Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
Practical Paper
Industrial Training
Firebase - Write List Data
In our last chapter, we showed you how to write data in Firebase. Sometimes you need to have a unique identifier for your data. When you want to create unique identifiers for your data, you need to use the push method instead of the set method.
The Push Method
The push() method will create a unique id when the data is pushed. If we want to create our players from the previous chapters with a unique id, we could use the code snippet given below.
var ref = new Firebase('https://tutorialsfirebase.firebaseio.com'); var playersRef = ref.child("players"); playersRef.push ({ name: "John", number: 1, age: 30 }); playersRef.push ({ name: "Amanda", number: 2, age: 20 });
Now our data will look differently. The name will just be a name/value pair like the rest of the properties.
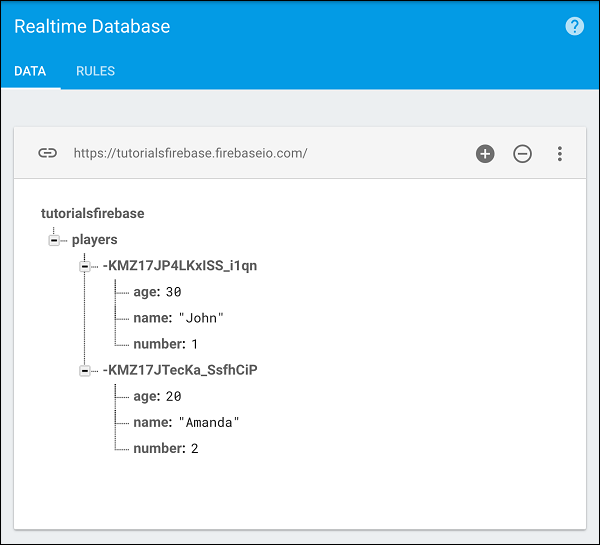
The Key Method
We can get any key from Firebase by using the key() method. For example, if we want to get our collection name, we could use the following snippet.
var ref = new Firebase('https://tutorialsfirebase.firebaseio.com'); var playersRef = ref.child("players"); var playersKey = playersRef.key(); console.log(playersKey);
The console will log our collection name (players).

More on this in our next chapters.