Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Android Web Service
In this tutorial, we will create the basic user registration and log-in module using Volley library and JSON. Volley is an HTTP Library which provides the facilities for network connectivity for our app.
The web API of registration and login is created using PHP with MySQL. Before implementing the client side Android application code, first look at the server side API code at https://www.mcatutorials.com/android-volley-library-registration-login-logout.
In Android application, we will create the three activity class for user registration, user log-in and display the user detail in main activity (as a profile).
Create an activity_main.xml in layout and add the following code. This activity is used to display the detail of the user profile.
activity_main.xml
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinvolleyregistrationloginsystem.MainActivity"> < LinearLayout android:layout_width="match_parent" android:layout_height="fill_parent" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:orientation="vertical" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.0"> < TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:gravity="center" android:text="Welcome to Profile" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" /> < TableLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="50dp"> < TableRow> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="Id" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" /> < TextView android:id="@+id/textViewId" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="id" android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" /> < /TableRow> < TableRow> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="Username" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" /> < TextView android:id="@+id/textViewUsername" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="username" android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" /> < /TableRow> < TableRow> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="Email" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" /> < TextView android:id="@+id/textViewEmail" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="useremail" android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" /> < /TableRow> < TableRow> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="Gender" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" /> < TextView android:id="@+id/textViewGender" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="gender" android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" /> < /TableRow> < /TableLayout> < Button android:id="@+id/buttonLogout" android:layout_width="210dp" android:layout_height="50dp" android:layout_marginTop="150dp" android:layout_marginLeft="75dp" android:text="Logout" /> < /LinearLayout> < /android.support.constraint.ConstraintLayout> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinvolleyregistrationloginsystem.MainActivity"> < LinearLayout android:layout_width="match_parent" android:layout_height="fill_parent" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:orientation="vertical" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.0"> < TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:gravity="center" android:text="Welcome to Profile" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" /> < TableLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="50dp"> < TableRow> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="Id" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" /> < TextView android:id="@+id/textViewId" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="id" android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" /> < /TableRow> < TableRow> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="Username" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" /> < TextView android:id="@+id/textViewUsername" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="username" android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" /> < /TableRow> < TableRow> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="Email" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" /> < TextView android:id="@+id/textViewEmail" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="useremail" android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" /> < /TableRow> < TableRow> < TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="Gender" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" /> < TextView android:id="@+id/textViewGender" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:text="gender" android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" /> < /TableRow> < /TableLayout> < Button android:id="@+id/buttonLogout" android:layout_width="210dp" android:layout_height="50dp" android:layout_marginTop="150dp" android:layout_marginLeft="75dp" android:text="Logout" /> < /LinearLayout> < /android.support.constraint.ConstraintLayout>
activity_login.xml
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinvolleyregistrationloginsystem.LoginActivity"> < TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="16dp" android:gravity="center" android:text="Login" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="1.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> < EditText android:id="@+id/etUserName" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:ems="10" android:inputType="textPersonName" android:hint="user name" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.144" /> < EditText android:id="@+id/etUserPassword" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:hint="password" android:ems="10" android:inputType="textPassword" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/etUserName" /> < Button android:id="@+id/btnLogin" android:layout_width="210dp" android:layout_height="50dp" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:text="Login" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/etUserName" app:layout_constraintVertical_bias="0.754" /> < TextView android:id="@+id/tvRegister" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:gravity="center" android:text="Create New Account\n Register Here" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/btnLogin" app:layout_constraintVertical_bias="0.405" /> < ProgressBar android:id="@+id/progressBar" android:visibility="gone" style="?android:attr/progressBarStyle" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.456" /> < /android.support.constraint.ConstraintLayout> < ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinvolleyregistrationloginsystem.LoginActivity"> < TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="16dp" android:gravity="center" android:text="Login" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="1.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> < EditText android:id="@+id/etUserName" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:ems="10" android:inputType="textPersonName" android:hint="user name" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.144" /> < EditText android:id="@+id/etUserPassword" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:hint="password" android:ems="10" android:inputType="textPassword" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/etUserName" /> < Button android:id="@+id/btnLogin" android:layout_width="210dp" android:layout_height="50dp" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:text="Login" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/etUserName" app:layout_constraintVertical_bias="0.754" /> < TextView android:id="@+id/tvRegister" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:gravity="center" android:text="Create New Account\n Register Here" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/btnLogin" app:layout_constraintVertical_bias="0.405" /> < ProgressBar android:id="@+id/progressBar" android:visibility="gone" style="?android:attr/progressBarStyle" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.456" /> < /android.support.constraint.ConstraintLayout>
Create an activity_register.xml file in the layout directory with following code. This activity is used for user registration UI.
