Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
Practical Paper
Industrial Training
Stack Organization
Stack Pointer
Stack is a storage structure that stores information in such a way that the last item stored is the first item retrieved. It is based on the principle of LIFO (Last-in-first-out). The stack in digital computers is a group of memory locations with a register that holds the address of top of element. This register that holds the address of top of element of the stack is called Stack Pointer
The two operations of a stack are:
1. Push: Inserts an item on top of stack.
2. Pop: Deletes an item from top of stack.
Implementation of Stack
1. Register Stack
2. Memory Stack
Register Stack
A stack can be organized as a collection of finite number of registers that are used to store temporary information during the execution of a program. The stack pointer (SP) is a register that holds the address of top of element of the stack.
Memory Stack
A stack can be implemented in a random access memory (RAM) attached to a CPU. The implementation of a stack in the CPU is done by assigning a portion of memory to a stack operation and using a processor register as a stack pointer. The starting memory location of the stack is specified by the processor register as stack pointer.
About Stack Organization
What is the stack? It's a special region of your computer's memory that stores temporary variables created by each function (including the main() function). The stack is a "FILO" (first in, last out) data structure, that is managed and optimized by the CPU quite closely. Every time a function declares a new variable, it is "pushed" onto the stack. Then every time a function exits, all of the variables pushed onto the stack by that function, are freed (that is to say, they are deleted). Once a stack variable is freed, that region of memory becomes available for other stack variables.
The advantage of using the stack to store variables, is that memory is managed for you. You don't have to allocate memory by hand, or free it once you don't need it any more. What's more, because the CPU organizes stack memory so efficiently, reading from and writing to stack variables is very fast.
A key to understanding the stack is the notion that when a function exits, all of its variables are popped off of the stack (and hence lost forever). Thus stack variables are local in nature. This is related to a concept we saw earlier known as variable scope, or local vs global variables. A common bug in C programming is attempting to access a variable that was created on the stack inside some function, from a place in your program outside of that function (i.e. after that function has exited).
Another feature of the stack to keep in mind, is that there is a limit (varies with OS) on the size of variables that can be store on the stack. This is not the case for variables allocated on the heap.
To summarize the stack:
- the stack grows and shrinks as functions push and pop local variables
- there is no need to manage the memory yourself, variables are allocated and freed automatically
- the stack has size limits
- stack variables only exist while the function that created them, is running
The stack in Digital Computer is essentially a memory unit with an address register that can count only (after an initial value is loaded into it.) The register that holds the address for the stack is called a Stack Pointer (SP) because its values always points at the top item in the stack.
The two operations – PUSH (insert)
- Pop (delete)
Cafeteria tray example
As an example of how a stack works, consider a spring-loaded tray dispenser of the type often found in cafeterias. Let us say that each tray has number engraved upon it. One tray at a time is loaded in from the top, each resting on the already loaded trays with the spring compressing to make room for more trays as necessary. For example, in Figure 1.1, the trays numbered 42, 23, 2, and 9 are loaded onto the stack of trays with 42 loaded first and 9 loaded last.
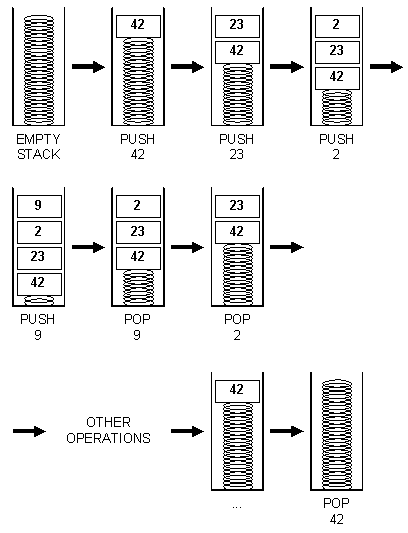
The "Last In" tray is number 9. Thus, the "First Out" tray is also number 9. As customers remove trays from the top of the stack, the first tray removed is tray number 9, and the second is tray number 2. Let us say that at this point more trays were added. These trays would then have to come off the stack before the very first tray we loaded. After any sequence of pushes and pops of the stack of trays, tray 42 would still be on the bottom. The stack would be empty once again only after tray 42 had been popped from the top of the stack.
A useful feature that is included in the CPU of most computers is a stack or last-in first out (LIFO) list. A stack is a storage device that stores information in such a manner that the item stored last is the first item retrieved. The operation a stack can be companied to a stack of trays.
