Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Android Media Player
MediaPlayer is a class that is used to control the playback of audio/video files and streams.
The android.media.MediaPlayer class is used to control the audio or video files. It accesses the built-in media player services such as playing audio, video, etc. To use the MediaPlayer class, we have to call the create the instance of it by calling the create() method of this class.
Methods of MediaPlayer class
There are several methods of MediaPlayer class available. Some of them are as follows:
Method | Description |
public void setDataSource(String path) | It sets the data source (file path or http url) to use. |
public void prepare() | It prepares the player for playback synchronously. |
public void start() | It starts or resumes the playback. |
public void stop() | It stops the playback. |
public void pause() | It pauses the playback. |
public boolean isPlaying() | It checks if the media player is playing. |
public void seekTo(int millis) | It seeks to specified time in milliseconds. |
public void setLooping(boolean looping) | It sets the player for looping or non-looping. |
public boolean isLooping() | It checks if the player is looping or non-looping. |
public void selectTrack(int index) | It selects a track for the specified index. |
public int getCurrentPosition() | It returns the current playback position. |
public int getDuration() | It returns duration of the file. |
public void setVolume(float leftVolume,float rightVolume) | It sets the volume on this player. |
Example of Android MediaPlayer with SeekBar
In this example, we will create a Media Player with playback control functionality such as play, pause, and stop. We also integrate the SeekBar to show the progress level of the media player.
activity_main.xml
In the activity_main.xml layout file, we add the Buttons to control media playback, TextView to display the time duration of song and SeekBar to show the progress level of the media file.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinmediaplayer.MainActivity"> < Button android:id="@+id/pauseBtn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:enabled="false" android:text="Pause" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@+id/playBtn" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> < Button android:id="@+id/playBtn" android:layout_width="88dp" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:text="Play" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@+id/stopBtn" app:layout_constraintStart_toEndOf="@+id/pauseBtn" app:layout_constraintTop_toTopOf="parent" /> < Button android:id="@+id/stopBtn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="24dp" android:layout_marginRight="24dp" android:layout_marginTop="8dp" android:enabled="false" android:text="Stop" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintTop_toTopOf="parent" /> < RelativeLayout android:layout_width="368dp" android:layout_height="wrap_content" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="76dp" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="1.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent"> < TextView android:id="@+id/tv_pass" android:layout_width="wrap_content" android:layout_height="wrap_content" /> < TextView android:id="@+id/tv_due" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentEnd="true" android:layout_alignParentRight="true" /> < SeekBar android:id="@+id/seek_bar" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/tv_pass" android:saveEnabled="false" /> < /RelativeLayout> < /android.support.constraint.ConstraintLayout> < ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinmediaplayer.MainActivity"> < Button android:id="@+id/pauseBtn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:enabled="false" android:text="Pause" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@+id/playBtn" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> < Button android:id="@+id/playBtn" android:layout_width="88dp" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:text="Play" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@+id/stopBtn" app:layout_constraintStart_toEndOf="@+id/pauseBtn" app:layout_constraintTop_toTopOf="parent" /> < Button android:id="@+id/stopBtn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="24dp" android:layout_marginRight="24dp" android:layout_marginTop="8dp" android:enabled="false" android:text="Stop" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintTop_toTopOf="parent" /> < RelativeLayout android:layout_width="368dp" android:layout_height="wrap_content" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="76dp" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="1.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent"> < TextView android:id="@+id/tv_pass" android:layout_width="wrap_content" android:layout_height="wrap_content" /> < TextView android:id="@+id/tv_due" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentEnd="true" android:layout_alignParentRight="true" /> < SeekBar android:id="@+id/seek_bar" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/tv_pass" android:saveEnabled="false" /> < /RelativeLayout> < /android.support.constraint.ConstraintLayout>
MianActivity.kt
The instance of MediaPlayer class is created using the MediaPlayer.create() method. In this example, we are reading the media file from the raw directory. The MediaPlayer.start() method starts playing media file, MediaPlayer.pause() method pause media and Media.stop() method stops playing media file.
