Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Android Explicit Intent
Android Intent is a messaging object used to request another app component to perform an action. Intent facilitates users to communicate with app component through several ways such as starting an activity, starting a service, delivering a broadcast receiver, etc.
Android intents are mainly used to:
- Start the service
- Launch an activity
- Display a web page
- Display a list of contacts
- Broadcast a message
- Dial a phone call etc.
Types of Android Intents
There are two types of intent in Android:
Explicit Intent: This intent satisfies the request within the application component. It takes the fully qualified class name of activities or services that we want to start.
intent = Intent(applicationContext, SecondActivity::class.java) startActivity(intent)
Implicit Intent: This intent does not specify the component name. It invokes the component of another app to handle it.
intent = Intent(Intent.ACTION_VIEW) intent.setData(Uri.parse("https://www.javatpoint.com/")) startActivity(intent) intent= Intent(Intent.ACTION_VIEW, Uri.parse("https://www.javatpoint.com/")) startActivity(intent)
Kotlin Android Explicit Intent Example
In this example, we will call the other activity class from another activity class using explicit intent. Using intent, we will send the data from the first activity class to second activity class. Second activity class gets this data and displays them in toast message.
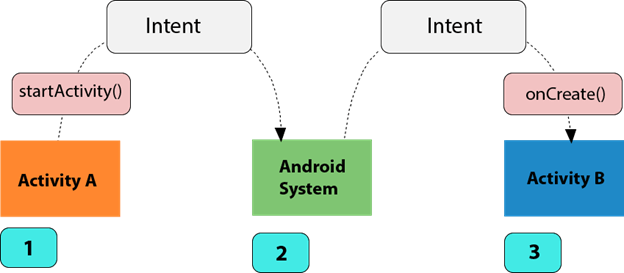
activity_main.xml
Add the following code in the activity_main.xml.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinexplicitintent.MainActivity"> < TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="8dp" android:text="First Activity" android:textSize="18sp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintHorizontal_bias="0.501" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.172" /> < Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:text="Click" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView" app:layout_constraintVertical_bias="0.77" /> < /android.support.constraint.ConstraintLayout>
MainActivity.kt
Add the following code in the MainActivity.kt class. In this class, we are creating an instance of Intent class and calling the component activity class SecondActivity.kt. The putExtra(key, value) method of Intent class send the data to the SecondActivity.kt class. The startActivity() method starts the Intent.
package example.javatpoint.com.kotlinexplicitintent import android.content.Intent import android.support.v7.app.AppCompatActivity import android.os.Bundle import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { val id:Int = 10 val language:String = "kotlin" override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) button.setOnClickListener() { intent = Intent(this, SecondActivity::class.java) intent.putExtra("id_value", id) intent.putExtra("language_value", language) startActivity(intent) } } }
Create another activity class named as SecondActivity.
second_activity.xml
In the second_activity.xml file add the following code.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinexplicitintent.SecondActivity"> < TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:text="SecondActivity" android:textSize="18sp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.107" /> < Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:text="back" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.774" /> < /android.support.constraint.ConstraintLayout>
SecondActivity.kt
Add the following code in the SecondActivity.kt class. In this class, we are receiving the intent data using creating the instance on Bundle class using intent.extras and displaying the data in toast message. By clicking on the button, we are invoking Intent to call MainActivity.kt class.
package example.javatpoint.com.kotlinexplicitintent import android.content.Intent import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.widget.Toast import kotlinx.android.synthetic.main.activity_second.* class SecondActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_second) val bundle:Bundle = intent.extras val id = bundle.get("id_value") val language = bundle.get("language_value") Toast.makeText(applicationContext,id.toString()+" "+language,Toast.LENGTH_LONG).show() button2.setOnClickListener(){ intent = Intent(this,MainActivity::class.java) startActivity(intent) } } }
Output:
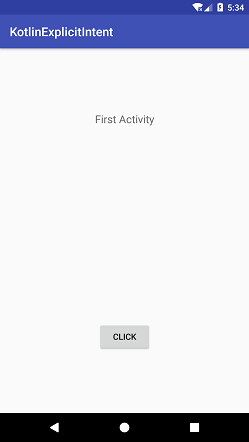
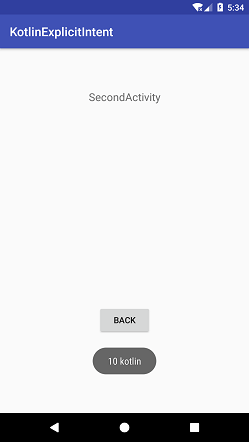