Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- VueJS
- Go
Practical Paper
Industrial Training
'If' statement
The 'if' statement determines whether the condition is true or not. If condition is true then the 'if' block is executed otherwise, control skips the 'if' block.
Different ways to represent 'if' block:
-
if block
if-else block
if else-if ladder
nested if
Syntax of 'if':
if condition { //block statements; }
In the above syntax, if the condition is true then the block statements are executed otherwise it skips the block.
Flow Chart of "if statement"
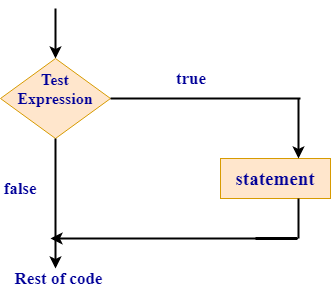
For Example:
Let's see a simple example of 'if' statement.
fn main() let a=1; if a==1 { println!("a is equal to 1"); }
Output:
a is equal to 1
In this example, value of a is equal to 1. Therefore, condition given in 'if' is true and the string passed as a parameter to the println! is displayed on the console.
"if-else"
If the condition is true then 'if' block is executed and the statements inside the 'else' block are skipped. If the condition is false then 'else' block is executed and the statements inside the 'if' block are skipped.
Syntax of "if-else"
if condition { //block statements } else { //block statements }
Flow Chart of "if-else"
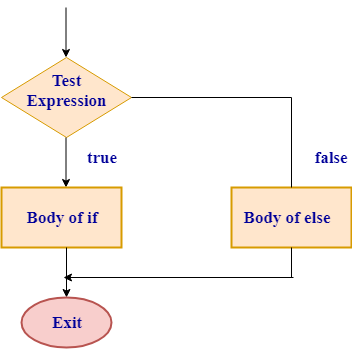
Let's see a simple example of 'if-else' statement.
fn main() { let a=3; let b=4; if a>b { println!("a is greater than b"); } else { println!("a is smaller than b"); } }
Output:
a is smaller than b
In this example, value of a is equal to 3 and value of a is less than the value of b. Therefore, else block is executed and prints "a is smaller than b" on the screen.
else-if
When you want to check the multiple conditions, then 'else-if' statement is used.
Syntax of else-if
if condition 1 { //block statements } else if condition 2 { //block statements } . . else{ //block statements }
In the above syntax, Rust executes the block for the first true condition and once it finds the first true condition then it will not execute the rest of the blocks.
Flow Chart of "else if"
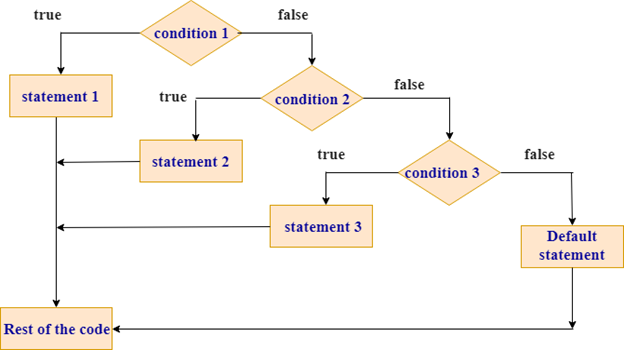
Let's see a simple example of else-if statement
fn main() let num= -5; if num>0 { println!("number is greater than 0"); } else if num< 0 { println!("number is less than 0 "); } else { println!("number is not equal to 0"); }
Output:
number is less than 0
In this example, value of num is equal to -5 and num is less than 0. Therefore, else if block is executed.
Nested if-else
When an if-else statement is present inside the body of another if or else block then it is known as nested if-else.
Syntax of Nested if-else
if condition 1 { // block statements if condition 2 { //block statements } else { //block statements } } else { //block statements }
Let's see a simple example of nested if-else
fn main() let a=5; let b=6; if a!=b { if a>b { println!("a is greater than b"); } else { println!("a is less than b"); } } else { println!("a is equal to b"); }
Output:
a is less than b
In this example, value of a is not equal to b. So, control goes inside the 'if' block and the value of a is less than b. Therefore, 'else' block is executed which is present inside the 'if' block.