Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
Practical Paper
Industrial Training
Flutter Tabbar
In this section, we are going to learn how the tab bar works in Flutter. The tabs are mainly used for mobile navigation. The styling of tabs is different for different operating systems. For example, it is placed at the top of the screen in android devices while it is placed at the bottom in iOS devices.
Working with tabs is a common pattern in Android and iOS apps that follow the Material Design guidelines. Flutter provides a convenient way to create a tab layout. To add tabs to the app, we need to create a TabBar and TabBarView and attach them with the TabController. The controller will sync both so that we can have the behavior which we need.
Let us see step by step to create a tab bar in Flutter application.
Step 1: First, you need to create a Flutter project in your IDE. Here, I am going to use Android Studio.
Step 2: Open the app in Android Studio and navigate to the lib folder. Inside the lib folder, create two dart files and named it FirstScreen and SecondScreen.
Write the following code in the FirstScreen:
import 'package:flutter/material.dart'; class FirstScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Container( child: Center( child: Text('It is a contact tab, which is responsible for displaying the contacts stored in your mobile', style: TextStyle(fontSize: 32.0), ) ), ); } }
Write the following code in the SecondScreen:
import 'package:flutter/material.dart'; class SecondScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Container( child: Center( child: Text('It is a second layout tab, which is responsible for taking pictures from your mobile.', style: TextStyle(fontSize: 35.0), ), ), ); } }
Step 3: Next, we need to create a DefaultTabController. The DefaultTabController creates a TabController and makes it available to all widgets.
DefaultTabController( // The number of tabs to display. length: 2, child: // Complete this code in the next step. );
In the above code, the length property tells about the number of tabs used in the app.
Step 4: Create the tab. We can create tabs by using the TabBar widget as below code.
DefaultTabController( length: 2, child: Scaffold( appBar: AppBar( bottom: TabBar( tabs: [ Tab(icon: Icon(Icons.directions_car)), Tab(icon: Icon(Icons.directions_bike)), ], ), ), ), );
Step 5: Create content for each tab so that when a tab is selected, it displays the content. For this purpose, we have to use the TabBarView widget as:
TabBarView( children: [ ], );
Step 6: Finally, open the main.dart file and insert the following code
import 'package:flutter/material.dart'; import './FirstScreen.dart'; import './SecondScreen.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: DefaultTabController( length: 2, child: Scaffold( appBar: AppBar( title: Text('Flutter Tabs Demo'), bottom: TabBar( tabs: [ Tab(icon: Icon(Icons.contacts), text: "Tab 1"), Tab(icon: Icon(Icons.camera_alt), text: "Tab 2") ], ), ), body: TabBarView( children: [ FirstScreen(), SecondScreen(), ], ), ), ), ); } }
Output
Now, run the app in your Android Studio. It will give the following screen where you can see two tab icons. So when you click any of the tab icons, it will display the associated screen.
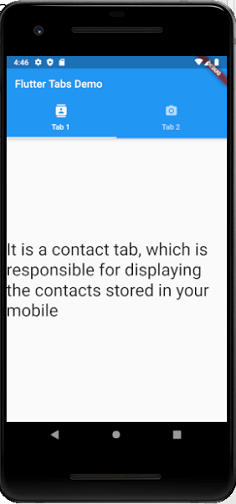
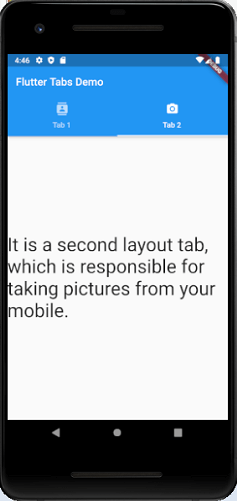
Display a SnackBar
SnackBar gives a brief message at the bottom of the screen on mobile devices and lower-left on larger devices and gets confirmation from the user. The snack bar automatically disappears after a certain amount of time.
Here, we are going to learn how we can implement a snack bar in Flutter. In Flutter, the snack bar only works with a scaffold widget context.
Let us take an example where we show the list of records, and contains a delete icon corresponding to every list. When we delete any record, it shows a message at the bottom of your screen. This message gets confirmation from the user. If it does not receive any confirmation, the message goes disappear automatically. The following example helps to understand it more clearly.
Create a Flutter project in Android Studio. Open the main.dart file and replace the following code.
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( theme: ThemeData( primarySwatch: Colors.green, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State< MyHomePage> { var lists = List< String>.generate(11, (index) => "List : $index"); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Flutter SnackBar Demo'), ), body: ListView.builder( itemCount: lists.length, itemBuilder: (context, index) { return ListTile( title: Text(lists[index]), trailing: Container( width: 60, child: FlatButton( child: Icon( Icons.delete, color: Colors.grey, ), onPressed: () { showSnackBar(context, index); }, ), ), ); }, ), ); } void showSnackBar(BuildContext context, int index) { var deletedRecord = lists[index]; setState(() { lists.removeAt(index); }); SnackBar snackBar = SnackBar( content: Text('Deleted $deletedRecord'), action: SnackBarAction( label: "UNDO", onPressed: () { setState(() { lists.insert(index, deletedRecord); }); }, ), ); Scaffold.of(context).showSnackBar(snackBar); } }
Output
Now, run the app in Android Studio. You can see the following screen in the emulator. When you delete any list, it shows a message at the bottom of the screen. If you tap on the undo, it does not remove the list.
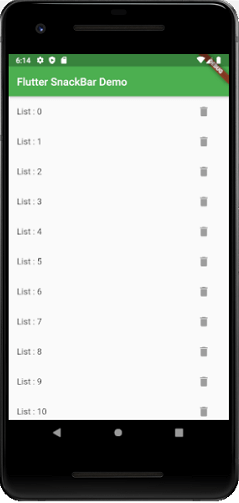
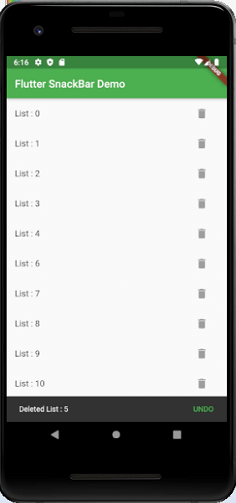