package example.javatpoint.com.kotlinmediaplayer import android.media.MediaPlayer import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.widget.Toast import kotlinx.android.synthetic.main.activity_main.* import android.os.Handler import android.widget.SeekBar class MainActivity : AppCompatActivity() { private lateinit var mediaPlayer: MediaPlayer private lateinit var runnable:Runnable private var handler: Handler = Handler() private var pause:Boolean = false override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // Start the media player playBtn.setOnClickListener{ if(pause){ mediaPlayer.seekTo(mediaPlayer.currentPosition) mediaPlayer.start() pause = false Toast.makeText(this,"media playing",Toast.LENGTH_SHORT).show() }else{ mediaPlayer = MediaPlayer.create(applicationContext,R.raw.school_bell) mediaPlayer.start() Toast.makeText(this,"media playing",Toast.LENGTH_SHORT).show() } initializeSeekBar() playBtn.isEnabled = false pauseBtn.isEnabled = true stopBtn.isEnabled = true mediaPlayer.setOnCompletionListener { playBtn.isEnabled = true pauseBtn.isEnabled = false stopBtn.isEnabled = false Toast.makeText(this,"end",Toast.LENGTH_SHORT).show() } } // Pause the media player pauseBtn.setOnClickListener { if(mediaPlayer.isPlaying){ mediaPlayer.pause() pause = true playBtn.isEnabled = true pauseBtn.isEnabled = false stopBtn.isEnabled = true Toast.makeText(this,"media pause",Toast.LENGTH_SHORT).show() } } // Stop the media player stopBtn.setOnClickListener{ if(mediaPlayer.isPlaying || pause.equals(true)){ pause = false seek_bar.setProgress(0) mediaPlayer.stop() mediaPlayer.reset() mediaPlayer.release() handler.removeCallbacks(runnable) playBtn.isEnabled = true pauseBtn.isEnabled = false stopBtn.isEnabled = false tv_pass.text = "" tv_due.text = "" Toast.makeText(this,"media stop",Toast.LENGTH_SHORT).show() } } // Seek bar change listener seek_bar.setOnSeekBarChangeListener(object : SeekBar.OnSeekBarChangeListener { override fun onProgressChanged(seekBar: SeekBar, i: Int, b: Boolean) { if (b) { mediaPlayer.seekTo(i * 1000) } } override fun onStartTrackingTouch(seekBar: SeekBar) { } override fun onStopTrackingTouch(seekBar: SeekBar) { } }) } // Method to initialize seek bar and audio stats private fun initializeSeekBar() { seek_bar.max = mediaPlayer.seconds runnable = Runnable { seek_bar.progress = mediaPlayer.currentSeconds tv_pass.text = "${mediaPlayer.currentSeconds} sec" val diff = mediaPlayer.seconds - mediaPlayer.currentSeconds tv_due.text = "$diff sec" handler.postDelayed(runnable, 1000) } handler.postDelayed(runnable, 1000) } } // Creating an extension property to get the media player time duration in seconds val MediaPlayer.seconds:Int get() { return this.duration / 1000 } // Creating an extension property to get media player current position in seconds val MediaPlayer.currentSeconds:Int get() { return this.currentPosition/1000 } package example.javatpoint.com.kotlinmediaplayer import android.media.MediaPlayer import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.widget.Toast import kotlinx.android.synthetic.main.activity_main.* import android.os.Handler import android.widget.SeekBar class MainActivity : AppCompatActivity() { private lateinit var mediaPlayer: MediaPlayer private lateinit var runnable:Runnable private var handler: Handler = Handler() private var pause:Boolean = false override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // Start the media player playBtn.setOnClickListener{ if(pause){ mediaPlayer.seekTo(mediaPlayer.currentPosition) mediaPlayer.start() pause = false Toast.makeText(this,"media playing",Toast.LENGTH_SHORT).show() }else{ mediaPlayer = MediaPlayer.create(applicationContext,R.raw.school_bell) mediaPlayer.start() Toast.makeText(this,"media playing",Toast.LENGTH_SHORT).show() } initializeSeekBar() playBtn.isEnabled = false pauseBtn.isEnabled = true stopBtn.isEnabled = true mediaPlayer.setOnCompletionListener { playBtn.isEnabled = true pauseBtn.isEnabled = false stopBtn.isEnabled = false Toast.makeText(this,"end",Toast.LENGTH_SHORT).show() } } // Pause the media player pauseBtn.setOnClickListener { if(mediaPlayer.isPlaying){ mediaPlayer.pause() pause = true playBtn.isEnabled = true pauseBtn.isEnabled = false stopBtn.isEnabled = true Toast.makeText(this,"media pause",Toast.LENGTH_SHORT).show() } } // Stop the media player stopBtn.setOnClickListener{ if(mediaPlayer.isPlaying || pause.equals(true)){ pause = false seek_bar.setProgress(0) mediaPlayer.stop() mediaPlayer.reset() mediaPlayer.release() handler.removeCallbacks(runnable) playBtn.isEnabled = true pauseBtn.isEnabled = false stopBtn.isEnabled = false tv_pass.text = "" tv_due.text = "" Toast.makeText(this,"media stop",Toast.LENGTH_SHORT).show() } } // Seek bar change listener seek_bar.setOnSeekBarChangeListener(object : SeekBar.OnSeekBarChangeListener { override fun onProgressChanged(seekBar: SeekBar, i: Int, b: Boolean) { if (b) { mediaPlayer.seekTo(i * 1000) } } override fun onStartTrackingTouch(seekBar: SeekBar) { } override fun onStopTrackingTouch(seekBar: SeekBar) { } }) } // Method to initialize seek bar and audio stats private fun initializeSeekBar() { seek_bar.max = mediaPlayer.seconds runnable = Runnable { seek_bar.progress = mediaPlayer.currentSeconds tv_pass.text = "${mediaPlayer.currentSeconds} sec" val diff = mediaPlayer.seconds - mediaPlayer.currentSeconds tv_due.text = "$diff sec" handler.postDelayed(runnable, 1000) } handler.postDelayed(runnable, 1000) } } // Creating an extension property to get the media player time duration in seconds val MediaPlayer.seconds:Int get() { return this.duration / 1000 } // Creating an extension property to get media player current position in seconds val MediaPlayer.currentSeconds:Int get() { return this.currentPosition/1000 }
Output:
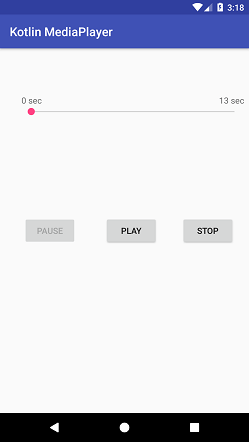
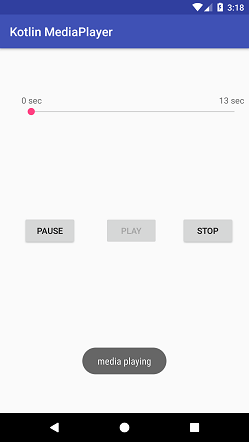
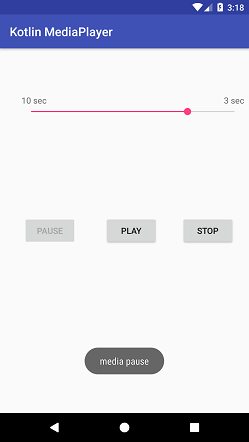