activity_register.xml
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinvolleyregistrationloginsystem.RegisterActivity"> < LinearLayout android:layout_width="match_parent" android:layout_height="519dp" android:layout_centerVertical="true" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:orientation="vertical" android:padding="10dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.0"> < TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Register" android:gravity="center" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" /> < EditText android:id="@+id/editTextUsername" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="20dp" android:hint="Username" android:inputType="text" /> < EditText android:id="@+id/editTextEmail" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="8dp" android:hint="Email" android:inputType="textEmailAddress" /> < EditText android:id="@+id/editTextPassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="8dp" android:fontFamily="sans-serif" android:hint="Password" android:inputType="textPassword" /> < RadioGroup android:id="@+id/radioGender" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="8dp" android:orientation="horizontal"> < RadioButton android:id="@+id/radioButtonMale" android:layout_width="wrap_content" android:layout_height="wrap_content" android:checked="true" android:text="Male" /> < RadioButton android:id="@+id/radioButtonFemale" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Female" /> < /RadioGroup> < Button android:id="@+id/buttonRegister" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="90dp" android:text="Register" /> < TextView android:id="@+id/textViewLogin" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="8dp" android:text="Already Registered?\nLogin Here" android:textAlignment="center" android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" /> < /LinearLayout> < ProgressBar android:visibility="gone" android:id="@+id/progressBar" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" /> < /android.support.constraint.ConstraintLayout> < ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinvolleyregistrationloginsystem.RegisterActivity"> < LinearLayout android:layout_width="match_parent" android:layout_height="519dp" android:layout_centerVertical="true" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:orientation="vertical" android:padding="10dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.0"> < TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Register" android:gravity="center" android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" /> < EditText android:id="@+id/editTextUsername" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="20dp" android:hint="Username" android:inputType="text" /> < EditText android:id="@+id/editTextEmail" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="8dp" android:hint="Email" android:inputType="textEmailAddress" /> < EditText android:id="@+id/editTextPassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="8dp" android:fontFamily="sans-serif" android:hint="Password" android:inputType="textPassword" /> < RadioGroup android:id="@+id/radioGender" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="8dp" android:orientation="horizontal"> < RadioButton android:id="@+id/radioButtonMale" android:layout_width="wrap_content" android:layout_height="wrap_content" android:checked="true" android:text="Male" /> < RadioButton android:id="@+id/radioButtonFemale" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Female" /> < /RadioGroup> < Button android:id="@+id/buttonRegister" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="90dp" android:text="Register" /> < TextView android:id="@+id/textViewLogin" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginTop="8dp" android:text="Already Registered?\nLogin Here" android:textAlignment="center" android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" /> < /LinearLayout> < ProgressBar android:visibility="gone" android:id="@+id/progressBar" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" /> < /android.support.constraint.ConstraintLayout>
Add the volley library dependency in build.gradle file.
build.gradle
dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version" implementation 'com.android.support:appcompat-v7:26.1.0' implementation 'com.android.support.constraint:constraint-layout:1.1.3' testImplementation 'junit:junit:4.12' implementation 'com.android.volley:volley:1.0.0' } dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version" implementation 'com.android.support:appcompat-v7:26.1.0' implementation 'com.android.support.constraint:constraint-layout:1.1.3' testImplementation 'junit:junit:4.12' implementation 'com.android.volley:volley:1.0.0' }
Create a data model class named as User.kt with the following code.
User.kt
package example.javatpoint.com.kotlinvolleyregistrationloginsystem class User(var id: Int, var name: String?, var email: String?, var gender: String?) package example.javatpoint.com.kotlinvolleyregistrationloginsystem class User(var id: Int, var name: String?, var email: String?, var gender: String?)
We need to define our URL that calls the API of server-side.
Create an URLs.kt class and define the URL.
