The title and icon of tabs are provided by implementing the text and icon attribute respectively.
To provide the space for each tab of TabLayout, we can use the FrameLayout. FrameLayout is designed to cover the area on the screen to display a single item.
Kotlin Android TabLayout with FrameLayout Example
In this example, we will create the TabLayout using TabItem with FrameLayout.
build.gradle
Add the following dependency in the build.gradle file.
implementation fileTree(dir: 'libs', include: ['*.jar']) implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk8:$kotlin_version" implementation 'com.android.support:appcompat-v7:26.1.0' implementation 'com.android.support.constraint:constraint-layout:1.1.3' implementation 'com.android.support:support-v4:26.1.0' testImplementation 'junit:junit:4.12' implementation 'com.android.support:design:26.1.0'
activity_main.xml
Add the TabLayout, TabItem, and FrameLayout in the activity_main.xml layout file.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlintablayoutwithframelayout.MainActivity"> < android.support.design.widget.TabLayout android:id="@+id/tabLayout" android:layout_width="match_parent" android:layout_height="wrap_content" android:background="#00a294" app:tabTextColor="@android:color/background_light"> < android.support.design.widget.TabItem android:id="@+id/tabJava" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Java" /> < android.support.design.widget.TabItem android:id="@+id/tabAndroid" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Android" /> < android.support.design.widget.TabItem android:id="@+id/tabKotlin" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Kotlin" /> < android.support.design.widget.TabItem android:id="@+id/tabPhp" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Php" /> < android.support.design.widget.TabItem android:id="@+id/tabPython" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Python" /> < /android.support.design.widget.TabLayout> < FrameLayout android:id="@+id/frameLayout" android:layout_width="match_parent" android:layout_height="match_parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/tabLayout"> < /FrameLayout> < /android.support.constraint.ConstraintLayout>
strings.xml
< resources> < string name="app_name">Kotlin TabLayout with FrameLayout< /string> < string name="java_fragment">Java fragment< /string> < string name="android_fragment">Android fragment< /string> < string name="kotlin_fragment">Kotlin fragment< /string> < string name="php_fragment">Php fragment< /string> < string name="python_fragment">Python fragment< /string> < /resources>
colors.xml
< ?xml version="1.0" encoding="utf-8"?> < resources> < color name="colorPrimary">#03DAC6< /color> < color name="colorPrimaryDark">#aeded9< /color> < color name="colorAccent">#00a294< /color> < /resources>
MainActivity.kt
Add the following code in MainActivity.kt class. In this class, we create the instance of TabLayout and FrameLayout. Calling the addOnTabSelectedListener() listener of TabLayout and overrides its methods.
package example.javatpoint.com.kotlintablayoutwithframelayout import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.support.design.widget.TabLayout import android.support.v4.app.Fragment import android.support.v4.app.FragmentManager import android.support.v4.app.FragmentTransaction import android.widget.FrameLayout class MainActivity : AppCompatActivity() { var tabLayout: TabLayout? = null var frameLayout: FrameLayout? = null var fragment: Fragment? = null var fragmentManager: FragmentManager? = null var fragmentTransaction: FragmentTransaction? = null override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) tabLayout = findViewById< TabLayout>(R.id.tabLayout) frameLayout = findViewById< FrameLayout>(R.id.frameLayout) fragment = JavaFragment() fragmentManager = supportFragmentManager fragmentTransaction = fragmentManager!!.beginTransaction() fragmentTransaction!!.replace(R.id.frameLayout, fragment) fragmentTransaction!!.setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN) fragmentTransaction!!.commit() //adding listener for tab select tabLayout!!.addOnTabSelectedListener(object : TabLayout.OnTabSelectedListener { override fun onTabSelected(tab: TabLayout.Tab) { // creating cases for fragment when (tab.position) { 0 -> fragment = JavaFragment() 1 -> fragment = AndroidFragment() 2 -> fragment = KotlinFragment() 3 -> fragment = PhpFragment() 4 -> fragment = PythonFragment() } val fm = supportFragmentManager val ft = fm.beginTransaction() ft.replace(R.id.frameLayout, fragment) ft.setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN) ft.commit() } override fun onTabUnselected(tab: TabLayout.Tab) { } override fun onTabReselected(tab: TabLayout.Tab) { } }) } }
Create the FrameLayout for each fragment as New -> Fragment -> Fragment (Blank).
