Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Android SeekBar
Android SeekBar is a kind of ProgressBar with a draggable thumb. The end user can drag the thumb left and right to move the progress of the song, file download etc.
The SeekBar.OnSeekBarChangeListener interface provides methods to perform even handling for seek bar.
We can create two types of SeekBar in Android
- SeekBar with smooth progress.
- SeekBar with discrete progress point.
The discrete progress point of SeekBar is created using attribute style="@style/Widget.AppCompat.SeekBar.Discrete" and specify the maximum point using attribute max.
Kotlin Android SeekBar Example
In this example, we will create the SeekBar with smooth progress and SeekBar with discrete progress point.
activity_main.xml
Add the SeekBar in the activity_main.xml layout.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinseekbar.MainActivity"> < SeekBar android:id="@+id/seekbar_Default" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.174" /> < SeekBar android:id="@+id/seekbar_Discrete" style="@style/Widget.AppCompat.SeekBar.Discrete" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:max="10" android:progress="0" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="1.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.333" /> < /android.support.constraint.ConstraintLayout>
MainActivity.kt
In this class, we call the SeekBar.OnSeekBarChangeListener interface and override its methods.
package example.javatpoint.com.kotlinseekbar import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.widget.SeekBar import android.widget.Toast class MainActivity : AppCompatActivity() { var seekBarNormal: SeekBar? = null var seekBarDiscrete: SeekBar? = null override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) seekBarNormal= findViewById(R.id.seekbar_Default) seekBarDiscrete = findViewById(R.id.seekbar_Discrete) seekBarNormal?.setOnSeekBarChangeListener(object : SeekBar.OnSeekBarChangeListener { override fun onProgressChanged(seekBar: SeekBar, progress: Int, fromUser: Boolean) { Toast.makeText(applicationContext, "seekbar progress: $progress", Toast.LENGTH_SHORT).show() } override fun onStartTrackingTouch(seekBar: SeekBar) { Toast.makeText(applicationContext, "seekbar touch started!", Toast.LENGTH_SHORT).show() } override fun onStopTrackingTouch(seekBar: SeekBar) { Toast.makeText(applicationContext, "seekbar touch stopped!", Toast.LENGTH_SHORT).show() } }) seekBarDiscrete?.setOnSeekBarChangeListener(object :SeekBar.OnSeekBarChangeListener{ override fun onProgressChanged(seekBar: SeekBar?, progress: Int, fromUser: Boolean) { // TODO("not implemented") //To change body of created functions use File | Settings | File Templates. Toast.makeText(applicationContext, "discrete seekbar progress: $progress", Toast.LENGTH_SHORT).show() } override fun onStartTrackingTouch(seekBar: SeekBar?) { // TODO("not implemented") //To change body of created functions use File | Settings | File Templates. Toast.makeText(applicationContext, "discrete seekbar touch started!", Toast.LENGTH_SHORT).show() } override fun onStopTrackingTouch(seekBar: SeekBar?) { // TODO("not implemented") //To change body of created functions use File | Settings | File Templates. Toast.makeText(applicationContext, "discrete seekbar touch stopped", Toast.LENGTH_SHORT).show() } }) } }
Output:
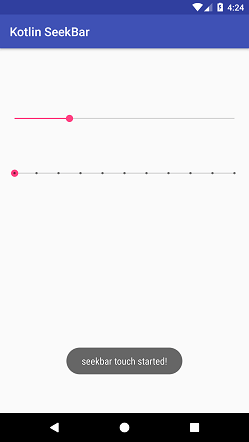
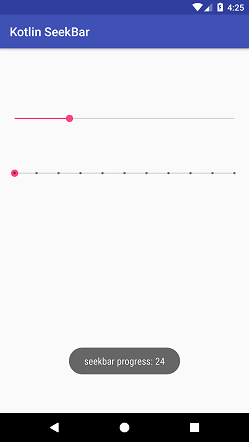
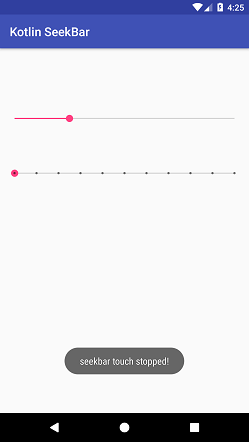
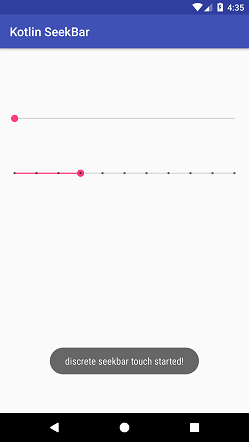
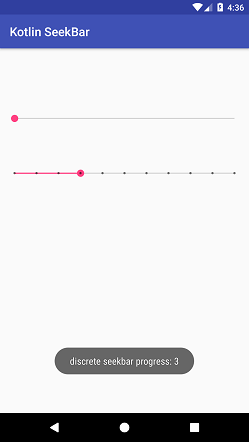
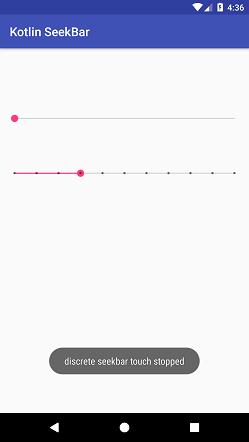