URLs.kt
package example.javatpoint.com.kotlinvolleyregistrationloginsystem object URLs { private val ROOT_URL = "http://192.168.1.35/androidphpmysql/registrationapi.php?apicall=" val URL_REGISTER = ROOT_URL + "signup" val URL_LOGIN = ROOT_URL + "login" } package example.javatpoint.com.kotlinvolleyregistrationloginsystem object URLs { private val ROOT_URL = "http://192.168.1.35/androidphpmysql/registrationapi.php?apicall=" val URL_REGISTER = ROOT_URL + "signup" val URL_LOGIN = ROOT_URL + "login" }
VolleySingleton.kt
package example.javatpoint.com.kotlinvolleyregistrationloginsystem import android.content.Context import com.android.volley.Request import com.android.volley.RequestQueue import com.android.volley.toolbox.Volley class VolleySingleton private constructor(context: Context) { private var mRequestQueue: RequestQueue // applicationContext is key, it keeps you from leaking the // Activity or BroadcastReceiver if someone passes one in. val requestQueue: RequestQueue get() { if (mRequestQueue == null) { mRequestQueue = Volley.newRequestQueue(mCtx?.applicationContext) } return mRequestQueue } init { mCtx = context mRequestQueue = requestQueue } fun < T> addToRequestQueue(req: Request) { requestQueue.add(req) } companion object { private var mInstance: VolleySingleton? = null private var mCtx: Context? = null @Synchronized fun getInstance(context: Context): VolleySingleton { if (mInstance == null) { mInstance = VolleySingleton(context) } return mInstance as VolleySingleton } } } package example.javatpoint.com.kotlinvolleyregistrationloginsystem import android.content.Context import com.android.volley.Request import com.android.volley.RequestQueue import com.android.volley.toolbox.Volley class VolleySingleton private constructor(context: Context) { private var mRequestQueue: RequestQueue // applicationContext is key, it keeps you from leaking the // Activity or BroadcastReceiver if someone passes one in. val requestQueue: RequestQueue get() { if (mRequestQueue == null) { mRequestQueue = Volley.newRequestQueue(mCtx?.applicationContext) } return mRequestQueue } init { mCtx = context mRequestQueue = requestQueue } fun < T> addToRequestQueue(req: Request ) { requestQueue.add(req) } companion object { private var mInstance: VolleySingleton? = null private var mCtx: Context? = null @Synchronized fun getInstance(context: Context): VolleySingleton { if (mInstance == null) { mInstance = VolleySingleton(context) } return mInstance as VolleySingleton } } }
Create a class named as SharedPreferences.kt. In this class, we use the SharedPreferences class to store the user detail. The SharedPreferences class contains four methods with the following functionalities:
- userLogin(): This function is used to store the user information in SharedPreferences after log-in.
- isLoggedIn: This method checks whether the user is already log-in or not.
- user: User get(): This method gets the user information if log-in.
- logout(): This function clears the SharedPreferences data and makes user log-out.
SharedPrefManager.kt
package example.javatpoint.com.kotlinvolleyregistrationloginsystem import android.content.Context import android.content.Intent class SharedPrefManager private constructor(context: Context) { //this method will checker whether user is already logged in or not val isLoggedIn: Boolean get() { val sharedPreferences = ctx?.getSharedPreferences(SHARED_PREF_NAME, Context.MODE_PRIVATE) return sharedPreferences?.getString(KEY_USERNAME, null) != null } //this method will give the logged in user val user: User get() { val sharedPreferences = ctx?.getSharedPreferences(SHARED_PREF_NAME, Context.MODE_PRIVATE) return User( sharedPreferences!!.getInt(KEY_ID, -1), sharedPreferences.getString(KEY_USERNAME, null), sharedPreferences.getString(KEY_EMAIL, null), sharedPreferences.getString(KEY_GENDER, null) ) } init { ctx = context } //this method will store the user data in shared preferences fun userLogin(user: User) { val sharedPreferences = ctx?.getSharedPreferences(SHARED_PREF_NAME, Context.MODE_PRIVATE) val editor = sharedPreferences?.edit() editor?.putInt(KEY_ID, user.id) editor?.putString(KEY_USERNAME, user.name) editor?.putString(KEY_EMAIL, user.email) editor?.putString(KEY_GENDER, user.gender) editor?.apply() } //this method will logout the user fun logout() { val sharedPreferences = ctx?.getSharedPreferences(SHARED_PREF_NAME, Context.MODE_PRIVATE) val editor = sharedPreferences?.edit() editor?.clear() editor?.apply() ctx?.startActivity(Intent(ctx, LoginActivity::class.java)) } companion object { private val SHARED_PREF_NAME = "volleyregisterlogin" private val KEY_USERNAME = "keyusername" private val KEY_EMAIL = "keyemail" private val KEY_GENDER = "keygender" private val KEY_ID = "keyid" private var mInstance: SharedPrefManager? = null private var ctx: Context? = null @Synchronized fun getInstance(context: Context): SharedPrefManager { if (mInstance == null) { mInstance = SharedPrefManager(context) } return mInstance as SharedPrefManager } } } package