Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
- Kotlin Tutorial
Practical Paper
Industrial Training
XMLPullParser
XML document is commonly used to share the data on the internet. The data provided in XML format are able to update frequently and parsing them is a common task for network-based apps.
In android, there are three types of XML parsers to parse the XML data and read them in android applications.
These parsers are
- DOM Parser
- SAX Parser
- XMLPullParser
Android recommends to use XMLPullParser to parse the XML file rather than SAX and DOM because it is fast.
The org.xmlpull.v1.XmlPullParser interface provides the functionality to parse the XML document using XMLPullParser.
Events of XmlPullParser
The next() method of XMLPullParser moves the cursor pointer to the next event. Generally, we use four constants (works as the event) defined in the XMLPullParser interface.
- START_TAG: An XML start tag will read.
- TEXT: Text content was read the text content can be retrieved using the getText() method.
- END_TAG: An end tag will read.
- END_DOCUMENT: No more events are available.
Example of XML Parsing using XMLPullParser
In this example, we read the XML data and bind them into a ListView using XMLPullParser.
activity_main.xml
Add the ListView in the activity_main.xml layout.
< ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinxmlparsingusingxmlpullparser.MainActivity"> < ListView android:id="@+id/listView" android:layout_width="match_parent" android:layout_height="wrap_content" > < /ListView> < /android.support.constraint.ConstraintLayout> < ?xml version="1.0" encoding="utf-8"?> < android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="example.javatpoint.com.kotlinxmlparsingusingxmlpullparser.MainActivity"> < ListView android:id="@+id/listView" android:layout_width="match_parent" android:layout_height="wrap_content" > < /ListView> < /android.support.constraint.ConstraintLayout>
employees.xml
Create the XML document employees.xml in assets directory to parse the data using XMLPullParser.
< ?xml version="1.0" encoding="UTF-8"?> < employees> < employee> < id>1< /id> < name>Sachin Kumar< /name> < salary>50000< /salary> < /employee> < employee> < id>2< /id> < name>Rahul Kumar< /name> < salary>60000< /salary> < /employee> < employee> < id>3< /id> < name>John Mike< /name> < salary>70000< /salary> < /employee> < employee> < id>4< /id> < name>Ajay Kumar< /name> < salary>45000< /salary> < /employee> < employee> < id>5< /id> < name>Toni Nayer< /name> < salary>55000< /salary> < /employee> < employee> < id>6< /id> < name>Mr Bony< /name> < salary>42000< /salary> < /employee> < employee> < id>7< /id> < name>Raj Kumar< /name> < salary>30000< /salary> < /employee> < employee> < id>8< /id> < name>Rahul Kumar< /name> < salary>60000< /salary> < /employee> < employee> < id>9< /id> < name>John Mike< /name> < salary>70000< /salary> < /employee> < employee> < id>10< /id> < name>Sachin Kumar< /name> < salary>50000< /salary> < /employee> < employee> < id>11< /id> < name>Rahul Kumar< /name> < salary>60000< /salary> < /employee> < employee> < id>12< /id> < name>John Mike< /name> < salary>70000< /salary> < /employee> < /employees> < ?xml version="1.0" encoding="UTF-8"?> < employees> < employee> < id>1< /id> < name>Sachin Kumar< /name> < salary>50000< /salary> < /employee> < employee> < id>2< /id> < name>Rahul Kumar< /name> < salary>60000< /salary> < /employee> < employee> < id>3< /id> < name>John Mike< /name> < salary>70000< /salary> < /employee> < employee> < id>4< /id> < name>Ajay Kumar< /name> < salary>45000< /salary> < /employee> < employee> < id>5< /id> < name>Toni Nayer< /name> < salary>55000< /salary> < /employee> < employee> < id>6< /id> < name>Mr Bony< /name> < salary>42000< /salary> < /employee> < employee> < id>7< /id> < name>Raj Kumar< /name> < salary>30000< /salary> < /employee> < employee> < id>8< /id> < name>Rahul Kumar< /name> < salary>60000< /salary> < /employee> < employee> < id>9< /id> < name>John Mike< /name> < salary>70000< /salary> < /employee> < employee> < id>10< /id> < name>Sachin Kumar< /name> < salary>50000< /salary> < /employee> < employee> < id>11< /id> < name>Rahul Kumar< /name> < salary>60000< /salary> < /employee> < employee> < id>12< /id> < name>John Mike< /name> < salary>70000< /salary> < /employee> < /employees>
Employee.kt
Create a data model class Employee.kt corresponds to the XML data file.
package example.javatpoint.com.kotlinxmlparsingusingxmlpullparser class Employee { var id: Int = 0 var name: String? = null var salary: Float = 0.toFloat() override fun toString(): String { return " Id = $id\n Name = $name\n Salary = $salary" } } package example.javatpoint.com.kotlinxmlparsingusingxmlpullparser class Employee { var id: Int = 0 var name: String? = null var salary: Float = 0.toFloat() override fun toString(): String { return " Id = $id\n Name = $name\n Salary = $salary" } }
XmlPullParserHandler.kt
Write the code to parse the XML file using XMLPullParser. In this class, we return all the employees in the list.
