Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
Practical Paper
Industrial Training
Firebase - Google Authentication
In this chapter, we will show you how to set up Google authentication in Firebase.
Step 1 - Enable Google Authentication
Open Firebase dashboard and click Auth on the left side menu. To open the list of available methods, you need to click on SIGN_IN_METHODS in the tab menu.
Now you can choose Google from the list, enable it and save it.
Step 2 - Create Buttons
Inside our index.html, we will add two buttons.
index.html
< button onclick = "googleSignin()"> Google Signin < /button> < button onclick = "googleSignout()"> Google Signout < /button>
Step 3 - Signin and Signout
In this step, we will create Signin and Signout functions. We will use signInWithPopup() and signOut() methods.
Example
Let us consider the following example.
var provider = new firebase.auth.GoogleAuthProvider(); function googleSignin() { firebase.auth() .signInWithPopup(provider).then(function(result) { var token = result.credential.accessToken; var user = result.user; console.log(token) console.log(user) }).catch(function(error) { var errorCode = error.code; var errorMessage = error.message; console.log(error.code) console.log(error.message) }); } function googleSignout() { firebase.auth().signOut() .then(function() { console.log('Signout Succesfull') }, function(error) { console.log('Signout Failed') }); }
After we refresh the page, we can click on the Google Signin button to trigger the Google popup. If signing in is successful, the developer console will log in our user.
We can also click on the Google Signout button to logout from the app. The console will confirm that the logout was successful.
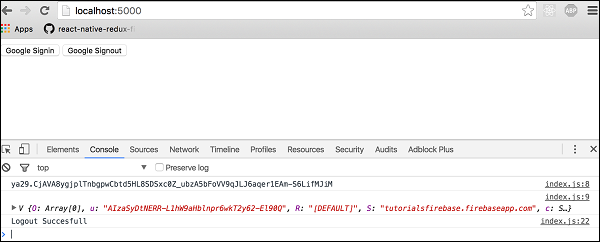