example.javatpoint.com.kotlinvolleyregistrationloginsystem import android.content.Context import android.content.Intent class SharedPrefManager private constructor(context: Context) { //this method will checker whether user is already logged in or not val isLoggedIn: Boolean get() { val sharedPreferences = ctx?.getSharedPreferences(SHARED_PREF_NAME, Context.MODE_PRIVATE) return sharedPreferences?.getString(KEY_USERNAME, null) != null } //this method will give the logged in user val user: User get() { val sharedPreferences = ctx?.getSharedPreferences(SHARED_PREF_NAME, Context.MODE_PRIVATE) return User( sharedPreferences!!.getInt(KEY_ID, -1), sharedPreferences.getString(KEY_USERNAME, null), sharedPreferences.getString(KEY_EMAIL, null), sharedPreferences.getString(KEY_GENDER, null) ) } init { ctx = context } //this method will store the user data in shared preferences fun userLogin(user: User) { val sharedPreferences = ctx?.getSharedPreferences(SHARED_PREF_NAME, Context.MODE_PRIVATE) val editor = sharedPreferences?.edit() editor?.putInt(KEY_ID, user.id) editor?.putString(KEY_USERNAME, user.name) editor?.putString(KEY_EMAIL, user.email) editor?.putString(KEY_GENDER, user.gender) editor?.apply() } //this method will logout the user fun logout() { val sharedPreferences = ctx?.getSharedPreferences(SHARED_PREF_NAME, Context.MODE_PRIVATE) val editor = sharedPreferences?.edit() editor?.clear() editor?.apply() ctx?.startActivity(Intent(ctx, LoginActivity::class.java)) } companion object { private val SHARED_PREF_NAME = "volleyregisterlogin" private val KEY_USERNAME = "keyusername" private val KEY_EMAIL = "keyemail" private val KEY_GENDER = "keygender" private val KEY_ID = "keyid" private var mInstance: SharedPrefManager? = null private var ctx: Context? = null @Synchronized fun getInstance(context: Context): SharedPrefManager { if (mInstance == null) { mInstance = SharedPrefManager(context) } return mInstance as SharedPrefManager } } }
Now, in the MainActivity.kt class, we will display the user information if the user is logged-in otherwise, it redirects to LoginActivity.kt class. The onClick() method is used to log-out the user when clicking on the button.
MainActivity.kt
package example.javatpoint.com.kotlinvolleyregistrationloginsystem import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.content.Intent import android.view.View import android.widget.Button import android.widget.TextView class MainActivity : AppCompatActivity(), View.OnClickListener { internal lateinit var id: TextView internal lateinit var userName: TextView internal lateinit var userEmail: TextView internal lateinit var gender: TextView internal lateinit var btnLogout: Button override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) if (SharedPrefManager.getInstance(this).isLoggedIn) { id = findViewById(R.id.textViewId) userName = findViewById(R.id.textViewUsername) userEmail = findViewById(R.id.textViewEmail) gender = findViewById(R.id.textViewGender) btnLogout = findViewById(R.id.buttonLogout) val user = SharedPrefManager.getInstance(this).user id.text = user.id.toString() userEmail.text = user.email gender.text = user.gender userName.text = user.name btnLogout.setOnClickListener(this) } else { val intent = Intent(this@MainActivity, LoginActivity::class.java) startActivity(intent) finish() } } override fun onClick(view: View) { if (view == btnLogout) { SharedPrefManager.getInstance(applicationContext).logout() } } } package example.javatpoint.com.kotlinvolleyregistrationloginsystem import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.content.Intent import android.view.View import android.widget.Button import android.widget.TextView class MainActivity : AppCompatActivity(), View.OnClickListener { internal lateinit var id: TextView internal lateinit var userName: TextView internal lateinit var userEmail: TextView internal lateinit var gender: TextView internal lateinit var btnLogout: Button override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) if (SharedPrefManager.getInstance(this).isLoggedIn) { id = findViewById(R.id.textViewId) userName = findViewById(R.id.textViewUsername) userEmail = findViewById(R.id.textViewEmail) gender = findViewById(R.id.textViewGender) btnLogout = findViewById(R.id.buttonLogout) val user = SharedPrefManager.getInstance(this).user id.text = user.id.toString() userEmail.text = user.email gender.text = user.gender userName.text = user.name btnLogout.setOnClickListener(this) } else { val intent = Intent(this@MainActivity, LoginActivity::class.java) startActivity(intent) finish() } } override fun onClick(view: View) { if (view == btnLogout) { SharedPrefManager.getInstance(applicationContext).logout() } } }
In the LoginActivity.kt class, we check that the user is already logged-in or not, if true then redirect to MainActivity.kt class otherwise, allow a user to log-in. StringRequest class of Volley library is used for network module. The object of StringRequest class takes the parameters of the type request method, URL, and the response.