package example.javatpoint.com.kotlinxmlparsingusingxmlpullparser import org.xmlpull.v1.XmlPullParserException import org.xmlpull.v1.XmlPullParser import org.xmlpull.v1.XmlPullParserFactory import java.io.IOException import java.io.InputStream class XmlPullParserHandler { private val employees = ArrayList< Employee>() private var employee: Employee? = null private var text: String? = null fun parse(inputStream: InputStream): List< Employee> { try { val factory = XmlPullParserFactory.newInstance() factory.isNamespaceAware = true val parser = factory.newPullParser() parser.setInput(inputStream, null) var eventType = parser.eventType while (eventType != XmlPullParser.END_DOCUMENT) { val tagname = parser.name when (eventType) { XmlPullParser.START_TAG -> if (tagname.equals("employee", ignoreCase = true)) { // create a new instance of employee employee = Employee() } XmlPullParser.TEXT -> text = parser.text XmlPullParser.END_TAG -> if (tagname.equals("employee", ignoreCase = true)) { // add employee object to list employee?.let { employees.add(it) } } else if (tagname.equals("id", ignoreCase = true)) { employee!!.id = Integer.parseInt(text) } else if (tagname.equals("name", ignoreCase = true)) { employee!!.name = text } else if (tagname.equals("salary", ignoreCase = true)) { employee!!.salary = java.lang.Float.parseFloat(text) } else -> { } } eventType = parser.next() } } catch (e: XmlPullParserException) { e.printStackTrace() } catch (e: IOException) { e.printStackTrace() } return employees } } package example.javatpoint.com.kotlinxmlparsingusingxmlpullparser import org.xmlpull.v1.XmlPullParserException import org.xmlpull.v1.XmlPullParser import org.xmlpull.v1.XmlPullParserFactory import java.io.IOException import java.io.InputStream class XmlPullParserHandler { private val employees = ArrayList< Employee>() private var employee: Employee? = null private var text: String? = null fun parse(inputStream: InputStream): List< Employee> { try { val factory = XmlPullParserFactory.newInstance() factory.isNamespaceAware = true val parser = factory.newPullParser() parser.setInput(inputStream, null) var eventType = parser.eventType while (eventType != XmlPullParser.END_DOCUMENT) { val tagname = parser.name when (eventType) { XmlPullParser.START_TAG -> if (tagname.equals("employee", ignoreCase = true)) { // create a new instance of employee employee = Employee() } XmlPullParser.TEXT -> text = parser.text XmlPullParser.END_TAG -> if (tagname.equals("employee", ignoreCase = true)) { // add employee object to list employee?.let { employees.add(it) } } else if (tagname.equals("id", ignoreCase = true)) { employee!!.id = Integer.parseInt(text) } else if (tagname.equals("name", ignoreCase = true)) { employee!!.name = text } else if (tagname.equals("salary", ignoreCase = true)) { employee!!.salary = java.lang.Float.parseFloat(text) } else -> { } } eventType = parser.next() } } catch (e: XmlPullParserException) { e.printStackTrace() } catch (e: IOException) { e.printStackTrace() } return employees } }
MainActivity.kt
In this class, we send XML data into ArrayAdapter and bind them into ListView.
package example.javatpoint.com.kotlinxmlparsingusingxmlpullparser import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.widget.ArrayAdapter import android.widget.ListView import java.io.IOException class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val listView = findViewById< ListView>(R.id.listView) var employees: List< Employee>? = null try { val parser = XmlPullParserHandler() val istream = assets.open("employees.xml") employees = parser.parse(istream) val adapter = ArrayAdapter(this, android.R.layout.simple_list_item_1, employees) listView.adapter = adapter } catch (e: IOException) { e.printStackTrace() } } } package example.javatpoint.com.kotlinxmlparsingusingxmlpullparser import android.support.v7.app.AppCompatActivity import android.os.Bundle import android.widget.ArrayAdapter import android.widget.ListView import java.io.IOException class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val listView = findViewById< ListView>(R.id.listView) var employees: List< Employee>? = null try { val parser = XmlPullParserHandler() val istream = assets.open("employees.xml") employees = parser.parse(istream) val adapter = ArrayAdapter(this, android.R.layout.simple_list_item_1, employees) listView.adapter = adapter } catch (e: IOException) { e.printStackTrace() } } }
Output:
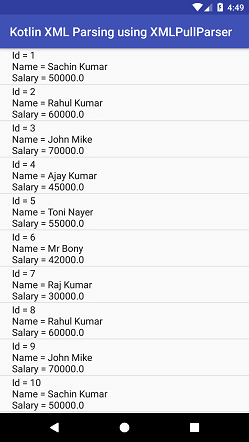