Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
Practical Paper
Industrial Training
Flutter TextField
A TextField or TextBox is an input element which holds the alphanumeric data, such as name, password, address, etc. It is a GUI control element that enables the user to enter text information using a programmable code. It can be of a single-line text field (when only one line of information is required) or multiple-line text field (when more than one line of information is required).
TextField in Flutter is the most commonly used text input widget that allows users to collect inputs from the keyboard into an app. We can use the TextField widget in building forms, sending messages, creating search experiences, and many more. By default, Flutter decorated the TextField with an underline. We can also add several attributes with TextField, such as label, icon, inline hint text, and error text using an InputDecoration as the decoration. If we want to remove the decoration properties entirely, it is required to set the decoration to null.
The following code explains a demo example of TextFiled widget in Flutter:
TextField ( decoration: InputDecoration( border: InputBorder.none, labelText: 'Enter Name', hintText: 'Enter Your Name' ), );
Some of the most common attributes used with the TextField widget are as follows:
- decoration: It is used to show the decoration around TextField.
- border: It is used to create a default rounded rectangle border around TextField.
- labelText: It is used to show the label text on the selection of TextField.
- hintText: It is used to show the hint text inside TextField.
- icon: It is used to add icons directly to the TextField.
We are going to see how to use TextField widget in the Flutter app through the following steps:
Step 1: Create a Flutter project in the IDE you used. Here, I am going to use Android Studio.
Step 2: Open the project in Android Studio and navigate to the lib folder. In this folder, open the main.dart file and import the material.dart package as given below:
import 'package:flutter/material.dart';
Step 3: Next, call the main MyApp class using void main run app function and then create your main widget class named as MyApp extends with StatefulWidget:
void main() => runApp( MyApp() ); class MyApp extends StatefulWidget { }
Step 4: Next, we need to create the Scaffold widget -> Column widget in the class widget build area as given below:
class MyApp extends StatefulWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Flutter TextField Example'), ), body: Padding( padding: EdgeInsets.all(15), child: Column( children: < Widget> [ ] ) ) ) ); } }
Step 5: Finally, create the TextField widget as the below code.
child: TextField( obscureText: true, decoration: InputDecoration( border: OutlineInputBorder(), labelText: 'Password', hintText: 'Enter Password', ), ),
Let us see the complete source code that contains the TextField Widget. This Flutter application takes two TextFields and one RaisedButton. After filling the details, the user clicks on the button. Since we have not specified any value in the onPressed () property of the button, it cannot print them to console.
Replace the following code in the main.dart file and see the output.
import 'package:flutter/material.dart'; void main() { runApp(MaterialApp( home: MyApp(),)); } class MyApp extends StatefulWidget { @override _State createState() => _State(); } class _State extends State< MyApp> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Flutter TextField Example'), ), body: Padding( padding: EdgeInsets.all(15), child: Column( children: < Widget>[ Padding( padding: EdgeInsets.all(15), child: TextField( decoration: InputDecoration( border: OutlineInputBorder(), labelText: 'User Name', hintText: 'Enter Your Name', ), ), ), Padding( padding: EdgeInsets.all(15), child: TextField( obscureText: true, decoration: InputDecoration( border: OutlineInputBorder(), labelText: 'Password', hintText: 'Enter Password', ), ), ), RaisedButton( textColor: Colors.white, color: Colors.blue, child: Text('Sign In'), onPressed: (){}, ) ], ) ) ); } }
Output:
When we run the application in android emulator, we should get UI similar to the following screenshot:
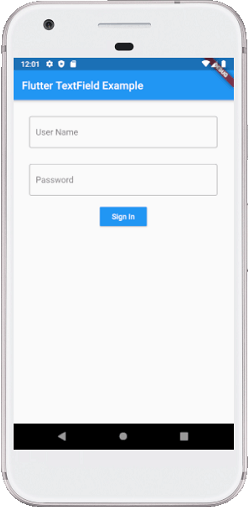
If we click inside the text box, we can see that a keyboard has appeared from the bottom of the screen, the label goes into the top left corner of the border, and the hint text shown into the field. The below screenshot explains it more clearly:
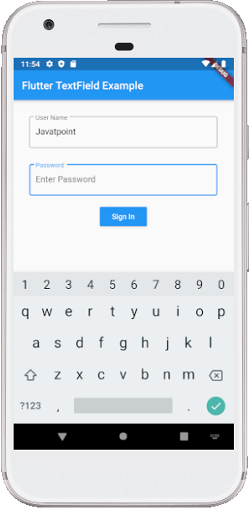
How to retrieve the value of a TextField?