LoginActivity.kt
package example.javatpoint.com.kotlinvolleyregistrationloginsystem import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.content.Intent import android.text.TextUtils import android.view.View import android.widget.EditText import android.widget.ProgressBar import android.widget.Toast import com.android.volley.AuthFailureError import com.android.volley.Request import com.android.volley.Response import com.android.volley.toolbox.StringRequest import kotlinx.android.synthetic.main.activity_login.* import org.json.JSONException import org.json.JSONObject import java.util.HashMap class LoginActivity : AppCompatActivity() { internal lateinit var etName: EditText internal lateinit var etPassword: EditText internal lateinit var progressBar: ProgressBar override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_login) if (SharedPrefManager.getInstance(this).isLoggedIn) { finish() startActivity(Intent(this, MainActivity::class.java)) } progressBar = findViewById(R.id.progressBar) etName = findViewById(R.id.etUserName) etPassword = findViewById(R.id.etUserPassword) //calling the method userLogin() for login the user btnLogin.setOnClickListener(View.OnClickListener { userLogin() }) //if user presses on textview it call the activity RegisterActivity tvRegister.setOnClickListener(View.OnClickListener { finish() startActivity(Intent(applicationContext, RegisterActivity::class.java)) }) } private fun userLogin() { //first getting the values val username = etName.text.toString() val password = etPassword.text.toString() //validating inputs if (TextUtils.isEmpty(username)) { etName.error = "Please enter your username" etName.requestFocus() return } if (TextUtils.isEmpty(password)) { etPassword.error = "Please enter your password" etPassword.requestFocus() return } //if everything is fine val stringRequest = object : StringRequest(Request.Method.POST, URLs.URL_LOGIN, Response.Listener { response -> progressBar.visibility = View.GONE try { //converting response to json object val obj = JSONObject(response) //if no error in response if (!obj.getBoolean("error")) { Toast.makeText(applicationContext, obj.getString("message"), Toast.LENGTH_SHORT).show() //getting the user from the response val userJson = obj.getJSONObject("user") //creating a new user object val user = User( userJson.getInt("id"), userJson.getString("username"), userJson.getString("email"), userJson.getString("gender") ) //storing the user in shared preferences SharedPrefManager.getInstance(applicationContext).userLogin(user) //starting the MainActivity finish() startActivity(Intent(applicationContext, MainActivity::class.java)) } else { Toast.makeText(applicationContext, obj.getString("message"), Toast.LENGTH_SHORT).show() } } catch (e: JSONException) { e.printStackTrace() } }, Response.ErrorListener { error -> Toast.makeText(applicationContext, error.message, Toast.LENGTH_SHORT).show() }) { @Throws(AuthFailureError::class) override fun getParams(): Map< String, String> { val params = HashMap< String, String>() params["username"] = username params["password"] = password return params } } VolleySingleton.getInstance(this).addToRequestQueue(stringRequest) } } package example.javatpoint.com.kotlinvolleyregistrationloginsystem import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.content.Intent import android.text.TextUtils import android.view.View import android.widget.EditText import android.widget.ProgressBar import android.widget.Toast import com.android.volley.AuthFailureError import com.android.volley.Request import com.android.volley.Response import com.android.volley.toolbox.StringRequest import kotlinx.android.synthetic.main.activity_login.