fragment_java.xml
< FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlintablayoutwithframelayout.JavaFragment"> < !-- TODO: Update blank fragment layout --> < TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:textSize="20sp" android:text="@string/java_fragment" /> < Button android:id="@+id/androidButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center|bottom" android:text="Click Me"/> < /FrameLayout>
MainActivity.kt
package example.javatpoint.com.kotlintablayoutwithframelayout import android.os.Bundle import android.support.v4.app.Fragment import android.view.LayoutInflater import android.view.View import android.view.ViewGroup import android.widget.Toast import kotlinx.android.synthetic.main.fragment_android.view.* class JavaFragment : Fragment() { override fun onCreateView(inflater: LayoutInflater?, container: ViewGroup?, savedInstanceState: Bundle?): View? { // Inflate the layout for this fragment val view: View = inflater!!.inflate(R.layout.fragment_java, container, false) view.androidButton!!.setOnClickListener(View.OnClickListener { Toast.makeText(context,"java fragment", Toast.LENGTH_SHORT).show() }) return view } }// Required empty public constructor
fragment_android.xml
< FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlintablayoutwithframelayout.AndroidFragment"> < !-- TODO: Update blank fragment layout --> < TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:textSize="20sp" android:text="@string/android_fragment" /> < Button android:id="@+id/androidButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center|bottom" android:text="Click Me"/> < /FrameLayout>
AndroidFragment.kt
package example.javatpoint.com.kotlintablayoutwithframelayout import android.os.Bundle import android.support.v4.app.Fragment import android.view.LayoutInflater import android.view.View import android.view.ViewGroup import android.widget.Toast import kotlinx.android.synthetic.main.fragment_android.view.* class AndroidFragment : Fragment() { override fun onCreateView(inflater: LayoutInflater?, container: ViewGroup?, savedInstanceState: Bundle?): View? { // Inflate the layout for this fragment val view: View = inflater!!.inflate(R.layout.fragment_android, container, false) view.androidButton!!.setOnClickListener(View.OnClickListener { Toast.makeText(context,"android fragment",Toast.LENGTH_SHORT).show() }) return view } }// Required empty public constructor
fragment_kotlin.xml
< FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlintablayoutwithframelayout.KotlinFragment"> < !-- TODO: Update blank fragment layout --> < TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:textSize="20sp" android:text="@string/kotlin_fragment" /> < Button android:id="@+id/androidButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center|bottom" android:text="Click Me"/> < /FrameLayout>
KotlinFragment.kt
