Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
Practical Paper
Industrial Training
Curve Fitting
C Program
In some engineering works or scientific experiments, a certain number of data are available in a fixed interval, but they may not sufficient. A data lying in between the interval may be required. In order to find such data, a function or curve needs to be fitted using available data to get the required data through easy and convenient way.
Such technique of approximation of given data into curve which may be liner or of higher degree or exponential is known as curve fitting. It is based on the principle of least square.
A number of manipulations of data are required in curve fitting problems which take a long time to solve and are quite laborious. In order to simplify such calculations using programming approach, here I have presented source code in for linear and exponential curve fitting in C with sample output.
The working procedure of C program for curve fitting (in general) as linear equation is as follows:
Variables Used:
- When the program is executed, it asks for value of number of data, n.
- Then, the user has to input the values of x and corresponding y. In the program, x and y are defined as array. Therefore, x and y are input using for loop.
- After that, the program calculates the sum of x, y, xy, x2 etc.
- Since the data are required to be approximated to linear equation i.e y = ax + b , the values of a and b are to be calculated which is performed by using following formula:
a= (∑y∑x- n∑xy)/((∑x)2 – n∑x2)
b=(∑y – a∑x)/n
- Finally, the program prints the equation y = ax+b on screen.
The working principle of curve fitting C program as exponential equation is also similar to linear but this program first converts exponential equation into linear equation by taking log on both sides as follows:
y = ae^(bx)
lny= bx + lna
Y = Bx + A, which is a linear equation.
Source Code for Linear Curve Fitting in C:
#include< stdio.h> #include< conio.h> #include< math.h> int main() { int n,i,x[20],y[20],sumx=0,sumy=0,sumxy=0,sumx2=0; float a,b; printf("\n C program for Linear Curve Fitting \n "); printf("\n Enter the value of number of terms n:"); scanf("%d",&n); printf("\n Enter the values of x:\n"); for(i=0;i<=n-1;i++) { scanf(" %d",&x[i]); } printf("\n Enter the values of y:"); for(i=0;i<=n-1;i++) { scanf("%d",&y[i]); } for(i=0;i<=n-1;i++) { sumx=sumx +x[i]; sumx2=sumx2 +x[i]*x[i]; sumy=sumy +y[i]; sumxy=sumxy +x[i]*y[i]; } a=((sumx2*sumy -sumx*sumxy)*1.0/(n*sumx2-sumx*sumx)*1.0); b=((n*sumxy-sumx*sumy)*1.0/(n*sumx2-sumx*sumx)*1.0); printf("\n\nThe line is Y=%3.3f +%3.3f X",a,b); return(0); }
Input/Output:
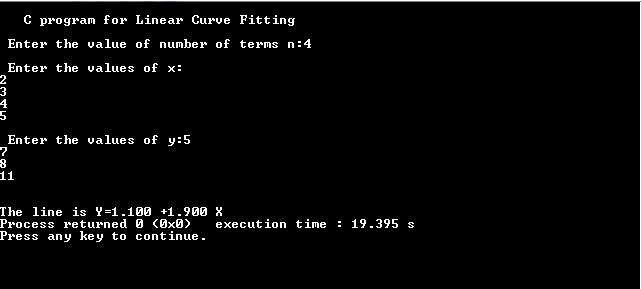
Source Code for Exponential Curve Fitting in C:
#include< stdio.h> #include< conio.h> #include< math.h> int main() { int n,i; float Y[20],sumx=0,sumy=0,sumxy=0,sumx2=0,x[20],y[20]; float a,b,A; printf("\n C program for Exponential Curve fitting\n"); printf("\n Enter the value of number of terms n:"); scanf("%d",&n); printf("\n Enter the values of x:"); for(i=0;i<=n-1;i++) { scanf("%f",&x[i]); } printf("\n Enter the values of y:"); for(i=0;i<=n-1;i++) { scanf("%f",&y[i]); } for(i=0;i<=n-1;i++) { Y[i]=log(y[i]); } for(i=0;i<=n-1;i++) { sumx=sumx +x[i]; sumx2=sumx2 +x[i]*x[i]; sumy=sumy +Y[i]; sumxy=sumxy +x[i]*Y[i]; } A=((sumx2*sumy -sumx*sumxy)*1.0/(n*sumx2-sumx*sumx)*1.0); b=((n*sumxy-sumx*sumy)*1.0/(n*sumx2-sumx*sumx)*1.0); a=exp(A); printf("\n\n The curve is Y= %4.3fe^%4.3fX",a,b); return(0); }
Input/Output:
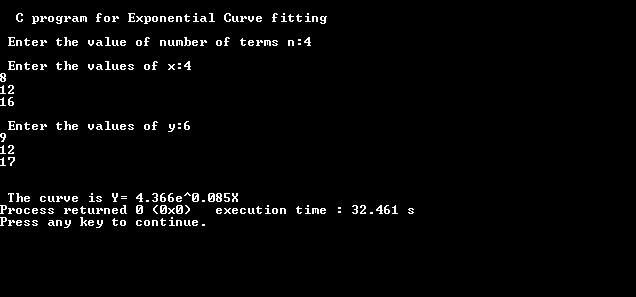
The above given source codes in C language for curve fitting are applicable for linear and exponential approximation of available data or for fitting linear or exponential curves through easy, accurate and fast way.