* import org.json.JSONException import org.json.JSONObject import java.util.HashMap class LoginActivity : AppCompatActivity() { internal lateinit var etName: EditText internal lateinit var etPassword: EditText internal lateinit var progressBar: ProgressBar override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_login) if (SharedPrefManager.getInstance(this).isLoggedIn) { finish() startActivity(Intent(this, MainActivity::class.java)) } progressBar = findViewById(R.id.progressBar) etName = findViewById(R.id.etUserName) etPassword = findViewById(R.id.etUserPassword) //calling the method userLogin() for login the user btnLogin.setOnClickListener(View.OnClickListener { userLogin() }) //if user presses on textview it call the activity RegisterActivity tvRegister.setOnClickListener(View.OnClickListener { finish() startActivity(Intent(applicationContext, RegisterActivity::class.java)) }) } private fun userLogin() { //first getting the values val username = etName.text.toString() val password = etPassword.text.toString() //validating inputs if (TextUtils.isEmpty(username)) { etName.error = "Please enter your username" etName.requestFocus() return } if (TextUtils.isEmpty(password)) { etPassword.error = "Please enter your password" etPassword.requestFocus() return } //if everything is fine val stringRequest = object : StringRequest(Request.Method.POST, URLs.URL_LOGIN, Response.Listener { response -> progressBar.visibility = View.GONE try { //converting response to json object val obj = JSONObject(response) //if no error in response if (!obj.getBoolean("error")) { Toast.makeText(applicationContext, obj.getString("message"), Toast.LENGTH_SHORT).show() //getting the user from the response val userJson = obj.getJSONObject("user") //creating a new user object val user = User( userJson.getInt("id"), userJson.getString("username"), userJson.getString("email"), userJson.getString("gender") ) //storing the user in shared preferences SharedPrefManager.getInstance(applicationContext).userLogin(user) //starting the MainActivity finish() startActivity(Intent(applicationContext, MainActivity::class.java)) } else { Toast.makeText(applicationContext, obj.getString("message"), Toast.LENGTH_SHORT).show() } } catch (e: JSONException) { e.printStackTrace() } }, Response.ErrorListener { error -> Toast.makeText(applicationContext, error.message, Toast.LENGTH_SHORT).show() }) { @Throws(AuthFailureError::class) override fun getParams(): Map< String, String> { val params = HashMap< String, String>() params["username"] = username params["password"] = password return params } } VolleySingleton.getInstance(this).addToRequestQueue(stringRequest) } }
The RegisterActivity.kt class is used to register the user. This class initially checks the user log-in, if true then redirect to MainActivity.kt class otherwise, allow user for registration.
Similar to LoginActivity.kt class, we use the StringRequest class of Volley library for network connection and pass the parameters of the type request method, URL, and the response. The Response.Listener handles the response generated by the server.
RegisterActivity.kt
package example.javatpoint.com.kotlinvolleyregistrationloginsystem import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.content.Intent import android.text.TextUtils import android.view.View import android.widget.EditText import android.widget.ProgressBar import android.widget.RadioButton import android.widget.RadioGroup import android.widget.Toast import com.android.volley.AuthFailureError import com.android.volley.Request import com.android.volley.Response import com.android.volley.toolbox.StringRequest import kotlinx.android.synthetic.main.activity_register.