We know that Flutter does not have an ID like in Android for the TextField widget. Flutter allows the user to retrieve the text in mainly two ways: First is the onChanged method, and another is the controller method. Both are discussed below:
1. onChanged method: It is the easiest way to retrieve the text field value. This method store the current value in a simple variable and then use it in the TextField widget. Below is the sample example:
String value = ""; TextField( onChanged: (text) { value = text; }, )
2. Controller method: It is a popular method to retrieve text field value using TextEditingController. It will be attached to the TextField widget and then listen to change and control the widget's text value. Below is the sample code:
TextEditingController mycontroller = TextEditingController(); TextField( controller: mycontroller, )
Sample code for listening to the changes.
controller.addListener(() { // Do something here });
Sample code to get or set the value.
print(controller.text); // Print current value controller.text = "Demo Text"; // Set new value
Let us see the second way in detail to retrieve the text field value in Flutter application with the help of following steps:
- Create a TextEditingController.
- Attach the TextEditingController to a TextField using controller property.
- Retrieve the value of the TextField by using the text() method provided by the TextEditingController.
Now, create a new Flutter project in your IDE and open the main.dart file. Replace the below code in the main.dart file. In this example, we are going to display the alert dialog with the current value of the text field when the user taps on a button.
import 'package:flutter/material.dart'; void main() { runApp(MaterialApp( home: MyApp(),)); } class MyApp extends StatefulWidget { @override _State createState() => _State(); } class _State extends State< MyApp> { TextEditingController nameController = TextEditingController(); TextEditingController passwordController = TextEditingController(); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Flutter TextField Example'), ), body: Padding( padding: EdgeInsets.all(15), child: Column( children: < Widget>[ Padding( padding: EdgeInsets.all(15), child: TextField( controller: nameController, decoration: InputDecoration( border: OutlineInputBorder(), labelText: 'User Name', hintText: 'Enter Your Name', ), ), ), Padding( padding: EdgeInsets.all(15), child: TextField( controller: passwordController, obscureText: true, decoration: InputDecoration( border: OutlineInputBorder(), labelText: 'Password', hintText: 'Enter Password', ), ), ), RaisedButton( textColor: Colors.white, color: Colors.blue, child: Text('Sign In'), onPressed: (){ return showDialog( context: context, builder: (context) { return AlertDialog( title: Text("Alert Message"), // Retrieve the text which the user has entered by // using the TextEditingController. content: Text(nameController.text), actions: < Widget>[ new FlatButton( child: new Text('OK'), onPressed: () { Navigator.of(context).pop(); }, ) ], ); }, ); }, ) ], ) ) ); } }
Output:
When we run the application in android emulator, we should get UI similar to the following screenshot:
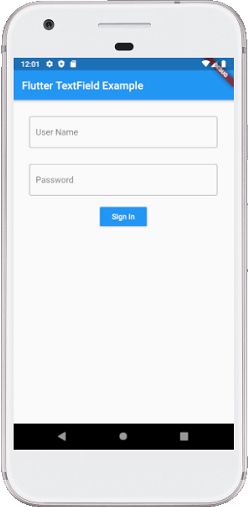
Click inside the text box and add values, as shown in the field. When we press the sign-in button, an alert dialog box appeared containing the text that the user has entered into the field. If we click on the OK button, the alert dialog will disappear. Look into the below screenshot:
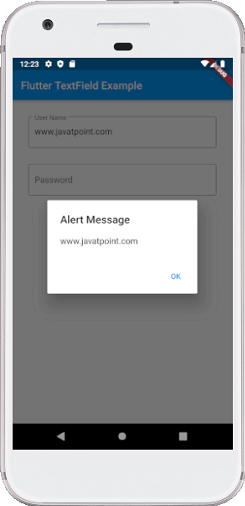
How to make TextField expandable?
Sometimes, we want to expand a TextField that means it can have more than one line. Flutter can do this very easily by adding the attributes maxLines and set it to null, which is one by default. We can also specify the exact value to expand the number of lines by default.
TextField( maxLines: 4, )
How to control the size of TextField value?
TextField widget also allows us to restrict the maximum number of characters inside the text field. We can do this by adding the maxLength attributes as below:
TextField( maxLength: 10, ),
How to obscure text field value?
Obscure means to make the field not readable or not understandable easily. The obscure text cannot visible clear. In Flutter, it is mainly used with a text field that contains a password. We can make the value in a TextField obscure by setting the obscureText to true.
TextField( obscureText: true, ),