Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
Practical Paper
Industrial Training
Django Templates
Templates are the third and most important part of Django’s MVT Structure. A template in Django is basically written in HTML, CSS and Javascript in an .html file. Django framework efficiently handles and generates dynamically HTML web pages that are visible to end-user. Django mainly functions with a backend so, in order to provide frontend and provide a layout to our website, we use templates. There are two methods of adding the template to our website depending on our needs.
We can use a single template directory which will be spread over the entire project. For each app of our project, we can create a different template directory.
For our current project, we will create a single template directory which will be spread over the entire project for simplicity. App-level templates are generally used in big projects or in case we want to provide a different layout to each component of our webpage.
Configuration
Django Templates can be configured in app_name/settings.py,
TEMPLATES = [ { # Template backend to be used, For example Jinja 'BACKEND': 'django.template.backends.django.DjangoTemplates', # Directories for templates 'DIRS': [], 'APP_DIRS': True, # options to configure 'OPTIONS': { 'context_processors': [ 'django.template.context_processors.debug', 'django.template.context_processors.request', 'django.contrib.auth.context_processors.auth', 'django.contrib.messages.context_processors.messages', ], }, }, ]Using Django Templates
Illustration of How to use templates in Django using an Example Project. Templates not only show static data but also the data form different databases connected to the application through a context dictionary. Consider a project named mcatutorials having an app named mcatutorials.
Refer to the following articles to check how to create a project and an app in Django.
To render a template one needs a view and a url mapped to that view. Let’s begin by creating a view in mcatutorials/views.py,
# import Http Response from django from django.shortcuts import render # create a function def mcatutorials_view(request): # create a dictionary to pass # data to the template context ={ "data":"Gfg is the best", "list":[1, 2, 3, 4, 5, 6, 7, 8, 9, 10] } # return response with template and context return render(request, "mcatutorials.html", context)
Now we need to map a URL to render this view,
from django.urls import path # importing views from views..py from .views import mcatutorials_view urlpatterns = [ path('', mcatutorials_view), ]
Finally create a template in templates/mcatutorials.html,
< !DOCTYPE html> < html lang="en"> < head> < meta charset="UTF-8"> < meta name="viewport" content="width=device-width, initial-scale=1.0"> < meta http-equiv="X-UA-Compatible" content="ie=edge"> < title>Homepage< /title> < /head> < body> < h1>Welcome to Mcatutorials.< /h1> < p> Data is {{ data }}< /p> < h4>List is < /h4> < ul> {% for i in list %} < li>{{ i }}< /li> {% endfor %} < /body> < /html>
Let’s check if it is working,
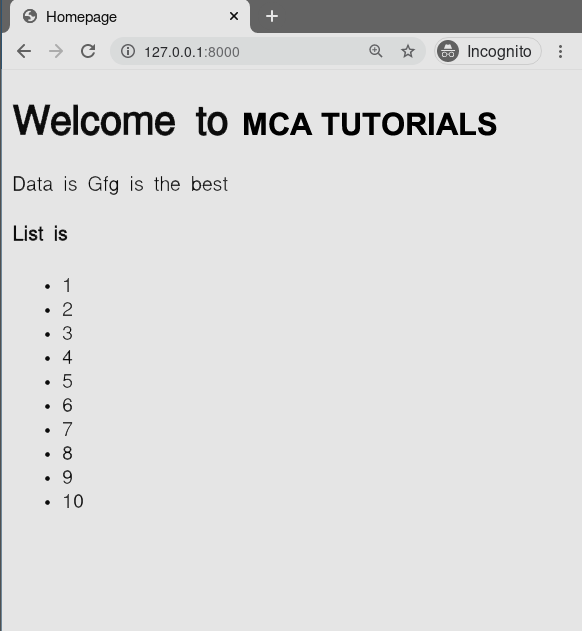
This is one of most important facilities provided by Django Templates. A Django template is a text document or a Python string marked-up using the Django template language. Some constructs are recognized and interpreted by the template engine. The main ones are variables and tags. As we used for loop in above example, we used it as a tag. similarly, we can used various other conditions such as if, else, if-else, empty, etc. Main characterisitcs of django Template language are Variables, Tags, Filters and Comments.
VariablesVariables outputs a value from the context, which is a dict-like object mapping keys to values. The context object we sent from the view can be accesses in template using variables of Django Template.
Syntax{{ variable_name }}Example
Variables are surrounded by {{ and }} like this:
My first name is {{ first_name }}. My last name is {{ last_name }}.
With a context of {'first_name': 'Naveen', 'last_name': 'Arora'}, this template renders to:
My first name is Naveen. My last name is Arora.
To know more about Django Template Variables visit – variables – Django Templates
TagsTags provide arbitrary logic in the rendering process. For example, a tag can output content, serve as a control structure e.g. an “if” statement or a “for” loop, grab content from a database, or even enable access to other template tags.
Syntax{% tag_name %}Example
Tags are surrounded by {% and %} like this:
{% csrf_token %}
Most tags accept arguments, for example :
{% cycle 'odd' 'even' %}
COMMONLY USED TAGS | ||
---|---|---|
Comment | cycle | extends |
if | for loop | for … empty loop |
Boolean Operators | firstof | include |
lorem | now | url |
Django Template Engine provides filters are used to transform the values of variables and tag arguments. We have already discussed major Django Template Tags. Tags can’t modify value of a variable whereas filters can be used for incrementing value of a variable or modifying it to one’s own need.
Syntax{{ variable_name | filter_name }}
Filters can be “chained.” The output of one filter is applied to the next. {{ text|escape|linebreaks }} is a common idiom for escaping text contents, then converting line breaks to < p> tags.
Example{{ value | length }}
If value is [‘a’, ‘b’, ‘c’, ‘d’], the output will be 4.
MAJOR TEMPLATE FILTERS | ||
---|---|---|
add | addslashes | capfirst |
center | cut | date |
default | dictsort | divisibleby |
escape | filesizeformat | first |
join | last | length |
linenumbers | lower | make_list |
random | slice | slugify |
time | timesince | title |
unordered_list | upper | wordcount |
Template ignores everything between {% comment %} and {% endcomment %}. An optional note may be inserted in the first tag. For example, this is useful when commenting out code for documenting why the code was disabled.
Syntax{% commment 'comment_name' %} {% endcomment %}Example
{% comment "Optional note" %} Commented out text with {{ create_date|date:"c" }} {% endcomment %}
To know more about using comments in Templates, visit comment – Django template tags
Template InheritanceThe most powerful and thus the most complex part of Django’s template engine is template inheritance. Template inheritance allows you to build a base “skeleton” template that contains all the common elements of your site and defines blocks that child templates can override.extendstag is used for inheritance of templates in django. One needs to repeat the same code again and again. Usingextendswe can inherit templates as well as variables.
Syntax{% extends 'template_name.html' %}Example
assume the following directory structure:
dir1/ template.html base2.html my/ base3.html base1.html
In template.html, the following paths would be valid:
{% extends "./base2.html" %} {% extends "../base1.html" %} {% extends "./my/base3.html" %}
To know more about Template inheritance and extends, visit extends – Django Template Tags