* import org.json.JSONException import org.json.JSONObject import java.util.HashMap class RegisterActivity : AppCompatActivity() { internal lateinit var editTextUsername: EditText internal lateinit var editTextEmail: EditText internal lateinit var editTextPassword: EditText internal lateinit var radioGroupGender: RadioGroup internal lateinit var progressBar: ProgressBar override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_register) progressBar = findViewById(R.id.progressBar) //if the user is already logged in we will directly start the MainActivity (profile) activity if (SharedPrefManager.getInstance(this).isLoggedIn) { finish() startActivity(Intent(this, MainActivity::class.java)) return } editTextUsername = findViewById(R.id.editTextUsername) editTextEmail = findViewById(R.id.editTextEmail) editTextPassword = findViewById(R.id.editTextPassword) radioGroupGender = findViewById(R.id.radioGender) buttonRegister.setOnClickListener(View.OnClickListener { //if user pressed on button register //here we will register the user to server registerUser() }) textViewLogin.setOnClickListener(View.OnClickListener { finish() startActivity(Intent(this@RegisterActivity, LoginActivity::class.java)) }) } private fun registerUser() { val username = editTextUsername.text.toString().trim { it <= ' ' } val email = editTextEmail.text.toString().trim { it <= ' ' } val password = editTextPassword.text.toString().trim { it <= ' ' } val gender = (findViewById< View>(radioGroupGender.checkedRadioButtonId) as RadioButton).text.toString() //first we will do the validations if (TextUtils.isEmpty(username)) { editTextUsername.error = "Please enter username" editTextUsername.requestFocus() return } if (TextUtils.isEmpty(email)) { editTextEmail.error = "Please enter your email" editTextEmail.requestFocus() return } if (!android.util.Patterns.EMAIL_ADDRESS.matcher(email).matches()) { editTextEmail.error = "Enter a valid email" editTextEmail.requestFocus() return } if (TextUtils.isEmpty(password)) { editTextPassword.error = "Enter a password" editTextPassword.requestFocus() return } val stringRequest = object : StringRequest(Request.Method.POST, URLs.URL_REGISTER, Response.Listener { response -> progressBar.visibility = View.GONE try { //converting response to json object val obj = JSONObject(response) //if no error in response if (!obj.getBoolean("error")) { Toast.makeText(applicationContext, obj.getString("message"), Toast.LENGTH_SHORT).show() //getting the user from the response val userJson = obj.getJSONObject("user") //creating a new user object val user = User( userJson.getInt("id"), userJson.getString("username"), userJson.getString("email"), userJson.getString("gender") ) //storing the user in shared preferences SharedPrefManager.getInstance(applicationContext).userLogin(user) //starting the MainActivity activity finish() startActivity(Intent(applicationContext, MainActivity::class.java)) } else { Toast.makeText(applicationContext, obj.getString("message"), Toast.LENGTH_SHORT).show() } } catch (e: JSONException) { e.printStackTrace() } }, Response.ErrorListener { error -> Toast.makeText(applicationContext, error.message, Toast.LENGTH_SHORT).show() }) { @Throws(AuthFailureError::class) override fun getParams(): Map< String, String> { val params = HashMap< String, String>() params["username"] = username params["email"] = email params["password"] = password params["gender"] = gender return params } } VolleySingleton.getInstance(this).addToRequestQueue(stringRequest) } } package example.javatpoint.com.kotlinvolleyregistrationloginsystem import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.content.Intent import android.text.TextUtils import android.view.View import android.widget.EditText import android.widget.ProgressBar import android.widget.RadioButton import android.widget.RadioGroup import android.widget.Toast import com.android.volley.AuthFailureError import com.android.volley.Request import com.android.volley.Response import com.android.volley.toolbox.StringRequest import kotlinx.android.synthetic.main.activity_register.