Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Android Video Player
The android.widget.VideoView class is used to play video player in an application. The android.widget.MediaController class provides the playback controls of video player such as play, pause, backward, forward, etc. to control the video player.
Using VideoView and MediaController class we can create a video player.
Method of VideoView class
The android.widget.VideoView class provides several methods to play and control the video player. The commonly used methods of VideoView class are as follows:
Method | Description |
public void setMediaController(MediaController controller) | It sets the media controller to the video view. |
public void setVideoURI (Uri uri) | It sets the URI of the video file. |
public void start() | It starts the video view. |
public void stopPlayback() | It stops the playback. |
public void pause() | It pauses the playback. |
public void suspend() | It suspends the playback. |
public void resume() | It resumes the playback. |
public void seekTo(int millis) | It seeks to specified time in milliseconds. |
Kotlin Android Video Player Example
In this example, we play the video inside the VideoView.
activity_main.xml
Add the following design code in an activity_main.xml file. In this file, we place the VideoView inside the FrameLayout.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinvideoplayer.MainActivity"> < FrameLayout android:layout_width="match_parent" android:layout_height="wrap_content"> < VideoView android:id="@+id/videoView" android:layout_width="wrap_content" android:layout_height="308dp" android:layout_marginBottom="0dp" android:layout_marginEnd="0dp" android:layout_marginStart="0dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="1.0" /> < /FrameLayout> < /android.support.constraint.ConstraintLayout> < ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinvideoplayer.MainActivity"> < FrameLayout android:layout_width="match_parent" android:layout_height="wrap_content"> < VideoView android:id="@+id/videoView" android:layout_width="wrap_content" android:layout_height="308dp" android:layout_marginBottom="0dp" android:layout_marginEnd="0dp" android:layout_marginStart="0dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="1.0" /> < /FrameLayout> < /android.support.constraint.ConstraintLayout>
MainActivity.kt
Add the following code in MainActivity.kt class. In this class, we are reading the video file video.mp4 from the sdcard/Movies directory. The path of a video file is placed by creating the instance of Uri class and passed it to VideoView.setVideoURI(Uri). To play the video file call the start() method of VideoView.
package example.javatpoint.com.kotlinvideoplayer import android.net.Uri import android.net.Uri.* import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.os.Environment import android.widget.MediaController import android.widget.VideoView class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val videoView = findViewById< VideoView>(R.id.videoView) //Creating MediaController val mediaController = MediaController(this) mediaController.setAnchorView(videoView) //specify the location of media file val uri:Uri = parse(Environment.getExternalStorageDirectory().getPath() + "/Movies/video.mp4") //Setting MediaController and URI, then starting the videoView videoView.setMediaController(mediaController) videoView.setVideoURI(uri) videoView.requestFocus() videoView.start() } } package example.javatpoint.com.kotlinvideoplayer import android.net.Uri import android.net.Uri.* import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.os.Environment import android.widget.MediaController import android.widget.VideoView class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val videoView = findViewById< VideoView>(R.id.videoView) //Creating MediaController val mediaController = MediaController(this) mediaController.setAnchorView(videoView) //specify the location of media file val uri:Uri = parse(Environment.getExternalStorageDirectory().getPath() + "/Movies/video.mp4") //Setting MediaController and URI, then starting the videoView videoView.setMediaController(mediaController) videoView.setVideoURI(uri) videoView.requestFocus() videoView.start() } }
AndroidManifest.xml
Add the following uses-permission in AndroidManifest.xml file.
< uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/> < uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/> < uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/> < uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
Output:
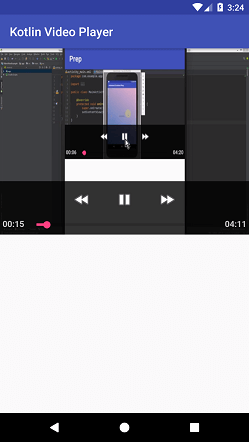