package example.javatpoint.com.kotlintablayoutwithframelayout import android.os.Bundle import android.support.v4.app.Fragment import android.view.LayoutInflater import android.view.View import android.view.ViewGroup import android.widget.Toast import kotlinx.android.synthetic.main.fragment_android.view.* class KotlinFragment : Fragment() { override fun onCreateView(inflater: LayoutInflater?, container: ViewGroup?, savedInstanceState: Bundle?): View? { // Inflate the layout for this fragment val view: View = inflater!!.inflate(R.layout.fragment_kotlin, container, false) view.androidButton!!.setOnClickListener(View.OnClickListener { Toast.makeText(context,"kotlin fragment", Toast.LENGTH_SHORT).show() }) return view } }// Required empty public constructor
fragment_php.xml
< FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlintablayoutwithframelayout.PhpFragment"> < !-- TODO: Update blank fragment layout --> < TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:textSize="20sp" android:text="@string/php_fragment" /> < Button android:id="@+id/androidButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center|bottom" android:text="Click Me"/> < /FrameLayout>
PhpFragment.kt
package example.javatpoint.com.kotlintablayoutwithframelayout import android.os.Bundle import android.support.v4.app.Fragment import android.view.LayoutInflater import android.view.View import android.view.ViewGroup import android.widget.Toast import kotlinx.android.synthetic.main.fragment_android.view.* class PhpFragment : Fragment() { override fun onCreateView(inflater: LayoutInflater?, container: ViewGroup?, savedInstanceState: Bundle?): View? { // Inflate the layout for this fragment val view: View = inflater!!.inflate(R.layout.fragment_php, container, false) view.androidButton!!.setOnClickListener(View.OnClickListener { Toast.makeText(context,"php fragment", Toast.LENGTH_SHORT).show() }) return view } }// Required empty public constructor
fragment_python.xml
< FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlintablayoutwithframelayout.PythonFragment"> < !-- TODO: Update blank fragment layout --> < TextView android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:textSize="20sp" android:text="@string/python_fragment" /> < Button android:id="@+id/androidButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center|bottom" android:text="Click Me"/> < /FrameLayout>
PythonFragment.kt
package example.javatpoint.com.kotlintablayoutwithframelayout import android.os.Bundle import android.support.v4.app.Fragment import android.view.LayoutInflater import android.view.View import android.view.ViewGroup import android.widget.Toast import kotlinx.android.synthetic.main.fragment_android.view.* class PythonFragment : Fragment() { override fun onCreateView(inflater: LayoutInflater?, container: ViewGroup?, savedInstanceState: Bundle?): View? { // Inflate the layout for this fragment val view: View = inflater!!.inflate(R.layout.fragment_python, container, false) view.androidButton!!.setOnClickListener(View.OnClickListener { Toast.makeText(context,"python fragment", Toast.LENGTH_SHORT).show() }) return view } }// Required empty public constructor
Output:
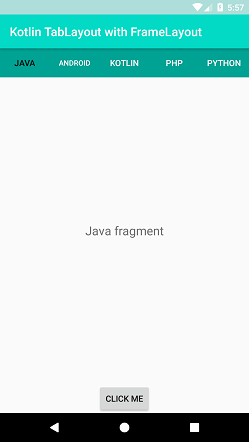
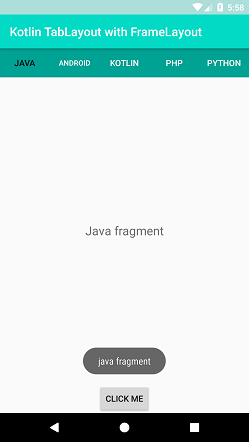
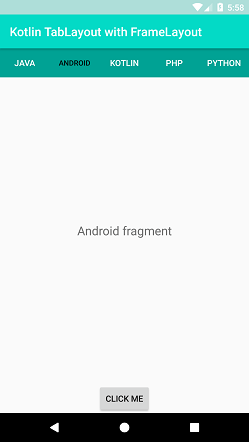
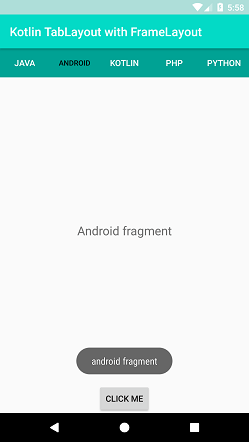
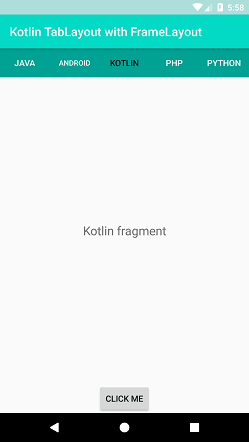
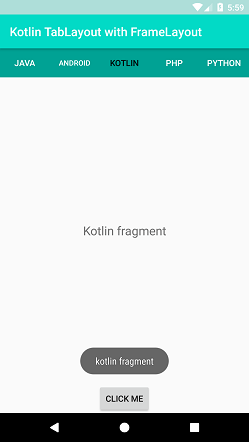