Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
Practical Paper
Industrial Training
Lagrange Interpolation
C Program
Interpolation is the process of estimation of an unknown data by analyzing the given reference data. For the given data, (say ‘y’ at various ‘x’ in tabulated form), the ‘y’ value corresponding to ‘x’ values can be found by interpolation.
In case of equally spaced ‘x’ values, a number of interpolation methods are available such as the Newton’s forward and backward interpolation, Gauss’s forward and backward interpolation, Bessel’s formula, Laplace-Everett’s formula etc. But, all these methods fail when the spacing of ‘x’ is unequal. In such case, Lagrange interpolation is one of the best options.
The source code given below in C program for Lagrange interpolation is for interpolating data in which augments are unequally spaced or in cases where it is not possible to fit the curve of given data. In the code, interpolation is done by following the steps given below:
- As the program is executed, it first asks for number of known data.
- Then, values of x and corresponding y are asked. In Lagrange interpolation in C language, x and y are defined as arrays so that a number of data can be stored under a single variable name.
- After getting the value of x and y, the program displays the input data so that user can correct any incorrectly input data or re-input some missing data.
- The user is asked to input the value of ‘x’ at which the value of ‘y’ is to be interpolated.
- At this step, the value of ‘y’ is computed in loops using Lagrange interpolation formula.
f(x)=xy0+xy1+…………..+ x yn - Finally the value of ‘y’ corresponding to ‘x’ is found.
- At last, user is asked to input ‘1’ to run the program again.
Source Code for Lagrange Interpolation in C:
#include< stdio.h> main() { float x[100],y[100],a,s=1,t=1,k=0; int n,i,j,d=1; printf("\n\n Enter the number of the terms of the table: "); scanf("%d",&n); printf("\n\n Enter the respective values of the variables x and y: \n"); for(i=0; i< n; i++) { scanf ("%f",&x[i]); scanf("%f",&y[i]); } printf("\n\n The table you entered is as follows :\n\n"); for(i=0; i< n; i++) { printf("%0.3f\t%0.3f",x[i],y[i]); printf("\n"); } while(d==1) { printf(" \n\n\n Enter the value of the x to find the respective value of y\n\n\n"); scanf("%f",&a); for(i=0; i< n; i++) { s=1; t=1; for(j=0; j< n; j++) { if(j!=i) { s=s*(a-x[j]); t=t*(x[i]-x[j]); } } k=k+((s/t)*y[i]); } printf("\n\n The respective value of the variable y is: %f",k); printf("\n\n Do you want to continue?\n\n Press 1 to continue and any other key to exit"); scanf("%d",&d); } }
Input/Output:
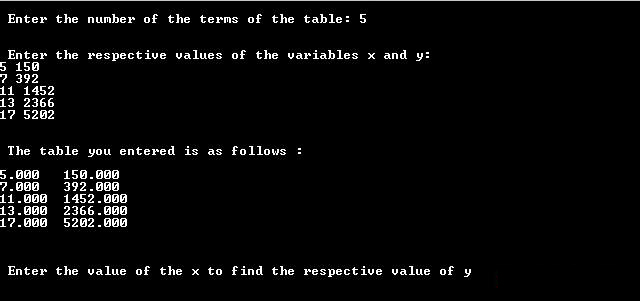
Lagrange interpolation is very simple to implement in computer programming. The code above uses a single header file