Register Stack
A stack can be placed in a portion of a large memory as it can be organized as a collection of a finite number of memory words as register.
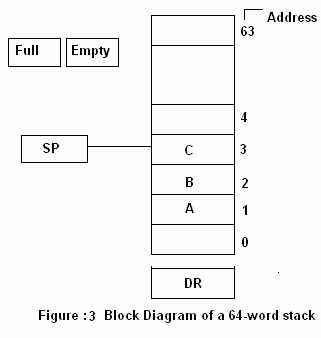
In a 64- word stack, the stack pointer contains 6 bits because 26 = 64.
The one bit register FULL is set to 1 when the stack is full, and the one-bit register EMTY is set to 1 when the stack is empty. DR is the data register that holes the binary data to be written into on read out of the stack.
Initially, SP is decide to O, EMTY is set to 1, FULL = 0, so that SP points to the word at address O and the stack is masked empty and not full.
PUSH SP ® SP + 1 increment stack pointer
M [SP] ® DR unit item on top of the Stack
It (SP = 0) then (FULL ® 1) check it stack is full
EMTY ® 0 mask the stack not empty.
POP DR ® [SP] read item trans the top of stack
SP ® SP –1 decrement SP
It (SP = 0) then (EMTY ® 1) check it stack is empty
FULL ® 0 mark the stack not full. A stack can be placed in a portion of a large memory or it can be organized as
a collection of a finite number of memory words or registers. Figure X shows the
organization of a 64-word register stack. The stack pointer register SP contains a
binary number whose value is equal to the address of the word that is currently on top
of the stack. Three items are placed in the stack: A, B, and C in the order. item C is
on the top of the stack so that the content of sp is now 3. To remove the top item, the
stack is popped by reading the memory word at address 3 and decrementing the content of SP. Item B is now on top of the stack since SP holds address 2. To insert a
new item, the stack is pushed by incrementing SP and writing a word in the next
higher location in the stack. Note that item C has read out but not physically removed.
This does not matter because when the stack is pushed, a new item is written in its
place.
In a 64-word stack, the stack pointer contains 6 bits because 26
=64. since SP
has only six bits, it cannot exceed a number grater than 63(111111 in binary). When
63 is incremented by 1, the result is 0 since 111111 + 1 =1000000 in binary, but SP
can accommodate only the six least significant bits. Similarly, when 000000 is
decremented by 1, the result is 111111. The one bit register Full is set to 1 when the
stack is full, and the one-bit register EMTY is set to 1 when the stack is empty of
items. DR is the data register that holds the binary data to be written in to or read out
of the stack.
Initially, SP is cleared to 0, Emty is set to 1, and Full is cleared to 0, so that SP points
to the word at address o and the stack is marked empty and not full. if the stack is not
full , a new item is inserted with a push operation. the push operation is implemented
with the following sequence of micro-operation.
SP ←SP + 1 (Increment stack pointer)
M(SP) ← DR (Write item on top of the stack)
if (sp=0) then (Full ← 1) (Check if stack is full)
Emty ← 0 ( Marked the stack not empty)
The stac pointer is incremented so that it points to the address of the next-higher word. A memory write operation inserts the word from DR into the top of the stack. Note that SP holds the address of the top of the stack and that M(SP) denotes the memory word specified by the address presently available in SP, the first item stored in the stack is at address 1. The last item is stored at address 0, if SP reaches 0, the stack is full of item, so FULLL is set to 1. This condition is reached if the top item prior to the last push was in location 63 and after
increment SP, the last item stored in location 0. Once an item is stored in location 0, there are no more empty register in the stack. If an item is written in the stack, Obviously the stack can not be empty, so EMTY is cleared to 0. DR← M[SP] Read item from the top of stack SP ← SP-1 Decrement stack Pointer if( SP=0) then (Emty ← 1) Check if stack is empty FULL ← 0 Mark the stack not full The top item is read from the stack into DR. The stack pointer is then decremented. if its value reaches zero, the stack is empty, so Emty is set to 1. This condition is reached if the item read was in location 1. once this item is read out , SP is decremented and reaches the value 0, which is the initial value
of SP. Note that if a pop operation reads the item from location 0 and then SP is decremented, SP changes to 111111, which is equal to decimal 63. In this configuration, the word in address 0 receives the last item in the stack. Note also that an erroneous operation will result if the stack is pushed when FULL=1 or popped when EMTY =1.