Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
Kotlin Android AlertDialog
Android AlertDialog is a subclass of Dialog class. It is used to prompt a small dialog window to make a decision before a user can proceed in the same activity without changing the screen. A dialog shows the title, message, up to three buttons or a custom layout.
The instance of AlertDialog.Builder class is used to make an alert dialog. Through alert dialog, we create positive (yes), negative (no) and neutral (cancel) decision.
Kotlin Android AlertDialog Example
In this example, we prompt an alert dialog window by clicking a button. This dialog window sets three decision actions as positive, negative and neutral.
activity_main.xml
Add the following code in the activity_main.xml file. In this layout file, we add a button to prompt an alert dialog.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinalertdialog.MainActivity"> < Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="8dp" android:layout_marginEnd="8dp" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:text="@string/button" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> < /android.support.constraint.ConstraintLayout>
strings.xml
In the strings.xml file add the following code.
< resources> < string name="app_name">Kotlin AlertDialog< /string> < string name="button">click button< /string> < string name="dialogTitle">Delete File< /string> < string name="dialogMessage">Deleting file may be harm your system< /string> < /resources>
MainActivity.kt
Add the following code in the MainActivity.kt class. The instance of AlertDialog.Builder class call the setTitle(), setMessage(), setIcon() methods to set the dialog title, message, icon respectively.
To set the action on alert dialog call the setPositiveButton(), setNeutralButton() and setNegativeButton() methods for positive, neutral and negative action respectively. The show() method of AlertDialog.Builder is used to display the alert dialog.
package example.javatpoint.com.kotlinalertdialog import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.support.v7.app.AlertDialog import android.widget.Button import android.widget.Toast class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val button = findViewById< Button>(R.id.button) button.setOnClickListener { val builder = AlertDialog.Builder(this) //set title for alert dialog builder.setTitle(R.string.dialogTitle) //set message for alert dialog builder.setMessage(R.string.dialogMessage) builder.setIcon(android.R.drawable.ic_dialog_alert) //performing positive action builder.setPositiveButton("Yes"){dialogInterface, which -> Toast.makeText(applicationContext,"clicked yes",Toast.LENGTH_LONG).show() } //performing cancel action builder.setNeutralButton("Cancel"){dialogInterface , which -> Toast.makeText(applicationContext,"clicked cancel\n operation cancel",Toast.LENGTH_LONG).show() } //performing negative action builder.setNegativeButton("No"){dialogInterface, which -> Toast.makeText(applicationContext,"clicked No",Toast.LENGTH_LONG).show() } // Create the AlertDialog val alertDialog: AlertDialog = builder.create() // Set other dialog properties alertDialog.setCancelable(false) alertDialog.show() } } }
Output:
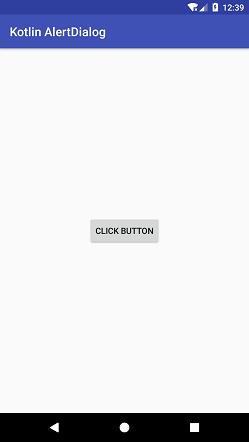
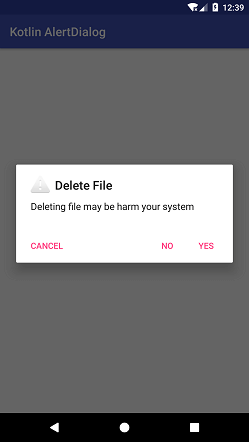
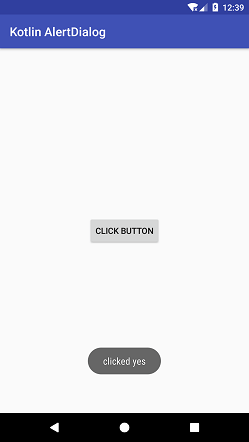