Theoretical Paper
- Computer Organization
- Data Structure
- Digital Electronics
- Object Oriented Programming
- Discrete Mathematics
- Graph Theory
- Operating Systems
- Software Engineering
- Computer Graphics
- Database Management System
- Operation Research
- Computer Networking
- Image Processing
- Internet Technologies
- Micro Processor
- E-Commerce & ERP
- Dart Programming
- Flutter Tutorial
- Numerical Methods Tutorials
- VueJS
- Go
Practical Paper
Industrial Training
What is a slice?
Slice is a data type that does not have ownership. Slice references a contiguous memory allocation rather than the whole collection. It allows safe, efficient access to an array without copying. Slice is not created directly but from an existing variable. A slice consists of the length, and it can be mutable or not. Slices behave like arrays only.
String slice
A string slice refers to a part of the string. Slice looks like:
let str=String::from("javaTpoint tutorial"); let javaTpoint=&str[0..10]; let tutorial=&str[11,18];
Rather than taking an entire string, we want to take a part of the string. The [start..end] syntax is a range that begins at the start but does not include end. Therefore, we can create a slice by specifying the range within brackets such as [start..end] where 'start' specifies the starting position of an element and 'end' is one more than the last position in the slice. If we want to include the end of the string, then we have to use '..=' instead of '..'.
let str= String::from("javaTpoint tutorial"); let javaTpoint = &str[0..=9]; let tutorial= &str[11..=18] ;
Diagrammatically representation:
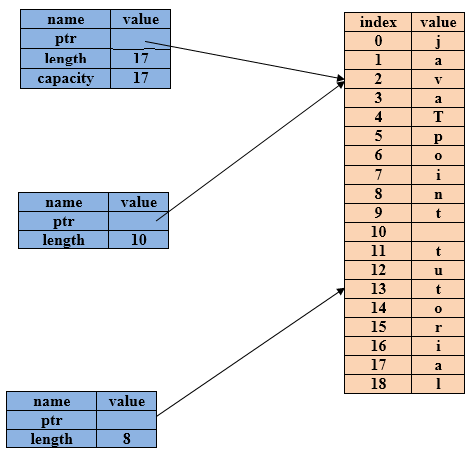
-
If you want to start the index from 0, then we can drop the starting index also. It looks like:
let str= String::from("hello world"); let hello = &str[0..5]; let hello=&str[..5];
-
If slice includes the last byte of the string, then we can drop the starting index. It looks like:
let str= String::from("hello world") ; let hello=&str[6..len]; let world = &str[6..];
Let's see a simple example of string slice:
fn main() let str=String::from("javaTpoint tutorial"); let javatpoint=&str[..=9]; println!("first word of the given string is {}",javatpoint);
Output:
first word of the given string is javaTpoint
String slices are literals
String literals are stored in binary and string literals are considered as string slices only. Let's look:
let str = "Hello javaTpoint" ;
The type of 'str' is '&str'. It is a slice pointing to a specific point of the binary. String literals are immutable, and '&str' is an immutable reference.
String slices as parameters
If we have a string slice, then we can pass it directly. Instead of passing the reference, we pass the string slice as a parameter to the function to make an API more general and useful without losing its functionality.
fn main() { let str= String:: from("Computer Science"); let first_word= first_word(&str[..]); //first_word function finds the first word of the string. let s="Computer Science" ; //string literal let first_word=first_word(&s[..]); // first_word function finds the first word of the string. let first_word=first_word(s) ; //string slice is same as string literal. Therefore, it can also be written in this way also. }
Other slices
An array can also be treated as slices. They behave similarly as a string slice. The slice has the type [&i32]. They work similarly as a string slice by storing a reference as a first element and length as a second element.
Consider a array:
let arr = [100,200,300,400,500]; // array initialization et a = &arr[1..=3]; // retrieving second,third and fourth element
Let's see a simple example.
fn main() let arr = [100,200,300,400,500,600]; let mut i=0; let a=&arr[1..=3]; let len=a.len(); println!("Elements of 'a' array:"); while i< len { println!("{}",a[i]); i=i+1; } }
Output:
Elements of 'a' array: 200 300 400