Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
Practical Paper
Industrial Training
Django Basic App Model – Makemigrations and Migrate
In this article, we will create a basic model of an app. Say, we have a project mcatutorials in which we will create a new app in order to simplify and make independent model units.
To create an app run command through terminal :
python manage.py startapp mcatutorials
and add mcatutorials to INSTALLED_APPS list in settings.py. Now directory structure of the app will be,
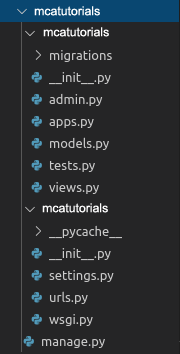
Now go to models.py in mcatutorials app, Here we will create our first model. To create a model you need to first import the Model from django.db.models library.
Now models.py will look like,
# importing Model from django from django.db.models import Model
According to Django documentation, A model is the single, definitive source of information about your data. It contains the essential fields and behaviours of the data you’re storing. Generally, each model maps to a single database table.
Django provides a number of predefined fields and methods to create a Model. To create a model you need to specify a model name first.
Enter the following code into models.py
from django.db import models from django.db.models import Model # Created an empty model class McatutorialsModel(Model): pass
The similar syntax would always be used to create a model.
After making any change in any of app’s models file you need to run following command from the terminal
Python manage.py makemigrations
After this command run following command to finally implement database changes accordingly
Python manage.py migrate
After you run makemigrations and migrate a new table would have been created in database. You can check it from mcatutorials -> makemigrations -> 0001_initial.py.
# Generated by Django 2.2.5 on 2019-09-25 06:00 from django.db import migrations, models class Migration(migrations.Migration): initial = True dependencies = [] operations = [ migrations.CreateModel( name ='McatutorialsModel', fields =[ ('id', models.AutoField(auto_created = True, primary_key = True, serialize = False, verbose_name ='ID')),], ), ]
Let’s understand clearly what Makemigrations and Migrate do.
Makemigrations –makemigrations basically generates the SQL commands for preinstalled apps (which can be viewed in installed apps in settings.py) and your newly created apps’ model which you add in installed apps. It does not execute those commands in your database file. So tables are not created after makemigrations. After applying makemigrations you can see those SQL commands with sqlmigrate which shows all the SQL commands which have been generated by makemigrations. To check more about makemigrations visit – Django App Model – Python manage.py makemigrations command
Migrate –
migrate executes those SQL commands in the database file. So after executing migrate all the tables of your installed apps are created in your database file. You can confirm this by installing sqlite browser and opening db.sqlite3 you can see all the tables appears in the database file after executing migrate command. To check more about makemigrations visit Django manage.py migrate command | Python