* import org.json.JSONException import org.json.JSONObject import java.util.HashMap class RegisterActivity : AppCompatActivity() { internal lateinit var editTextUsername: EditText internal lateinit var editTextEmail: EditText internal lateinit var editTextPassword: EditText internal lateinit var radioGroupGender: RadioGroup internal lateinit var progressBar: ProgressBar override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_register) progressBar = findViewById(R.id.progressBar) //if the user is already logged in we will directly start the MainActivity (profile) activity if (SharedPrefManager.getInstance(this).isLoggedIn) { finish() startActivity(Intent(this, MainActivity::class.java)) return } editTextUsername = findViewById(R.id.editTextUsername) editTextEmail = findViewById(R.id.editTextEmail) editTextPassword = findViewById(R.id.editTextPassword) radioGroupGender = findViewById(R.id.radioGender) buttonRegister.setOnClickListener(View.OnClickListener { //if user pressed on button register //here we will register the user to server registerUser() }) textViewLogin.setOnClickListener(View.OnClickListener { finish() startActivity(Intent(this@RegisterActivity, LoginActivity::class.java)) }) } private fun registerUser() { val username = editTextUsername.text.toString().trim { it <= ' ' } val email = editTextEmail.text.toString().trim { it <= ' ' } val password = editTextPassword.text.toString().trim { it <= ' ' } val gender = (findViewById< View>(radioGroupGender.checkedRadioButtonId) as RadioButton).text.toString() //first we will do the validations if (TextUtils.isEmpty(username)) { editTextUsername.error = "Please enter username" editTextUsername.requestFocus() return } if (TextUtils.isEmpty(email)) { editTextEmail.error = "Please enter your email" editTextEmail.requestFocus() return } if (!android.util.Patterns.EMAIL_ADDRESS.matcher(email).matches()) { editTextEmail.error = "Enter a valid email" editTextEmail.requestFocus() return } if (TextUtils.isEmpty(password)) { editTextPassword.error = "Enter a password" editTextPassword.requestFocus() return } val stringRequest = object : StringRequest(Request.Method.POST, URLs.URL_REGISTER, Response.Listener { response -> progressBar.visibility = View.GONE try { //converting response to json object val obj = JSONObject(response) //if no error in response if (!obj.getBoolean("error")) { Toast.makeText(applicationContext, obj.getString("message"), Toast.LENGTH_SHORT).show() //getting the user from the response val userJson = obj.getJSONObject("user") //creating a new user object val user = User( userJson.getInt("id"), userJson.getString("username"), userJson.getString("email"), userJson.getString("gender") ) //storing the user in shared preferences SharedPrefManager.getInstance(applicationContext).userLogin(user) //starting the MainActivity activity finish() startActivity(Intent(applicationContext, MainActivity::class.java)) } else { Toast.makeText(applicationContext, obj.getString("message"), Toast.LENGTH_SHORT).show() } } catch (e: JSONException) { e.printStackTrace() } }, Response.ErrorListener { error -> Toast.makeText(applicationContext, error.message, Toast.LENGTH_SHORT).show() }) { @Throws(AuthFailureError::class) override fun getParams(): Map< String, String> { val params = HashMap< String, String>() params["username"] = username params["email"] = email params["password"] = password params["gender"] = gender return params } } VolleySingleton.getInstance(this).addToRequestQueue(stringRequest) } }
AndroidManifest.xml
Add the following permission in AndroidManifest.xml file
< uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> < uses-permission android:name="android.permission.INTERNET" /> < uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> < uses-permission android:name="android.permission.INTERNET" />
Output:
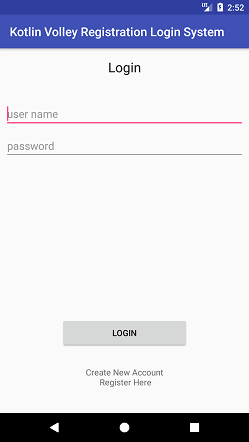
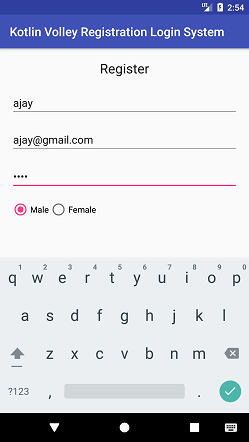
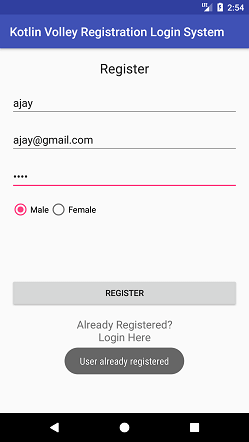
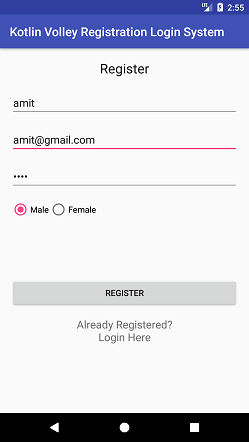
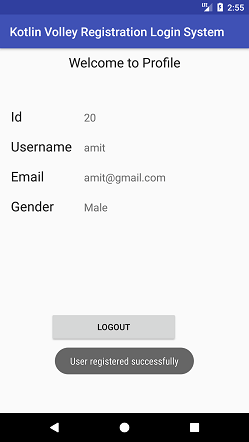
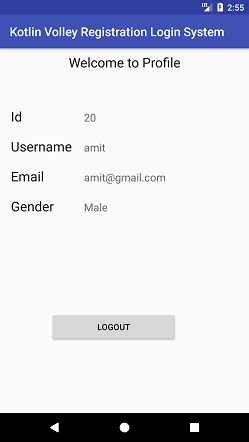
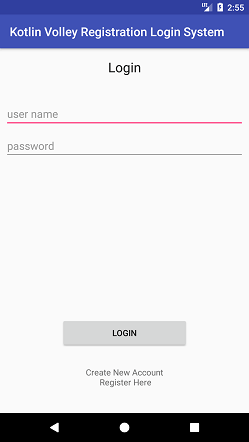
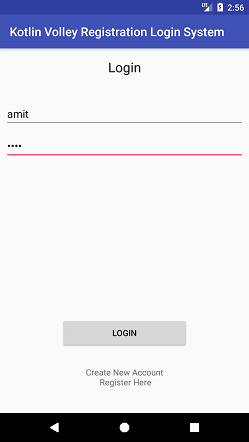
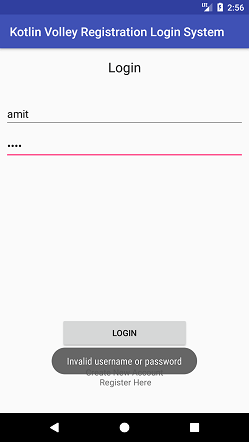
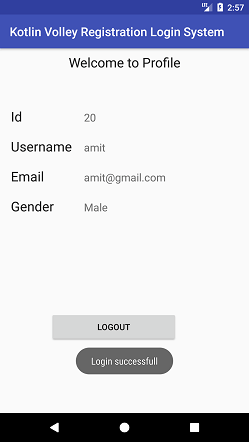