Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- Flutter Tutorials
Practical Paper
Industrial Training
Flutter Toast Notification
Flutter Toast is also called a Toast Notification message. It is a very small message which mainly popup at the bottom of the device screen. It will disappear on its own after completing the time provided by the developers. A developer mostly used the toast notification for showing feedback on the operation performed by the user.
Showing toast notification message is an essential feature in android applications. We can achieve it by using simple lines of code. In this section, we are going to learn how to show toast message in android and iOS by implementing it in Flutter. To implement toast notification, we need to import fluttertoast library in Flutter.
The following steps are needed to show toast notification in Flutter:
- Create a Flutter Project
- Add the Flutter Toast Dependencies in project
- Import the fluttertoast dart package in library
- Implement the code for showing toast message in Flutter
Flutter provides several properties to the user for showing the toast message, which is given below:
Property | Description |
msg | String(Required) |
toastlength | Toast.LENGTH_SHORT or Toast.LENGTH_LONG |
gravity | ToastGravity.TOP or ToastGravity.CENTER or ToastGravity.BOTTOM |
timeInSecForIos | It is used only for Ios ( 1 sec or more ) |
backgroundColor | It specifies the background color. |
textColor | It specifies text color. |
fontSize | It specifies the font size of the notification message. |
FlutterToast.cancel(): This function is used when you want to cancel all the requests to show message to the user.
Let us see how we can show toast notification in the Flutter app through the following steps:
Step 1: Create a Flutter project in the IDE. Here, I am going to use Android Studio.
Step 2: Open the project in Android Studio and navigate to the lib folder. In this folder, open the pubspec.yaml file. Here, we need to add the flutter toast library in the dependency section and then click on get package link to import the library in your main.dart file.
pubspec.yaml
dependencies: flutter: sdk: flutter cupertino_icons: ^0.1.2 fluttertoast: ^3.1.0
It ensures that while adding the dependencies, you have left two spaces from the left side of a fluttertoast dependency. The fluttertoast dependency provides the capability to show toast notification in a simple way. It can also customize the look of the toast popup very easily.
Step 3: Open the main.dart file and create a toast notification in the widget as the code given below.
Fluttertoast.showToast( msg: 'This is toast notification', toastLength: Toast.LENGTH_SHORT, gravity: ToastGravity.BOTTOM, timeInSecForIos: 1, backgroundColor: Colors.red, textColor: Colors.yellow );
Let us see the complete code of the above steps. Open the main.dart file and replace the following code. This code contains a button, and when we pressed on this, it will display the toast message by calling FlutterToast.showToast.
import 'package:flutter/material.dart'; import 'package:fluttertoast/fluttertoast.dart'; class ToastExample extends StatefulWidget { @override _ToastExampleState createState() { return _ToastExampleState(); } } class _ToastExampleState extends State { void showToast() { Fluttertoast.showToast( msg: 'This is toast notification', toastLength: Toast.LENGTH_SHORT, gravity: ToastGravity.BOTTOM, timeInSecForIos: 1, backgroundColor: Colors.red, textColor: Colors.yellow ); } @override Widget build(BuildContext context) { return MaterialApp( title: 'Toast Notification Example', home: Scaffold( appBar: AppBar( title: Text('Toast Notification Example'), ), body: Padding( padding: EdgeInsets.all(15.0), child: Center( child: RaisedButton( child: Text('click to show'), onPressed: showToast, ), ), ) ), ); } } void main() => runApp(ToastExample());
Output
Now, run the app in Android Studio. It will give the following screen.
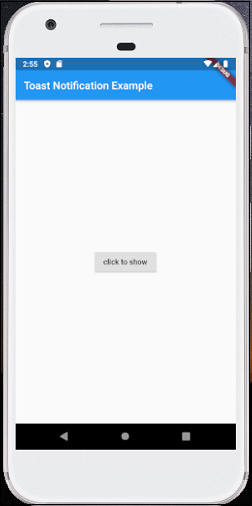
When we click on the "click to show" button, we can see the toast message at the bottom of the screen. See the below image:
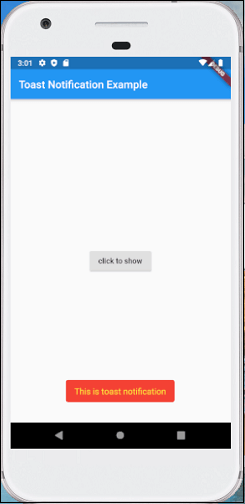