Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
Practical Paper
Industrial Training
Dart Programming - Packages
A package is a mechanism to encapsulate a group of programming units. Applications might at times need integration of some third-party libraries or plugins. Every language has a mechanism for managing external packages like Maven or Gradle for Java, Nuget for .NET, npm for Node.js, etc. The package manager for Dart is pub .
Pub helps to install packages in the repository. The repository of packages hosted can be found at https://pub.dartlang.org/.
The package metadata is defined in a file, pubsec.yaml . YAML is the acronym for Yet Another Markup Language . The pub tool can be used to download all various libraries that an application requires.
Every Dart application has a pubspec.yaml file which contains the application dependencies to other libraries and metadata of applications like application name, author, version, and description.
The contents of a pubspec.yaml file should look something like this −
name: 'vector_victor' version: 0.0.1 description: An absolute bare-bones web app. ... dependencies: browser: '>=0.10.0 <0.11.0'
The important pub commands are as follows −
Sr.No | Command & Description |
1 | ‘pub get’ |
2 | ‘pub upgrade’ |
3 | ‘pub build’ |
4 | ‘pub help’ |
If you are using an IDE like WebStorm, then you can right-click on the pubspec.yaml to get all the commands directly −
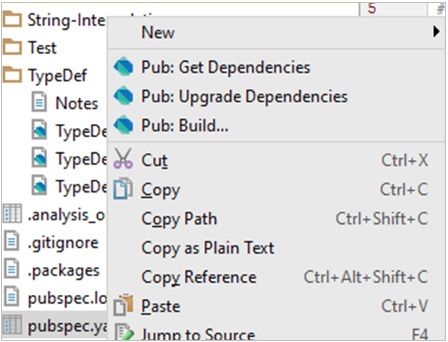
Installing a Package
Consider an example where an application needs to parse xml. Dart XML is a lightweight library that is open source and stable for parsing, traversing, querying and building XML documents.
The steps for achieving the said task is as follows −
Step 1 − Add the following to the pubsec.yaml file.
name: TestApp version: 0.0.1 description: A simple console application. #dependencies: # foo_bar: '>=1.0.0 < 2.0.0' dependencies: https://mail.google.com/mail/u/0/images/cleardot.gif xml:
Right-click on the pubsec.yaml and get dependencies. This will internally fire the pub get command as shown below.
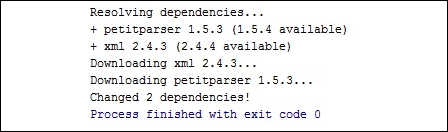
The downloaded packages and its dependent packages can be verified under the packages folder.
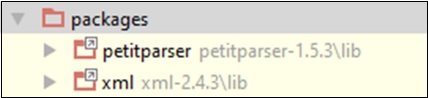
Since installation is completed now, we need to refer the dart xml in the project. The syntax is as follows −
import 'package:xml/xml.dart' as xml;
Read XML String
To read XML string and verify the input, Dart XML uses a parse() method. The syntax is as follows −
xml.parse(String input):
Example : Parsing XML String Input
The following example shows how to parse XML string input −
import 'package:xml/xml.dart' as xml; void main(){ print("xml"); var bookshelfXml = '''< ?xml version = "1.0"?> < bookshelf> < book> < title lang = "english">Growing a Language< /title> < price>29.99 < /book> < book> < title lang = "english">Learning XML< /title> < price>39.95< /price> < /book> < price>132.00< /price> < /bookshelf>'''; var document = xml.parse(bookshelfXml); print(document.toString()); }
It should produce the following output −
xml < ?xml version = "1.0"?>< bookshelf> < book> < title lang = "english">Growing a Language< /title> < price>29.99< /price> < /book> < book> < title lang = "english">Learning XML< /title> < price>39.95< /price> < /book> < price>132.00< /price> < /bookshelf>